How to Convert Floating-Point Number to an Integer in Python
-
Convert Floating-Point Number to Integer Using
int()
Function in Python -
Convert Floating-Point Number to Integer Using
math
Module in Python
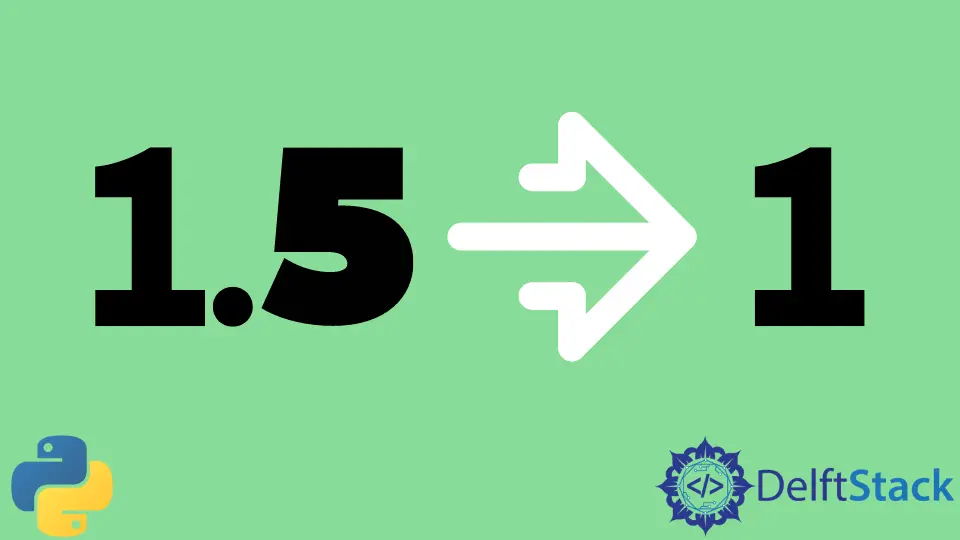
Converting a floating number to an integer in Python is relatively easy thanks to the in-built functions and libraries. When converting a floating number to an integer, we have two possibilities. Though it is easy to write a function ourselves to do this task, we will only talk about how to use the in-built functions and libraries in this article.
Suppose we have a number, say 1.52
. If we wish to convert this number to an integer, we can either go with 2
or 1
. The former is the ceiling value, and the latter is the floor value. Since there are several functions to do this task, they all carry out the above task differently and return different values. So choose the function accordingly based on your use case.
Convert Floating-Point Number to Integer Using int()
Function in Python
number = 25.49
print(int(number))
Output:
25
The int()
function accepts an argument representing a number and converts it to an integer. This argument can be a string, a float value, or an integer itself. The function considers the integer value or part before the decimal in the number and returns it.
But int()
acts a bit differently when a number of the form integer.9999999999999999
is passed as an argument. When there are more than or equal to sixteen 9
digits after the decimal, the function returns integer + 1
in case of a positive value and integer - 1
in case of a negative value as an answer.
Refer to the following code snippet for a better understanding of the concept.
print(int(1.5))
print(int(1.51))
print(int(1.49))
print(int(-1.5))
print(int(-1.51))
print(int(-1.49))
print(int(1.9999999999999999))
print(int(-1.9999999999999999))
Output:
0
1
1
1
1
-1
-1
-1
2
-2
Convert Floating-Point Number to Integer Using math
Module in Python
We can achieve the same task by using an in-built Python library, math
. This library has all sorts of mathematical functions required for mathematical calculations.
We’ll talk about only three mathematical functions from the math
library.
As the name suggests, the trunc()
function truncates or cuts or removes the decimal part of the number passed as an argument and only considers the integer part. It behaves exactly the same as the in-built int()
function and behaves differently for the exception we talked about above.
import math
print(math.trunc(0))
print(math.trunc(1))
print(math.trunc(1.5))
print(math.trunc(1.51))
print(math.trunc(1.49))
print(math.trunc(-1.5))
print(math.trunc(-1.51))
print(math.trunc(-1.49))
print(math.trunc(1.9999999999999999))
print(math.trunc(-1.9999999999999999))
Output:
0
1
1
1
1
-1
-1
-1
2
-2
Refer to the official documents to learn more about the function, here
Next, we have the ceil()
function. This function returns the ceiling value of the number or the smallest integer greater than or equal to the number passed as an argument.
import math
print(math.ceil(0))
print(math.ceil(1))
print(math.ceil(1.5))
print(math.ceil(1.51))
print(math.ceil(1.49))
print(math.ceil(-1.5))
print(math.ceil(-1.51))
print(math.ceil(-1.49))
print(math.ceil(1.9999999999999999))
print(math.ceil(-1.9999999999999999))
Output:
0
1
2
2
2
-1
-1
-1
2
-2
Refer to the official documents to learn more about the function, here
Lastly, we have the floor()
function. This function returns the floor value of the number or the largest integer that is less than or equal to the number passed as an argument.
import math
print(math.floor(0))
print(math.floor(1))
print(math.floor(1.5))
print(math.floor(1.51))
print(math.floor(1.49))
print(math.floor(-1.5))
print(math.floor(-1.51))
print(math.floor(-1.49))
print(math.floor(1.9999999999999999))
print(math.floor(-1.9999999999999999))
Output:
0
1
1
1
1
-2
-2
-2
2
-2
Refer to the official documents to learn more about the function, here
Related Article - Python Float
- How to Find Maximum Float Value in Python
- How to Fix Float Object Is Not Callable in Python
- How to Convert a String to a Float Value in Python
- How to Check if a String Is a Number in Python
- How to Convert String to Decimal in Python
- How to Convert List to Float in Python