How to Create Array of Objects in PHP
- Create an Array of Class’ Objects in PHP
-
Create an Array of
stdClass
Objects in PHP -
Create an Array of Object Using the
array()
Function in PHP
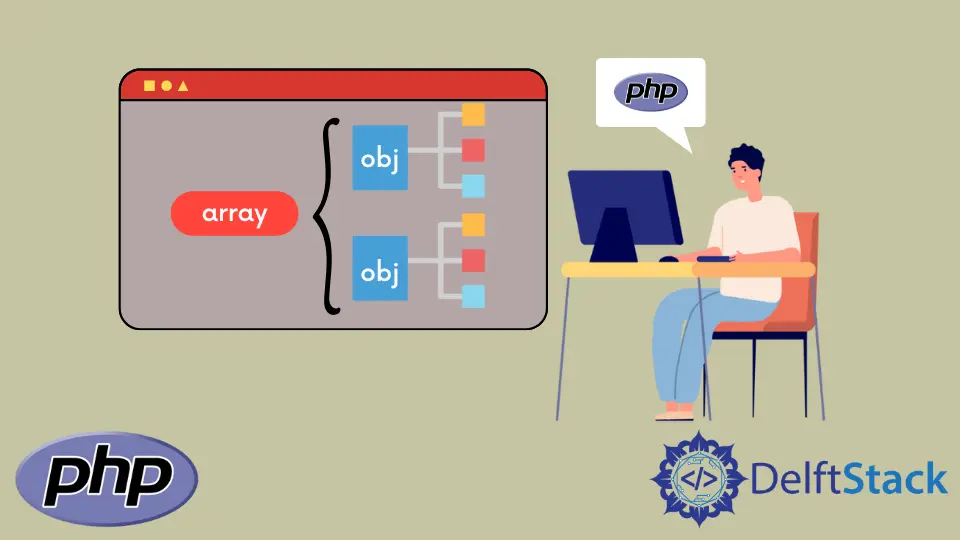
This article will introduce methods to create an array of objects in PHP.
Create an Array of Class’ Objects in PHP
We can use the array()
function to create an array of objects in PHP. The function will take the object as the arguments and will create an array of those objects. We can create objects by creating a class and defining some properties of the class. The properties of the class will have some values. Finally, the properties and values will form a key-value pair in the array.
For example, create a class Motorcycle
. Create two public properties, $name
and $type
. Then create an object $bike1
of the Motorcycle
class using the new
keyword. Populate the properties of the object with any suitable values. Similarly, create another object, $bike2
and populate the values accordingly. Next, create a variable $bike
and write the array()
function to it with the two objects $bike1
and $bike2
as the parameters. Finally, print the array variable $bikes
with the print_r()
function.
Thus, we can create an array of objects, as shown in the output section. We have created an array of the Motorcycle
objects in the example below. We can see the indexes 0
and 1
for each Motorcycle
object. The properties and values of each object are formed as a key-value pair, as stated above.
Example Code:
<?php
class Motorcycle
{
public $name;
public $type;
}
$bike1 = new Motorcycle();
$bike1->name = 'Husqvarna';
$bike1->type = 'dirt';
$bike2 = new Motorcycle();
$bike2->name = 'Goldwing';
$bike2->type = 'touring';
$bikes = array($bike1, $bike2);
?>
<pre><?php print_r($bikes);?> </pre>
Output:
Array
(
[0] => Motorcycle Object
(
[name] => Husqvarna
[type] => dirt
)
[1] => Motorcycle Object
(
[name] => Goldwing
[type] => touring
)
)
Create an Array of stdClass
Objects in PHP
We can create an array of objects by creating an object of the stdClass
in PHP. The stdClass
is defined in the standard set of functions in PHP. It is not a base class of objects; rather, it is an empty class that can be used to typecast and set dynamic properties. We can create an object of the stdClass
, which is an array by nature. Then, we can assign the dynamic properties to the object with the indexes.
For example, create an array $bikes[]
and make it an object of the stdClass
using the new
keyword. Then, give the index 0
to the $bikes[]
array and assign the properties name
and type
. Give some suitable values of your choice to the properties. Repeat the same process for index 1
in the $bikes[]
array. Next, print the $bikes
array.
The example below creates an array of stdClass
objects, as shown in the output section below.
Example Code:
<?php
$bikes[] = new stdClass;
$bikes[0]->name = 'Husqvarna';
$bikes[0]->type = 'dirt';
$bikes[1]->name = 'Goldwing';
$bikes[1]->type = 'touring';
?>
<pre><?php print_r($bikes);?> </pre>
Ouput:
Array
(
[0] => stdClass Object
(
[name] => Husqvarna
[type] => dirt
)
[1] => stdClass Object
(
[name] => Goldwing
[type] => touring
)
)
Create an Array of Object Using the array()
Function in PHP
This method is quite similar to the first method. We can create an array of objects by creating objects from a class. Here, we will first create an array using the array()
function and then populate the objects in the array. In the first method, we created objects and then populated them in the array using the array()
function. We will use the array index to set the values to the properties.
For example, create a Motorcycle
class with properties as in the first method. Then create an array with the $bikes
variable using the array()
function. Leave the array empty. Then, create an object of the class from the $bike
array using the 0
index. Set the properties and values for the 0
index as well. Repeat the same process for the 1
index. Finally, print the array using the print_r()
function.
Example Code:
<?php
class Motorcycle
{
public $name;
public $type;
}
$bikes = array();
$bikes[0] = new Motorcycle();
$bikes[0]->name = 'Husqvarna';
$bikes[0]->type = 'dirt';
$bikes[1] = new Motorcycle();
$bikes[1]->name = 'Goldwing';
$bikes[1]->type = 'touring';
?>
<pre><?php print_r($bikes);?> </pre>
Output:
Array
(
[0] => Motorcycle Object
(
[name] => Husqvarna
[type] => dirt
)
[1] => Motorcycle Object
(
[name] => Goldwing
[type] => touring
)
)
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedInRelated Article - PHP Array
- How to Determine the First and Last Iteration in a Foreach Loop in PHP
- How to Convert an Array to a String in PHP
- How to Get the First Element of an Array in PHP
- How to Echo or Print an Array in PHP
- How to Delete an Element From an Array in PHP
- How to Remove Empty Array Elements in PHP