How to Create an Object Without Class in PHP
-
Use
new stdClass()
to Create an Object Without a Class in PHP -
Typecast an Array Into an Object Using the
object
Data Type to Create an Object Without Creating a Class -
Use the
json_dencode()
Function to Create an Object Without Creating a Class in PHP
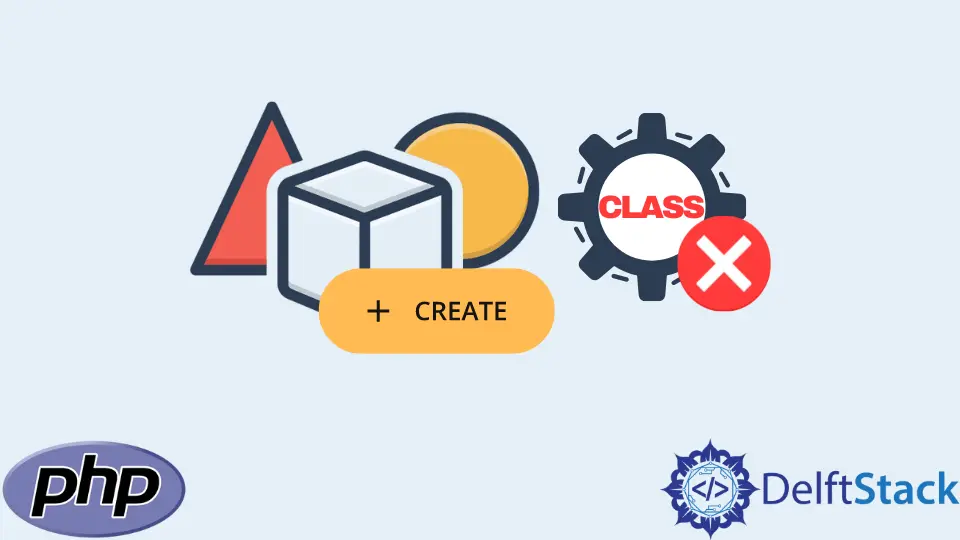
This article will introduce methods to create an object without creating a class in PHP.
Use new stdClass()
to Create an Object Without a Class in PHP
We can create an object from the stdClass()
without making a base class in PHP. We can use the new
operator to create an object of the stdClass()
. The object can access the properties directly by calling them. Thus, we can create dynamic objects and properties using the stdClass()
. The compiler creates an instance of the stdClass()
when an array is typecasted to an object.
For example, create a variable $object
and store an instance of the stdClass()
created by the new
operator. Call a variable property
from the $object
object and assign a string. Assign the string, I am an object's property
. Use the backslash \
to escape the apostrophe in the text. Apply the var_dump()
function on the $object
variable to dump the information about the variable.
In the example below, we created an object of the stdClass()
, and we instantly assigned a value to the object’s property. When we dump the object, we will see the object of the stdClass
class created in the output. Thus, we can create an object without creating a base class in PHP.
Example Code:
#php 7.x
<?php
$object = new stdClass();
$object->property = 'I am an object\'s property';
var_dump($object);
?>
Output:
object(stdClass)#1 (1) { ["property"]=> string(25) "I am an object's property" }
Typecast an Array Into an Object Using the object
Data Type to Create an Object Without Creating a Class
We can create an object without creating a class in PHP, typecasting a type into an object using the object
data type. We can typecast an array into a stdClass
object. The object
keyword is wrapped around with parenthesis right before the array typecasts the array into the object. We can use the var_dump()
function as the first method to see the information about the typecasted object.
For example, create a variable $place
and store an array in it. The array contains keys as city
and country
and the respective values as Pokhara
and Nepal
. Assign the $place
variable to a new $obj
variable. Write the object
data type wrapped inside a parenthesis just before the $place
variable. Call the var_dump()
function with the $obj
as parameter.
The example below typecasts an array into an object. The var_dump()
function shows the $obj
object in the output section. It shows that it is a stdClass
object. Check the PHP Manual to know how typecasting works.
Code Example:
#php 7.x
<?php
$place = ['city' => 'Pokhara', 'country' =>'Nepal'];
$obj = (object) $place;
var_dump($obj);
?>
Output:
object(stdClass)#1 (2) { ["city"]=> string(7) "Pokhara" ["country"]=> string(5) "Nepal" }
Use the json_dencode()
Function to Create an Object Without Creating a Class in PHP
The json_decode()
function converts the JSON string into a PHP object. The function takes a string parameter which will be converted into an object. The function also takes an optional boolean parameter. The default value of this boolean parameter is false
, which will convert the JSON object into a PHP object. If the value is true
, it will convert the JSON object into an associative array.
For example, create a variable $jsonobj
and store a JSON object into it. The object contains Harry
, Tony
and Juan
as the key and the integer values 27
, 24
and 32
as the respective values. The key-value pairs are enclosed within the curly braces. The JSON object is wrapped around with quotes as a string. Then use the json_decode()
function on the $jsonobj
variables. Apply the var_dump()
function to the json_encode()
function to display the information about the encoded object.
In the example below, json_encode()
function converts the JSON object to PHP object of stdClass
. The var_dump()
function shows the property and the value of the object. Thus, we created an object without creating a class in PHP. It also displays the type of the value. Please check the PHP Manual to understand more about the json_decode()
function.
Example Code:
# php 7.x
<?php
$jsonobj = '{"Harry":27,"Tony":24,"Juan":32}';
var_dump(json_decode($jsonobj));
?>
Output:
object(stdClass)#1 (3) { ["Harry"]=> int(27) ["Tony"]=> int(24) ["Juan"]=> int(32) }
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn