How to Create Default Object From Empty Value in PHP
-
Create a
stdClass()
Object in PHP - Typecast Array Into Object in PHP
- Create Object From Anonymous Class in PHP
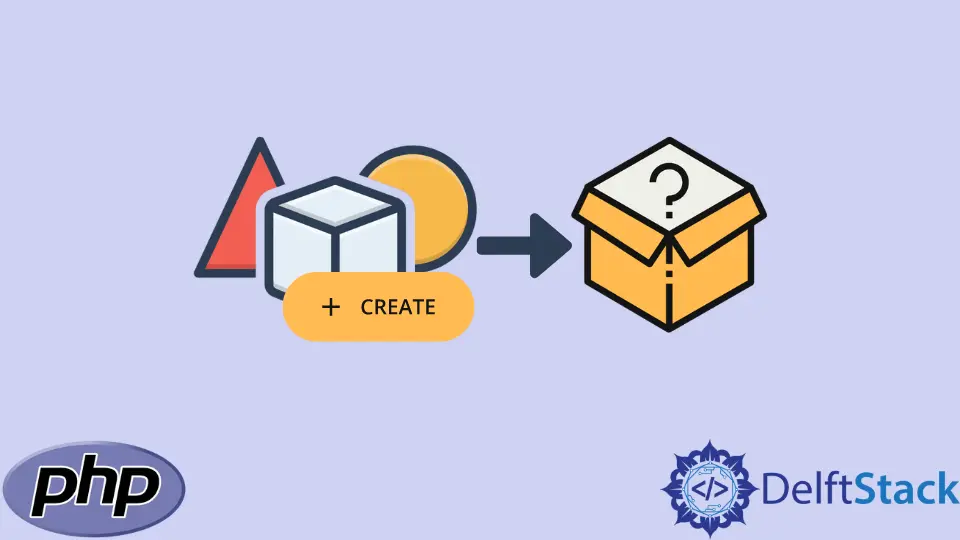
We will introduce some methods for creating objects in PHP and solving the error creating default object from empty value
.
Create a stdClass()
Object in PHP
When we try to assign properties of an object without initializing an object, an error will be thrown. The error will say creating default object from empty value
. The error also depends upon the configuration in the php.ini
file. When the error is suppressed in the configuration, then such script will not throw the error. It is not better to change the configuration file to suppress the error to get rid of it. Instead, we can create a stdClass()
object to get rid of the error. When we declare a variable as an object of the stdClass()
in the global namespace, we can dynamically assign properties to the objects.
For example, create a variable $obj
and set it to NULL
. Then, set the success
property to false
with the $obj
object. In this instance, an error will be thrown, as shown in the output section. It is because $obj
has not been initialized as an object.
Example Code:
$obj = NULL;
$obj->success = true;
Output:
Warning: Creating default object from empty value
To eliminate the error, first, assign the $obj
variable with the instance of stdClass()
. Next, set the success
property to true
with the $obj
. Then, print the $obj
object using the print_r()
function. It is even better to use the isset()
function to check if $obj
exists already. We can see the information about $obj
in the output section. Thus, we can eliminate the error by creating an object of stdClass()
.
Example Code:
$obj = new stdClass();
$obj->success =true;
print_r($obj);
Output:
stdClass Object ( [success] => 1 )
Typecast Array Into Object in PHP
We can typecast an array to an object using the object
keyword before the array. In this way, the object can be created. Then, we can assign the properties to the object. Since we already initialized the object, no error will be thrown while assigning properties of the object. This method also creates an object of the stdClass()
class.
For example, create a variable $obj
and assign it to the array()
function. Then, write the object
keyword in parenthesis before array()
. The array has been converted into an object. Then, assign the true
value to the success
property with the $obj
. Finally, print the object with the print_r()
function. In this way, we can create an object typecasting an array and get rid of the error.
Example Code:
$obj = (object)array();
$obj->success =true;
print_r($obj);
Output:
stdClass Object ( [success] => 1 )
Create Object From Anonymous Class in PHP
We can create an object from an anonymous class in PHP and assign properties to it. We can use the new class
keyword to create an anonymous class. We can set the value of the properties as in the generic class. Since the property will have a class, and we can access it with an object, no error will be thrown.
For example, create an object $obj
assign an anonymous class using the new class
keyword to the object. Then create a public
property $success
and set the value to true
. Outside, the class, print the object with the print_r()
function. In this way, we can create an object from an anonymous class in PHP and prevent the error.
Example Code:
$obj = new class {
public $success = true;
};
print_r($obj);
Output:
class@anonymous Object ( [success] => 1 )
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn