How to Change the Line Width of Lines in Matplotlib Legend
-
set_linewidth()
Method to Set the Line Width in Matplotliblegend
-
matplotlib.pyplot.setp()
Method to Set the Line Width in Matplotliblegend
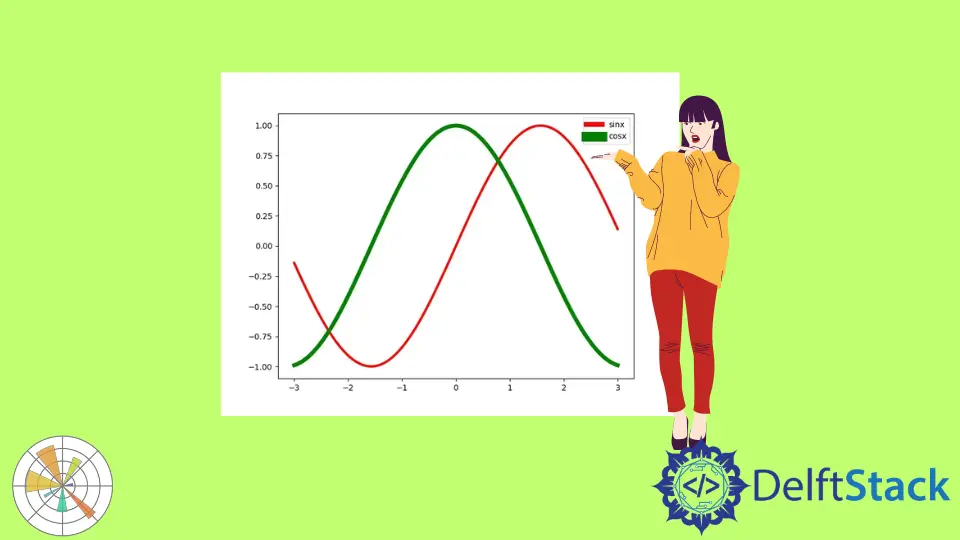
We can change the line width (line thickness) of lines in Python Matplotlib legend by using the set_linewidth()
method of the legend object and the setp()
method of the artist
objects.
set_linewidth()
Method to Set the Line Width in Matplotlib legend
The linewidth
parameter in the plot
function can be used to control the width of the plot of a particular object, and the set_linewidth()
method can be used to control the width of the lines of the legend in Matplotlib.
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(-3, 3, 100)
y1 = np.sin(x)
y2 = np.cos(x)
fig, ax = plt.subplots(figsize=(8, 6))
ax.plot(x, y1, c="r", label="sinx", linewidth=3.0)
ax.plot(x, y2, c="g", label="cosx", linewidth=5.0)
leg = plt.legend()
leg.get_lines()[0].set_linewidth(6)
leg.get_lines()[1].set_linewidth(10)
plt.show()
Output:
The line widths of two lines in the Python Matplotlib plot are 3.0
and 5.0
respectively, and the line width of lines in the legend by default uses the same line width as in the plot.
leg = plt.legend()
leg.get_lines()[0].set_linewidth(6)
leg
is the Python Matplotlib legend
object, and leg.get_lines()
returns the list of line instances in the legend.
set_linewidth()
could change the line width (line thickness) of the legend line to be another value rather than that in the plot.
matplotlib.pyplot.setp()
Method to Set the Line Width in Matplotlib legend
matplotlib.pyplot.setp()
method allows us to set the property of pyplot objects. We can use the linewidth
parameter of setp()
function to set linewidth of particular legend object.
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(-3, 3, 100)
y1 = np.sin(x)
y2 = np.cos(x)
fig, ax = plt.subplots(figsize=(8, 6))
ax.plot(x, y1, c="r", label="sinx", linewidth=3.0)
ax.plot(x, y2, c="g", label="cosx", linewidth=5.0)
leg = plt.legend()
leg_lines = leg.get_lines()
leg_texts = leg.get_texts()
plt.setp(leg_lines[0], linewidth=6)
plt.setp(leg_lines[1], linewidth=12)
plt.setp(leg_texts, fontsize=10)
plt.show()
Output:
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn