How to Set Legend Title in Matplotlib
-
Use the
title
Parameter to Add a Title to the Legend in Matplotlib Figures -
Use the
set_title()
Function to Add a Title to the Legend in Matplotlib Figures
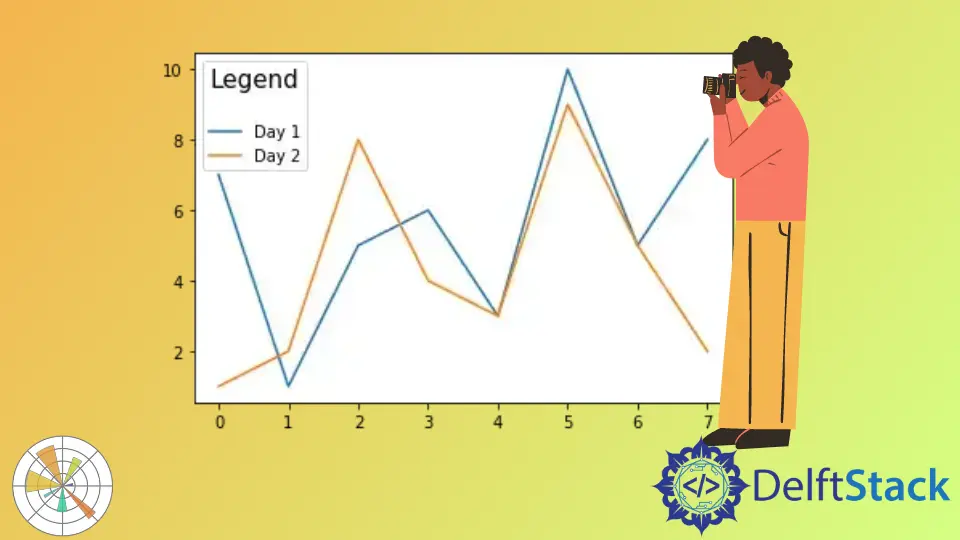
Legend is a small box that tells about the data plotted on the graph. It is used to explain the graph by telling which element or color represents what data. Generally, it is placed at some corner of the plot.
In matplotlib figures, we can add a legend using the matplotlib.pyplot.legend()
function.
In this tutorial, we will discuss how to add a title to the legend of a matplotlib figure in Python.
Use the title
Parameter to Add a Title to the Legend in Matplotlib Figures
We can easily use the title
parameter in the legend()
function to achieve this.
For example,
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
df = pd.DataFrame(
{"Day 1": [7, 1, 5, 6, 3, 10, 5, 8], "Day 2": [1, 2, 8, 4, 3, 9, 5, 2]}
)
plt.plot(df)
legend = plt.legend(["Day 1", "Day 2"], title="Legend")
The above method also works with an axes object when dealing with subplots.
The size of the title can be altered using the title_fontsize
parameter within the legend()
function. Other customizations can also be made. We can use the _legend_box.sep
method to control the distance between the legend’s content and the title. The alignment of the title can be changed using the _legend_box.align
.
We use some of the methods mentioned above in the following example.
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
df = pd.DataFrame(
{"Day 1": [7, 1, 5, 6, 3, 10, 5, 8], "Day 2": [1, 2, 8, 4, 3, 9, 5, 2]}
)
plt.plot(df)
legend = plt.legend(["Day 1", "Day 2"], title="Legend", title_fontsize=15)
legend._legend_box.sep = 20
Use the set_title()
Function to Add a Title to the Legend in Matplotlib Figures
This function is normaly used to add a legend to the axes. We can also use it to add a title to the legend. The properties of the title can be specified using the prop
argument.
For example,
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
df = pd.DataFrame(
{"Day 1": [7, 1, 5, 6, 3, 10, 5, 8], "Day 2": [1, 2, 8, 4, 3, 9, 5, 2]}
)
plt.plot(df)
legend = plt.legend(["Day 1", "Day 2"])
legend.set_title("Legend", prop={"size": 15})
In the above example, we increased the size of the title. Other customizations or tweaks can also be specified in the dictionary, which is passed to the prop
argument. The methods discussed in the previous example, the _legend_box.align
and _legend_box.sep
can also be used here.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn