How to Add Title to Subplots in Matplotlib
-
Set_title()
Method to Add Title to Subplot in Matplotlib -
title.set_text()
Method to Set Title of Subplots in Matplotlib -
plt.gca().set_title()
/plt.gca.title.set_text()
to Set Title to Subplots in Matplotlib
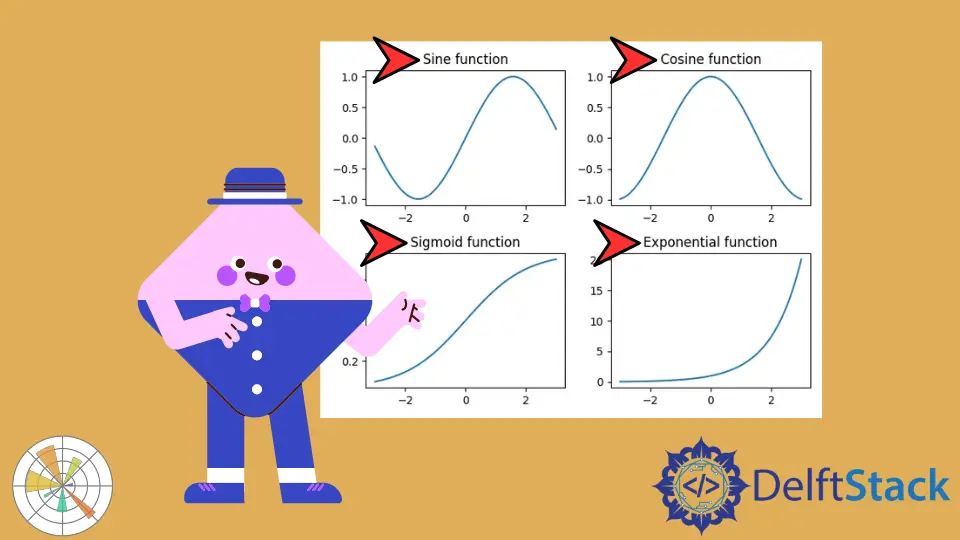
We use set_title(label)
and title.set_text(label)
methods to add titles to subplots in Matplotlib.
Set_title()
Method to Add Title to Subplot in Matplotlib
We use matplotlib.axes._axes.Axes.set_title(label)
method to set title (string label
) for the current subplot Axes
.
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(-3, 3, 100)
y1 = np.sin(x)
y2 = np.cos(x)
y3 = 1 / (1 + np.exp(-x))
y4 = np.exp(x)
fig, ax = plt.subplots(2, 2)
ax[0, 0].plot(x, y1)
ax[0, 1].plot(x, y2)
ax[1, 0].plot(x, y3)
ax[1, 1].plot(x, y4)
ax[0, 0].set_title("Sine function")
ax[0, 1].set_title("Cosine function")
ax[1, 0].set_title("Sigmoid function")
ax[1, 1].set_title("Exponential function")
fig.tight_layout()
plt.show()
Output:
If we want to loop over some subplots and show them one at a time along with titles, we can use the following shorter code:
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(-3, 3, 100)
y = [0, 0, 0, 0]
y[0] = np.sin(x)
y[1] = np.cos(x)
y[2] = 1 / (1 + np.exp(-x))
y[3] = np.exp(x)
figure, ax = plt.subplots(2, 2)
i = 0
for a in range(len(ax)):
for b in range(len(ax[a])):
ax[a, b].plot(x, y[i])
subplot_title = "Subplot" + str(i)
ax[a, b].set_title(subplot_title)
i = i + 1
figure.tight_layout()
plt.show()
Output:
title.set_text()
Method to Set Title of Subplots in Matplotlib
We can also add title to subplots in Matplotlib using title.set_text()
method, in similar way to set_title()
method.
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(-3, 3, 100)
y1 = np.sin(x)
y2 = np.cos(x)
y3 = 1 / (1 + np.exp(-x))
y4 = np.exp(x)
fig, ax = plt.subplots(2, 2)
ax[0, 0].plot(x, y1)
ax[0, 1].plot(x, y2)
ax[1, 0].plot(x, y3)
ax[1, 1].plot(x, y4)
ax[0, 0].title.set_text("Sine function")
ax[0, 1].title.set_text("Cosine function")
ax[1, 0].title.set_text("Sigmoid function")
ax[1, 1].title.set_text("Exponential function")
fig.tight_layout()
plt.show()
Output:
plt.gca().set_title()
/ plt.gca.title.set_text()
to Set Title to Subplots in Matplotlib
If you use Matlab-like style in the interactive plotting, then you could use plt.gca()
to get the reference of the current axes of the subplot and combine set_title()
or title.set_text()
method to set title to the subplots in Matplotlib.
import matplotlib.pyplot as plt
plt.subplots(2, 2)
x = [1, 2, 3]
y = [2, 4, 6]
for i in range(4):
plt.subplot(2, 2, i + 1)
plt.plot(x, y)
plt.gca().set_title("Title-" + str(i))
plt.show()
plt.tight_layout()
or,
import matplotlib.pyplot as plt
plt.subplots(2, 2)
x = [1, 2, 3]
y = [2, 4, 6]
for i in range(4):
plt.subplot(2, 2, i + 1)
plt.plot(x, y)
plt.gca().title.set_text("Title-" + str(i))
plt.show()
plt.tight_layout()
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn