如何在 Matplotlib 中向子圖新增標題
Suraj Joshi
2024年2月15日
Matplotlib
Matplotlib Subplot
- 使用 set_title()方法將標題新增到 Matplotlib 中的子圖
-
使用
title.set_text()
方法設定 Matplotlib 子圖的標題 -
plt.gca().set_title()
/plt.gca.title.set_text()
將標題設定為 Matplotlib 中的子圖
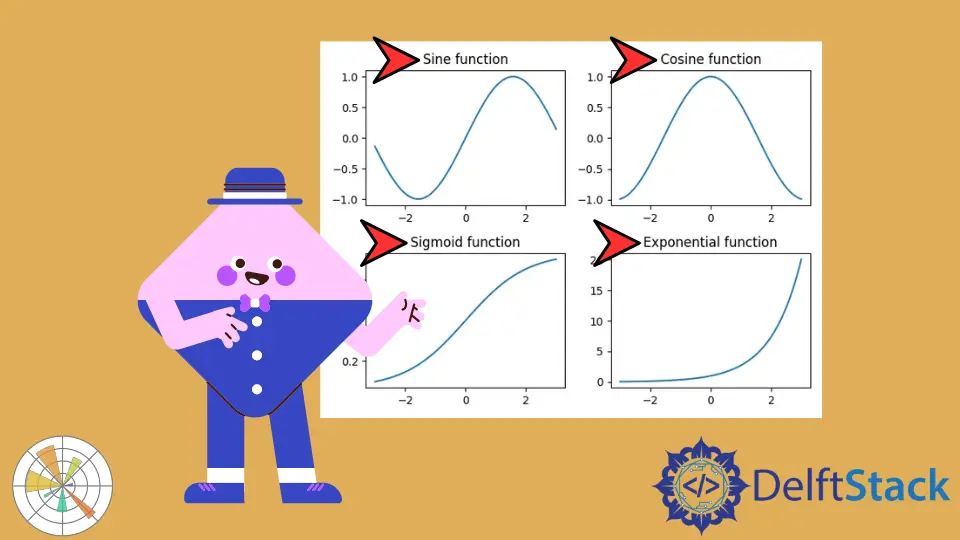
我們使用 set_title(label)
和 title.set_text(label)
方法將標題新增到 Matplotlib 中的子圖中。
使用 set_title()方法將標題新增到 Matplotlib 中的子圖
我們使用 matplotlib.axes._axes.Axes.set_title(label)
方法來設定當前子圖 Axes
的標題(字串 label
)。
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(-3, 3, 100)
y1 = np.sin(x)
y2 = np.cos(x)
y3 = 1 / (1 + np.exp(-x))
y4 = np.exp(x)
fig, ax = plt.subplots(2, 2)
ax[0, 0].plot(x, y1)
ax[0, 1].plot(x, y2)
ax[1, 0].plot(x, y3)
ax[1, 1].plot(x, y4)
ax[0, 0].set_title("Sine function")
ax[0, 1].set_title("Cosine function")
ax[1, 0].set_title("Sigmoid function")
ax[1, 1].set_title("Exponential function")
fig.tight_layout()
plt.show()
輸出:
如果我們要遍歷某些子圖並一次顯示一個子圖和標題,則可以使用以下較短的程式碼:
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(-3, 3, 100)
y = [0, 0, 0, 0]
y[0] = np.sin(x)
y[1] = np.cos(x)
y[2] = 1 / (1 + np.exp(-x))
y[3] = np.exp(x)
figure, ax = plt.subplots(2, 2)
i = 0
for a in range(len(ax)):
for b in range(len(ax[a])):
ax[a, b].plot(x, y[i])
subplot_title = "Subplot" + str(i)
ax[a, b].set_title(subplot_title)
i = i + 1
figure.tight_layout()
plt.show()
輸出:
使用 title.set_text()
方法設定 Matplotlib 子圖的標題
我們也可以使用 title.set_text()
方法將標題新增到 Matplotlib 中的子圖中,類似於 set_title()
方法。
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(-3, 3, 100)
y1 = np.sin(x)
y2 = np.cos(x)
y3 = 1 / (1 + np.exp(-x))
y4 = np.exp(x)
fig, ax = plt.subplots(2, 2)
ax[0, 0].plot(x, y1)
ax[0, 1].plot(x, y2)
ax[1, 0].plot(x, y3)
ax[1, 1].plot(x, y4)
ax[0, 0].title.set_text("Sine function")
ax[0, 1].title.set_text("Cosine function")
ax[1, 0].title.set_text("Sigmoid function")
ax[1, 1].title.set_text("Exponential function")
fig.tight_layout()
plt.show()
輸出:
plt.gca().set_title()
/ plt.gca.title.set_text()
將標題設定為 Matplotlib 中的子圖
如果在互動式繪圖中使用類似 Matlab 的樣式,則可以使用 plt.gca()
來獲得子圖的當前軸的參考,並結合使用 set_title()
或 title.set_text()
方法為 Matplotlib 中的子圖設定標題。
import matplotlib.pyplot as plt
plt.subplots(2, 2)
x = [1, 2, 3]
y = [2, 4, 6]
for i in range(4):
plt.subplot(2, 2, i + 1)
plt.plot(x, y)
plt.gca().set_title("Title-" + str(i))
plt.show()
plt.tight_layout()
要麼,
import matplotlib.pyplot as plt
plt.subplots(2, 2)
x = [1, 2, 3]
y = [2, 4, 6]
for i in range(4):
plt.subplot(2, 2, i + 1)
plt.plot(x, y)
plt.gca().title.set_text("Title-" + str(i))
plt.show()
plt.tight_layout()
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn