How to Move Elements Using jQuery
-
Use
appendTo
andprependTo
to Move Elements Using jQuery -
Use
insertBefore()
andinsertAfter()
to Move Elements Using jQuery
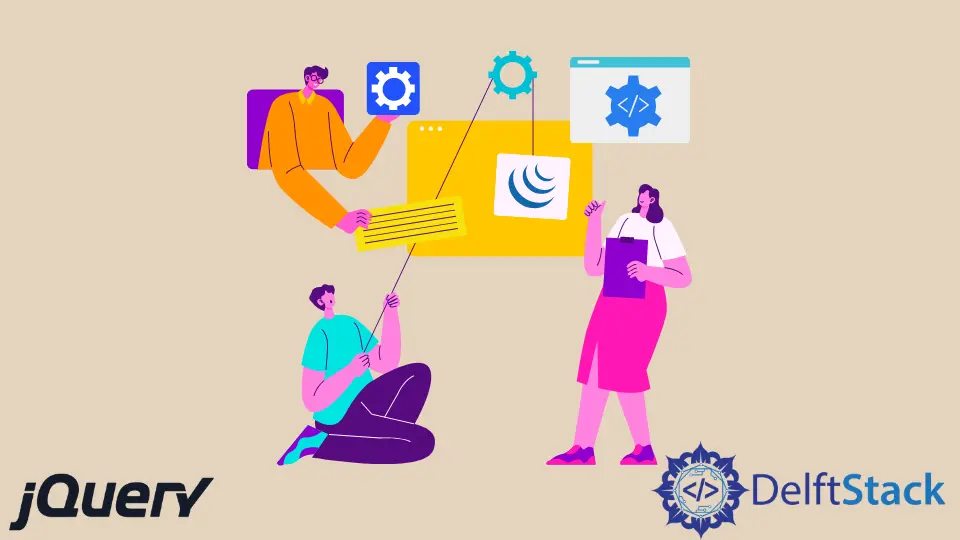
This tutorial demonstrates how to move elements in jQuery using different methods.
There are different methods in jQuery that can be used to move or insert elements at particular positions in an HTML document. These methods include appendTo()
, prependTo()
, insertBefore()
, insertAfter()
, and some other methods.
Let’s discuss the methods and show a few examples.
Use appendTo
and prependTo
to Move Elements Using jQuery
The appendTo()
and prependTo()
methods are used to move elements into another element in jQuery.
For example, suppose a div
contains three paragraphs, and we want to move one more paragraph into the div
. In that case, the appendTo()
method will move the new paragraphs into the div
after the three paragraphs, and the prependTo()
method will move the paragraph to the div
before the three paragraphs.
The syntax for both methods are shown below:
$("content").appendTo("target");
$("content").prependTo("target");
Where:
- The
content
is the content to be moved. - The
target
is the position where the element will be moved.
Let’s try an example for the appendTo()
method:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery appendTo</title>
<style>
#TargetDiv{
padding: 30px;
background: lightblue;
}
#ContentDiv{
padding: 30px;
margin: 40px 0;
border: 3px solid;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#ContentDiv").appendTo("#TargetDiv");
$(this).hide();
});
});
</script>
</head>
<body>
<div id="ContentDiv">
<h1>Hello Delftstack</h1>
<p>This is delftstack.com the best site for tutorials.</p>
<button type="button">Move Element</button>
</div>
<div id="TargetDiv">
<h1>DELFTSTACK</h1>
</div>
</body>
</html>
The code above will move the ContentDiv
to the TargetDiv
after the other elements in the div
. See output:
Now, let’s implement the same example for the prependTo()
method. See example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery prependTo</title>
<style>
#TargetDiv{
padding: 30px;
background: lightblue;
}
#ContentDiv{
padding: 30px;
margin: 40px 0;
border: 3px solid;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#ContentDiv").prependTo("#TargetDiv");
$(this).hide();
});
});
</script>
</head>
<body>
<div id="ContentDiv">
<h1>Hello Delftstack</h1>
<p>This is delftstack.com the best site for tutorials.</p>
<button type="button">Move Element</button>
</div>
<div id="TargetDiv">
<h1>DELFTSTACK</h1>
</div>
</body>
</html>
The code above will move the ContentDiv
to the TargetDiv
before the other elements in the div
. See output:
Use insertBefore()
and insertAfter()
to Move Elements Using jQuery
The insertBefore()
and insertAfter()
methods are used to move the elements before and after other elements. The syntax for these methods are shown below:
$("content").insertBefore("target");
$("content").insertAfter("target");
Where:
- The
content
is the content to be moved. - The
target
is the position after or before where the content will be moved.
Let’s try an example for the insertBefore()
method:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery insertBefore</title>
<style>
#TargetDiv{
padding: 30px;
background: lightblue;
}
#ContentDiv{
padding: 30px;
margin: 40px 0;
border: 3px solid;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#ContentDiv").insertBefore("#TargetDiv");
$(this).hide();
});
});
</script>
</head>
<body>
<div id="TargetDiv">
<h1>DELFTSTACK</h1>
</div>
<div id="ContentDiv">
<h1>Hello Delftstack</h1>
<p>This is delftstack.com the best site for tutorials.</p>
<button type="button">Move Element</button>
</div>
</body>
</html>
The code above will move the content element before the target element. See output:
Now, let’s try the example for the insertAfter()
method:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery insertAfter</title>
<style>
#TargetDiv{
padding: 30px;
background: lightblue;
}
#ContentDiv{
padding: 30px;
margin: 40px 0;
border: 3px solid;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#ContentDiv").insertAfter("#TargetDiv");
$(this).hide();
});
});
</script>
</head>
<body>
<div id="ContentDiv">
<h1>Hello Delftstack</h1>
<p>This is delftstack.com the best site for tutorials.</p>
<button type="button">Move Element</button>
</div>
<div id="TargetDiv">
<h1>DELFTSTACK</h1>
</div>
</body>
</html>
The code above will move the content after the target. See output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook