How to Create Elements in jQuery
- Create Elements in jQuery
-
Use the
append()
Method to Create Elements in jQuery -
Use the
prepend()
Method to Create Elements in jQuery -
Use the
after()
andbefore()
Methods to Create Elements in jQuery
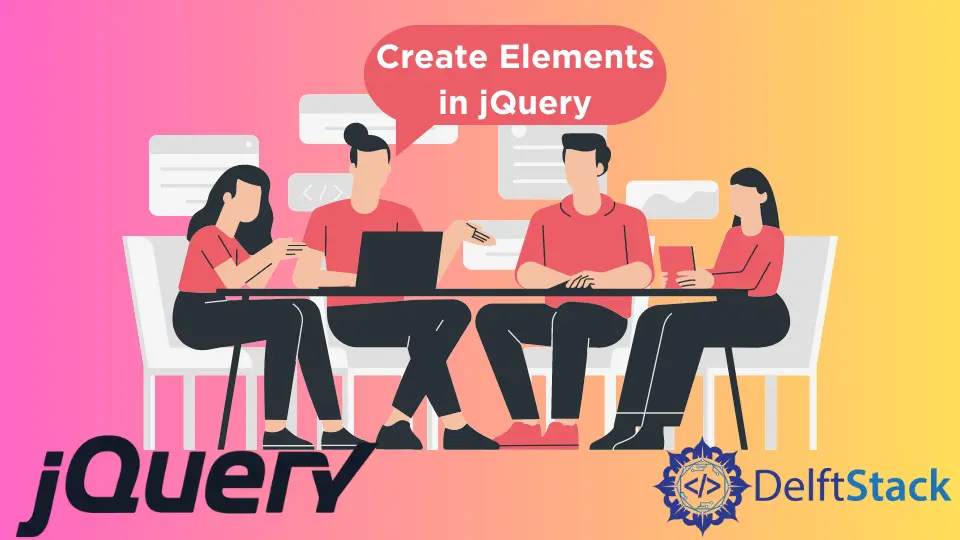
The createElement()
is a method from core JavaScript to create an HTML element. In jQuery, there are a few methods to perform a similar operation.
This tutorial demonstrates how to create an HTML element in jQuery.
Create Elements in jQuery
There are four methods in jQuery to create HTML elements:
append()
- This method will add the element at the end of the selected elements.prepend()
- This method will add the element at the beginning of the selected elements.after()
- This method will add the element after the selected elements.before()
- This method will add the element before the selected elements.
Let’s discuss and try an example for each method.
Use the append()
Method to Create Elements in jQuery
The append()
method adds the element at the end of the selected element. The syntax is simple to add one HTML element.
See example:
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("#DemoButton").click(function(){
$("#two").append(" <p> This is paragraph three </p>");
});
});
</script>
</head>
<body>
<p>This is paragraph One.</p>
<p id="two">This is paragraph two.</p>
<button id="DemoButton">Append paragraph</button>
</body>
</html>
The code above will add a single HTML paragraph element at once. See output:
We can also create multiple elements using the append()
method. See example:
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
function append_paragraphs() {
var ELement1 = "<p>This is a paragraph two. </p>"; // paragraph with HTML
var ELement2 = $("<p></p>").text("This is a paragraph three."); // paragraph with jQuery
var ELement3 = document.createElement("p");
ELement3.innerHTML = "This is a paragraph four"; // paragraph with DOM
$("body").append(ELement1, ELement2, ELement3); // Append all paragraph elements
}
</script>
</head>
<body>
<p>This is a paragraph one.</p>
<button onclick="append_paragraphs()">Append Paragraphs</button>
</body>
</html>
The code above will create three new paragraphs using the append()
method. See output:
Use the prepend()
Method to Create Elements in jQuery
The prepend()
method adds the element at the beginning of the selected element. The syntax is simple for one HTML element.
See example:
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("#DemoButton").click(function(){
$("#two").prepend(" <p> This is the new paragraph</p>");
});
});
</script>
</head>
<body>
<p>This is paragraph One.</p>
<p id="two">This is paragraph two.</p>
<button id="DemoButton">Append paragraph</button>
</body>
</html>
The code will append a paragraph before paragraph two. See output:
Similarly, we prepend multiple elements. See example:
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
function Prepend_paragraphs() {
var ELement1 = "<p>This is a paragraph one. </p>"; // paragraph with HTML
var ELement2 = $("<p></p>").text("This is a paragraph two."); // paragraph with jQuery
var ELement3 = document.createElement("p");
ELement3.innerHTML = "This is a paragraph three"; // paragraph with DOM
$("body").prepend(ELement1, ELement2, ELement3); // Prepend all paragraph elements
}
</script>
</head>
<body>
<p>This is a the last paragraph.</p>
<button onclick="Prepend_paragraphs()">Prepend Paragraphs</button>
</body>
</html>
The code above will create multiple elements before the given element. See output:
Use the after()
and before()
Methods to Create Elements in jQuery
The after()
method will create an element after the given element, and similarly, the before()
method will create an element before the given element.
See example for both methods below:
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("#button1").click(function(){
$("h1").before("<b> Element Before </b>");
});
$("#button2").click(function(){
$("h1").after("<b> Element After </b>");
});
});
</script>
</head>
<body>
<h1>Delfstack</h1>
<br><br>
<button id="button1">Insert element before</button>
<button id="button2">Insert element after</button>
</body>
</html>
The code above will create the elements before and after the given element. See output:
Similarly, we can create multiple elements with the after()
and before()
methods. See example:
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
function After_Element() {
var Element1 = "<b>This </b>"; // element with HTML
var Element2 = $("<b></b>").text("is "); //with jQuery
var Element3 = document.createElement("b"); // with DOM
Element3.innerHTML = "Delftstack.com.";
$("h1").after(Element1, Element2, Element3); // Insert new elements after h1
}
function Before_Element() {
var Element1 = "<b>This </b>"; // element with HTML
var Element2 = $("<b></b>").text("is "); //with jQuery
var Element3 = document.createElement("b"); // with DOM
Element3.innerHTML = "Delftstack.com.";
$("h1").before(Element1, Element2, Element3); // Insert new elements before h1
}
</script>
</head>
<body>
<h1>Delfstack</h1>
<br><br>
<p>Create Elements after the h1.</p>
<button onclick="After_Element()">Insert after</button>
<p>Create Elements before the h1.</p>
<button onclick="Before_Element()">Insert before</button>
</body>
</html>
The code above will add multiple elements before and after the given element. See output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook