How to Check if an Element Exists Using jQuery
- Use the Length Property to Check if an Element Exists Using jQuery
- Create a User-Defined Function to Check if an Element Exists Using jQuery
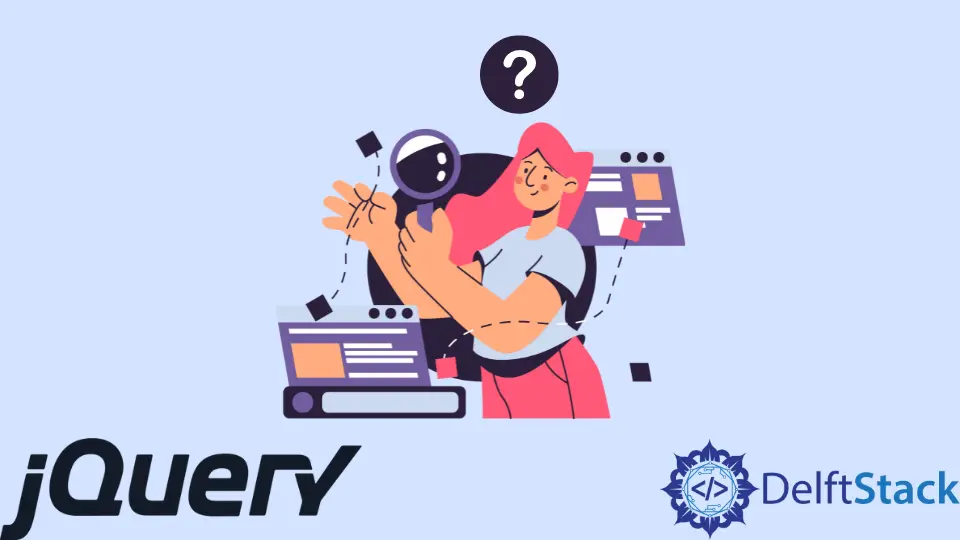
There are two methods to check if an element exists or not using jQuery, one is the length property, and the other is to use methods to create our own exist()
method.
This tutorial demonstrates how to check if an element exists or not.
Use the Length Property to Check if an Element Exists Using jQuery
The length
property of jQuery can be used to check if the element exists or not; it returns the total matched elements. If length returns 0, the element doesn’t exist, and any other value means the element exists.
The syntax for the length
property is:
($("element").length)
The above syntax will return 0 or any other number.
Let’s try an example to check if an element exists or not by using the length property. See example:
<!DOCTYPE html>
<html>
<head>
<script src= "https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"> </script>
<title>jQuery Length</title>
<style>
body {
text-align: center;
}
h1 {
color: lightblue;
font-size: 4.5rem;
}
button {
cursor: pointer;
margin-top: 4rem;
}
</style>
</head>
<body>
<h1>Delftstack | The Best Tutorial Site</h1>
<p id="DemoPara1">This is paragraph 1</p>
<button id="DemoButton1"> Check Paragraph 1</button>
<button id="DemoButton2"> Check Paragraph 2 </button>
<button id="DemoButton3"> Check Heading 1 </button>
<script type="text/javascript">
$(document).ready(function () {
$("#DemoButton1").click(function () {
if ($("#DemoPara1").length) {
alert("Paragraph 1 exists");
}
else {
alert("Paragraph 1 does not exist");
}
});
$("#DemoButton2").click(function () {
if ($("#DemoPara2").length) {
alert("Paragraph 2 exists");
}
else {
alert("Paragraph 2 does not exist");
}
});
$("#DemoButton3").click(function () {
if ($("h1").length) {
alert("Heading 1 exists");
}
else {
alert("Heading 1 does not exist");
}
});
});
</script>
</body>
</html>
The code above will check if the paragraphs and heading exist or not by pressing different buttons. See output:
Create a User-Defined Function to Check if an Element Exists Using jQuery
Now, let’s try to create our own method to check if the element exists or not in jQuery. The syntax to create a function exists is:
jQuery.fn.exists = function () {
return this.length > 0;
};
As you can see, we used the length
property to create our own exists
function with jQuery. The length
property is used to return the length of the elements, not to check their existence; that is why we can create our own method once and use it anywhere.
See example:
<!DOCTYPE html>
<html>
<head>
<script src= "https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"> </script>
<title>jQuery Exists</title>
<style>
body {
text-align: center;
}
h1 {
color: lightblue;
font-size: 4.5rem;
}
button {
cursor: pointer;
margin-top: 4rem;
}
</style>
</head>
<body>
<h1>Delftstack | The Best Tutorial Site</h1>
<p id="DemoPara1">This is paragraph 1</p>
<button id="DemoButton1"> Check Paragraph 1</button>
<button id="DemoButton2"> Check Paragraph 2 </button>
<button id="DemoButton3"> Check Heading 1 </button>
<script type="text/javascript">
$(document).ready(function () {
jQuery.fn.exists = function () {
return this.length > 0;
};
$("#DemoButton1").click(function () {
if ($("#DemoPara1").exists()) {
alert("Paragraph 1 exists");
}
else {
alert("Paragraph 1 does not exist");
}
});
$("#DemoButton2").click(function () {
if ($("#DemoPara2").exists()) {
alert("Paragraph 2 exists");
}
else {
alert("Paragraph 2 does not exist");
}
});
$("#DemoButton3").click(function () {
if ($("h1").exists()) {
alert("Heading 1 exists");
}
else {
alert("Heading 1 does not exist");
}
});
});
</script>
</body>
</html>
The code above uses a user-defined function exists
to check if the element exists or not. The output will be similar to the example one.
See output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook