Comprobar si un elemento existe usando jQuery
- Use la propiedad de longitud para verificar si existe un elemento usando jQuery
- Cree una función definida por el usuario para verificar si existe un elemento usando jQuery
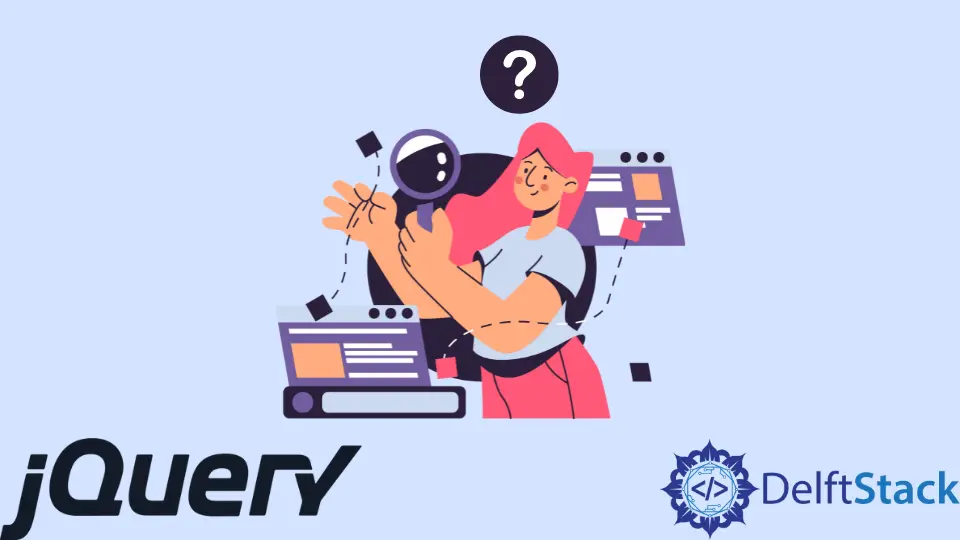
Hay dos métodos para verificar si un elemento existe o no usando jQuery, uno es la propiedad de longitud y el otro es usar métodos para crear nuestro propio método exist()
. Este tutorial demuestra cómo verificar si un elemento existe o no.
Use la propiedad de longitud para verificar si existe un elemento usando jQuery
La propiedad length
de jQuery se puede utilizar para comprobar si el elemento existe o no; devuelve el total de elementos coincidentes. Si length
devuelve 0, el elemento no existe y cualquier otro valor significa que el elemento existe.
La sintaxis de la propiedad length
es:
($("element").length)
La sintaxis anterior devolverá 0 o cualquier otro número.
Probemos un ejemplo para verificar si un elemento existe o no usando la propiedad length. Ver ejemplo:
<!DOCTYPE html>
<html>
<head>
<script src= "https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"> </script>
<title>jQuery Length</title>
<style>
body {
text-align: center;
}
h1 {
color: lightblue;
font-size: 4.5rem;
}
button {
cursor: pointer;
margin-top: 4rem;
}
</style>
</head>
<body>
<h1>Delftstack | The Best Tutorial Site</h1>
<p id="DemoPara1">This is paragraph 1</p>
<button id="DemoButton1"> Check Paragraph 1</button>
<button id="DemoButton2"> Check Paragraph 2 </button>
<button id="DemoButton3"> Check Heading 1 </button>
<script type="text/javascript">
$(document).ready(function () {
$("#DemoButton1").click(function () {
if ($("#DemoPara1").length) {
alert("Paragraph 1 exists");
}
else {
alert("Paragraph 1 does not exist");
}
});
$("#DemoButton2").click(function () {
if ($("#DemoPara2").length) {
alert("Paragraph 2 exists");
}
else {
alert("Paragraph 2 does not exist");
}
});
$("#DemoButton3").click(function () {
if ($("h1").length) {
alert("Heading 1 exists");
}
else {
alert("Heading 1 does not exist");
}
});
});
</script>
</body>
</html>
El código anterior verificará si los párrafos y el encabezado existen o no presionando diferentes botones. Ver salida:
Cree una función definida por el usuario para verificar si existe un elemento usando jQuery
Ahora, intentemos crear nuestro propio método para verificar si el elemento existe o no en jQuery. La sintaxis para crear una función existe es:
jQuery.fn.exists = function () {
return this.length > 0;
};
Como puede ver, usamos la propiedad length
para crear nuestra propia función exist
con jQuery. La propiedad length
se utiliza para devolver la longitud de los elementos, no para comprobar su existencia; es por eso que podemos crear nuestro propio método una vez y usarlo en cualquier lugar.
Ver ejemplo:
<!DOCTYPE html>
<html>
<head>
<script src= "https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"> </script>
<title>jQuery Exists</title>
<style>
body {
text-align: center;
}
h1 {
color: lightblue;
font-size: 4.5rem;
}
button {
cursor: pointer;
margin-top: 4rem;
}
</style>
</head>
<body>
<h1>Delftstack | The Best Tutorial Site</h1>
<p id="DemoPara1">This is paragraph 1</p>
<button id="DemoButton1"> Check Paragraph 1</button>
<button id="DemoButton2"> Check Paragraph 2 </button>
<button id="DemoButton3"> Check Heading 1 </button>
<script type="text/javascript">
$(document).ready(function () {
jQuery.fn.exists = function () {
return this.length > 0;
};
$("#DemoButton1").click(function () {
if ($("#DemoPara1").exists()) {
alert("Paragraph 1 exists");
}
else {
alert("Paragraph 1 does not exist");
}
});
$("#DemoButton2").click(function () {
if ($("#DemoPara2").exists()) {
alert("Paragraph 2 exists");
}
else {
alert("Paragraph 2 does not exist");
}
});
$("#DemoButton3").click(function () {
if ($("h1").exists()) {
alert("Heading 1 exists");
}
else {
alert("Heading 1 does not exist");
}
});
});
</script>
</body>
</html>
El código anterior utiliza una función definida por el usuario existe
para verificar si el elemento existe o no. La salida será similar a la del ejemplo.
Ver salida:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook