使用 jQuery 检查元素是否存在
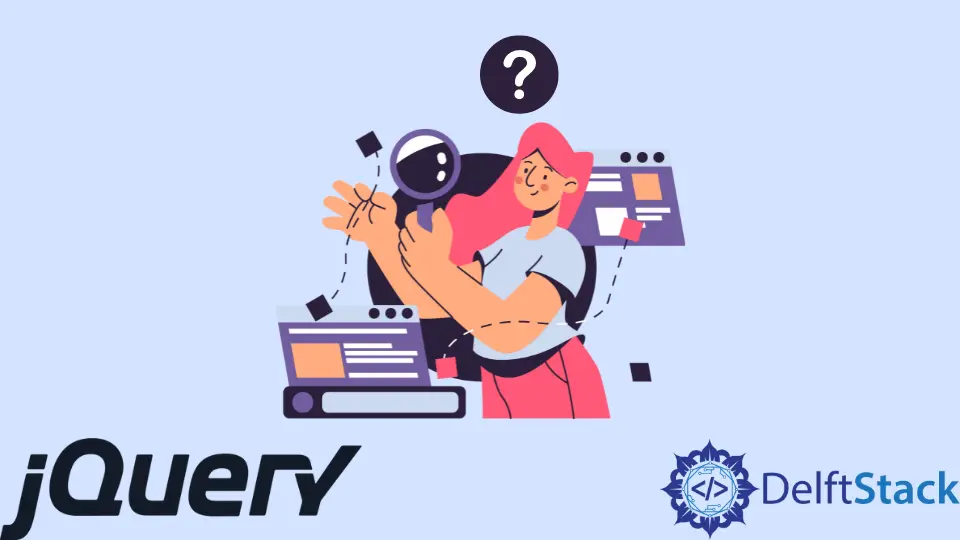
有两种方法可以使用 jQuery 来检查元素是否存在,一种是 length 属性,另一种是使用方法来创建我们自己的 exist()
方法。本教程演示如何检查元素是否存在。
使用 jQuery 使用 Length 属性检查元素是否存在
jQuery 的 length
属性可用于检查元素是否存在;它返回总匹配元素。如果 length 返回 0,则元素不存在,其他任何值表示元素存在。
length
属性的语法是:
($("element").length)
上面的语法将返回 0 或任何其他数字。
让我们尝试一个示例,通过使用 length 属性来检查元素是否存在。参见示例:
<!DOCTYPE html>
<html>
<head>
<script src= "https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"> </script>
<title>jQuery Length</title>
<style>
body {
text-align: center;
}
h1 {
color: lightblue;
font-size: 4.5rem;
}
button {
cursor: pointer;
margin-top: 4rem;
}
</style>
</head>
<body>
<h1>Delftstack | The Best Tutorial Site</h1>
<p id="DemoPara1">This is paragraph 1</p>
<button id="DemoButton1"> Check Paragraph 1</button>
<button id="DemoButton2"> Check Paragraph 2 </button>
<button id="DemoButton3"> Check Heading 1 </button>
<script type="text/javascript">
$(document).ready(function () {
$("#DemoButton1").click(function () {
if ($("#DemoPara1").length) {
alert("Paragraph 1 exists");
}
else {
alert("Paragraph 1 does not exist");
}
});
$("#DemoButton2").click(function () {
if ($("#DemoPara2").length) {
alert("Paragraph 2 exists");
}
else {
alert("Paragraph 2 does not exist");
}
});
$("#DemoButton3").click(function () {
if ($("h1").length) {
alert("Heading 1 exists");
}
else {
alert("Heading 1 does not exist");
}
});
});
</script>
</body>
</html>
上面的代码将通过按下不同的按钮来检查段落和标题是否存在。见输出:
使用 jQuery 创建一个用户定义的函数来检查元素是否存在
现在,让我们尝试创建自己的方法来检查元素在 jQuery 中是否存在。存在创建函数的语法是:
jQuery.fn.exists = function () {
return this.length > 0;
};
如你所见,我们使用 length
属性通过 jQuery 创建了我们自己的 exists
函数。length
属性用于返回元素的长度,而不是检查它们的存在;这就是为什么我们可以创建自己的方法一次并在任何地方使用它。
参见示例:
<!DOCTYPE html>
<html>
<head>
<script src= "https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"> </script>
<title>jQuery Exists</title>
<style>
body {
text-align: center;
}
h1 {
color: lightblue;
font-size: 4.5rem;
}
button {
cursor: pointer;
margin-top: 4rem;
}
</style>
</head>
<body>
<h1>Delftstack | The Best Tutorial Site</h1>
<p id="DemoPara1">This is paragraph 1</p>
<button id="DemoButton1"> Check Paragraph 1</button>
<button id="DemoButton2"> Check Paragraph 2 </button>
<button id="DemoButton3"> Check Heading 1 </button>
<script type="text/javascript">
$(document).ready(function () {
jQuery.fn.exists = function () {
return this.length > 0;
};
$("#DemoButton1").click(function () {
if ($("#DemoPara1").exists()) {
alert("Paragraph 1 exists");
}
else {
alert("Paragraph 1 does not exist");
}
});
$("#DemoButton2").click(function () {
if ($("#DemoPara2").exists()) {
alert("Paragraph 2 exists");
}
else {
alert("Paragraph 2 does not exist");
}
});
$("#DemoButton3").click(function () {
if ($("h1").exists()) {
alert("Heading 1 exists");
}
else {
alert("Heading 1 does not exist");
}
});
});
</script>
</body>
</html>
上面的代码使用用户定义的函数 exists
来检查元素是否存在。输出将类似于示例一。
见输出:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook