How to Get Element by ID in jQuery
-
Using jQuery’s
$("#id")
Selector -
Using
getElementById
for Compatibility -
Using jQuery’s
find()
Method -
Using
each()
to Iterate Over Multiple Elements - Conclusion
- FAQ
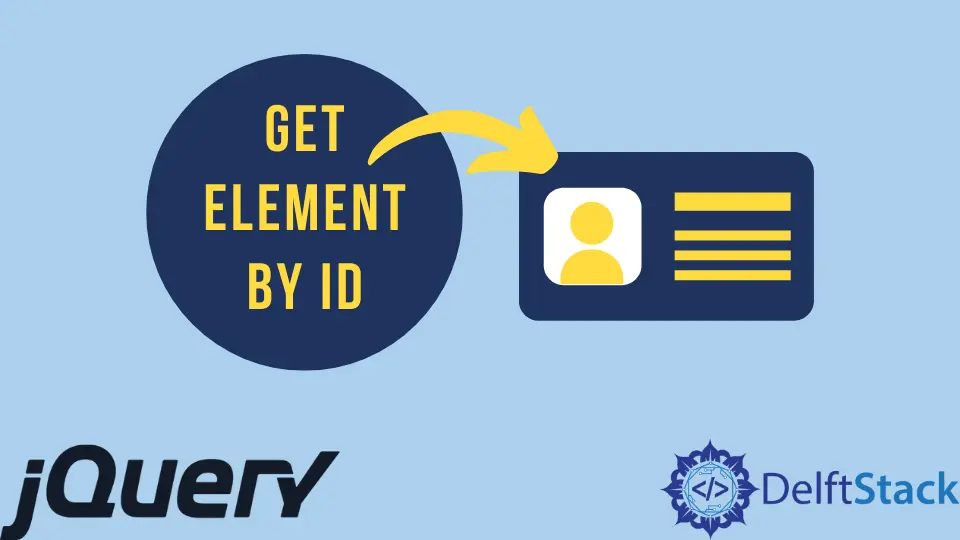
In the world of web development, jQuery has become a staple for simplifying JavaScript tasks, especially when it comes to DOM manipulation. One of the most common operations you’ll perform is retrieving an element by its ID.
This tutorial will walk you through the various methods of getting an element by ID using jQuery, providing you with practical examples and clear explanations. Whether you’re a beginner or just brushing up on your skills, understanding how to effectively use jQuery for this task will enhance your web development toolkit. Let’s dive in and explore how to get an element by ID in jQuery!
Using jQuery’s $("#id")
Selector
The simplest and most commonly used method to get an element by ID in jQuery is by using the $("#id")
selector. This method is straightforward and efficient, allowing you to access any element on your web page that has a specific ID. Below is a basic example demonstrating how to use this method.
$(document).ready(function() {
var element = $("#myElement");
element.css("color", "blue");
});
Output:
This code selects the element with the ID 'myElement' and changes its text color to blue.
In this example, the jQuery code waits for the document to be fully loaded before executing. The $("#myElement")
selector retrieves the element with the ID myElement
. The css
method then changes the text color of that element to blue. This method is not only simple but also very powerful, as it allows you to manipulate the selected element in numerous ways, such as changing styles, adding classes, or even modifying content.
Using getElementById
for Compatibility
While jQuery offers a convenient way to get elements by ID, there may be times when you want to use vanilla JavaScript for better performance or compatibility. The getElementById
method is a native JavaScript method that can be used alongside jQuery. Here’s how you can combine both methods effectively.
$(document).ready(function() {
var element = document.getElementById("myElement");
$(element).css("color", "red");
});
Output:
This code retrieves the element with the ID 'myElement' using vanilla JavaScript and then changes its text color to red using jQuery.
In this example, we first use document.getElementById("myElement")
to get the element with the specified ID. This method returns a reference to the DOM element, which we can then wrap with jQuery’s $()
to utilize jQuery’s methods. By doing so, we can change the text color to red. This approach is beneficial if you are working in a context where jQuery is not fully loaded or if you prefer to use native JavaScript for performance reasons.
Using jQuery’s find()
Method
Another useful method in jQuery is the find()
method, which allows you to search for a specific element within a parent element. This can be particularly handy if you have multiple elements and want to narrow down your selection. Here’s how you can use find()
to get an element by ID.
$(document).ready(function() {
var parentElement = $("#parent");
var childElement = parentElement.find("#myElement");
childElement.css("font-weight", "bold");
});
Output:
This code finds the element with the ID 'myElement' within a parent element and makes its text bold.
In this example, we first select a parent element with the ID parent
. Using the find()
method, we search for the child element with the ID myElement
within that parent. We then change the font weight of the found element to bold. This method is particularly useful in complex DOM structures where elements are nested within others, allowing for more specific selections.
Using each()
to Iterate Over Multiple Elements
Sometimes, you may have multiple elements with the same class or other selectors, and you want to perform actions on each of them. Although IDs should be unique, there are scenarios where you may want to iterate over a collection of elements that share a common attribute. Here’s how you can use the each()
method in conjunction with getting elements by ID.
$(document).ready(function() {
$(".myClass").each(function() {
var element = $(this);
element.css("border", "1px solid green");
});
});
Output:
This code iterates over all elements with the class 'myClass' and adds a green border to each of them.
In this case, we use the jQuery each()
method to loop through all elements with the class myClass
. Inside the loop, $(this)
refers to the current element being processed. We then apply a green border to each element. While this example does not directly use an ID, it demonstrates how jQuery can be used to manipulate multiple elements efficiently, which can be beneficial in various scenarios.
Conclusion
Getting an element by ID in jQuery is a fundamental skill for any web developer. Whether you choose to use jQuery’s $("#id")
selector, combine it with native JavaScript methods, or utilize jQuery’s powerful tools like find()
and each()
, understanding these techniques will significantly enhance your ability to manipulate the DOM effectively. As you continue to explore jQuery, you’ll find that these methods provide a solid foundation for more advanced operations. Happy coding!
FAQ
-
How do I select multiple elements by ID in jQuery?
IDs should be unique in HTML. If you need to select multiple elements, consider using classes instead. -
Can I use jQuery to manipulate elements without an ID?
Yes, you can use classes, attributes, or element types to select and manipulate elements in jQuery. -
What should I do if jQuery is not working?
Ensure that you have included the jQuery library correctly and check for JavaScript errors in the browser console. -
Is it better to use jQuery or vanilla JavaScript?
It depends on your project requirements. jQuery simplifies many tasks, but for performance-sensitive applications, vanilla JavaScript may be more efficient. -
Can I use jQuery with other JavaScript frameworks?
Yes, jQuery can be used alongside other frameworks, but be cautious of potential conflicts with libraries that manipulate the DOM.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook