How to Add ID to an HTML Element Using jQuery
-
Use the
.attr()
Method to Add ID to an HTML Element Using jQuery -
Use the
.add()
Method to Add ID to an HTML Element Using jQuery
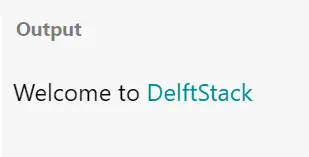
In jQuery, an explicit function solely works to add a class to an HTML element. This method is used as addClass("className")
.
But in the case of adding an id
, no such method is defined. Generally, an id
of an HTML element makes it more different and powerful in terms of specificity.
Here, we will examine two ways of adding an id
to an HTML element. And the whole process will be performed via jQuery conventions.
The first solution is to use the .attr()
method and add the attribute id
and a corresponding value. This will make the id
set for an element.
Next, we will go for an example that can add an id
but is not as defined as the previous one. Here, we will take an HTML element as a parameter in the .add()
method and add the id
.
So, let’s preview the explanation with instances.
Use the .attr()
Method to Add ID to an HTML Element Using jQuery
We will have a p
tag in our body
element of the HTML example, and there is a specific word in the p
tag that we wish to highlight. Also, it will be possible if there is a relevant tag and let it have a unique id
.
So, we will make a span
tag, create an id
for it, and then add some CSS
property to see if the newly created id
works.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<script src="https://code.jquery.com/jquery-3.6.0.min.js" integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4=" crossorigin="anonymous"></script>
<title>attr Id</title>
</head>
<body>
<p>Welcome to <span>DelftStack</span></p>
<script>
$("span").attr("id","intro");
$("#intro").css("color","darkcyan");
</script>
</body>
</html>
Output:
Use the .add()
Method to Add ID to an HTML Element Using jQuery
In this case, we have the p
tag but did not assign a span
under the p
tag. We will fetch the instance of the p
tag, add an HTML
element via the .add()
method, and append it to the p
tag.
It will not showcase its presence until you append the newly created element. Let’s check the code lines.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<script src="https://code.jquery.com/jquery-3.6.0.min.js" integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4=" crossorigin="anonymous"></script>
<title>attr Id</title>
</head>
<body>
<p>Welcome to </p>
<script>
$("p").add("<span id='intro'>DelftStack</span>").appendTo("p");
$("#intro").css("color","royalblue");
</script>
</body>
</html>
Output: