How to Hide Div Element in jQuery
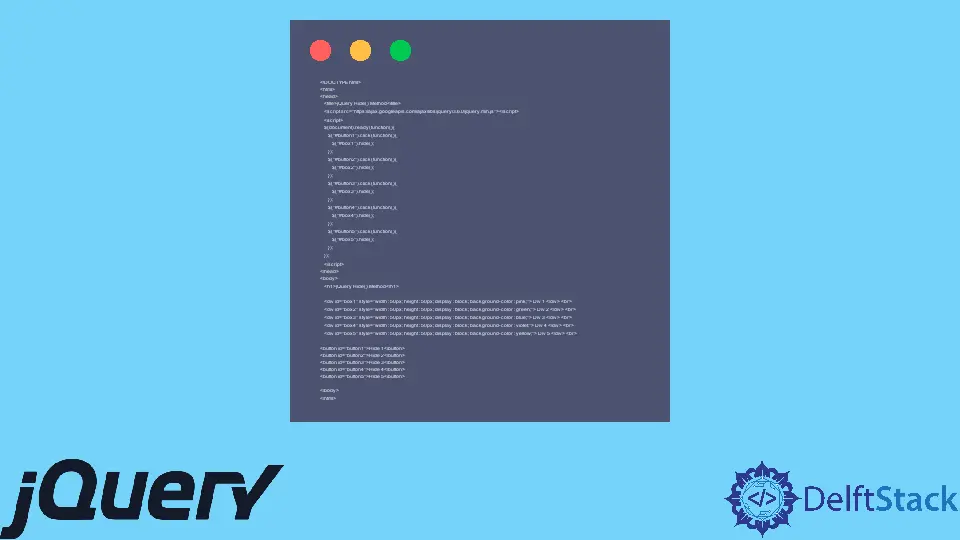
The hide()
is a built-in jQuery function used to hide HTML elements.
This tutorial demonstrates how to use the hide()
method in jQuery.
Use the hide()
Method in jQuery to Hide div
Element
The hide()
method is used to hide the HTML elements works similar to the CSS display:none
. Once the elements are hidden using hide, they cannot be seen again until we change the CSS or use the show()
method.
Syntax:
$(selector).hide(speed,easing,callback)
The selector
is the id, class, or element name. speed
is an optional parameter to specify the time for the hiding effect.
The value can be slow
, fast
, or milliseconds
; the default value is 400 milliseconds. The easing
is also an optional parameter used to specify the speed of the element at different points of the animation; the default value for easing is swing
; it can be changed to linear
; both values have different effects, and the callback
parameter is also an optional function that will be executed once the hide()
method is executed.
Let’s have an example of using the hide()
function without any parameters.
Code:
<!DOCTYPE html>
<html>
<head>
<title>jQuery Hide() Method</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("#button1").click(function(){
$("#box1").hide();
});
$("#button2").click(function(){
$("#box2").hide();
});
$("#button3").click(function(){
$("#box3").hide();
});
$("#button4").click(function(){
$("#box4").hide();
});
$("#button5").click(function(){
$("#box5").hide();
});
});
</script>
</head>
<body>
<h1>jQuery Hide() Method</h1>
<div id="box1" style="width : 50px; height : 50px; display : block; background-color : pink;"> Div 1 </div> <br>
<div id="box2" style="width : 50px; height : 50px; display : block; background-color : green;"> Div 2 </div> <br>
<div id="box3" style="width : 50px; height : 50px; display : block; background-color : blue;"> Div 3 </div> <br>
<div id="box4" style="width : 50px; height : 50px; display : block; background-color : violet;"> Div 4 </div> <br>
<div id="box5" style="width : 50px; height : 50px; display : block; background-color : yellow;"> Div 5 </div> <br>
<button id="button1">Hide 1</button>
<button id="button2">Hide 2</button>
<button id="button3">Hide 3</button>
<button id="button4">Hide 4</button>
<button id="button5">Hide 5</button>
</body>
</html>
The code above will hide the div
by clicking the corresponding button.
Output:
Let’s try the same example with the speed parameter.
Code:
<!DOCTYPE html>
<html>
<head>
<title>jQuery Hide() Method</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("#button1").click(function(){
$("#box1").hide("slow");
});
$("#button2").click(function(){
$("#box2").hide("fast");
});
$("#button3").click(function(){
$("#box3").hide(500);
});
$("#button4").click(function(){
$("#box4").hide(800);
});
$("#button5").click(function(){
$("#box5").hide(1000);
});
});
</script>
</head>
<body>
<h1>jQuery Hide() Method</h1>
<div id="box1" style="width : 50px; height : 50px; display : block; background-color : pink;"> Div 1 </div> <br>
<div id="box2" style="width : 50px; height : 50px; display : block; background-color : green;"> Div 2 </div> <br>
<div id="box3" style="width : 50px; height : 50px; display : block; background-color : blue;"> Div 3 </div> <br>
<div id="box4" style="width : 50px; height : 50px; display : block; background-color : violet;"> Div 4 </div> <br>
<div id="box5" style="width : 50px; height : 50px; display : block; background-color : yellow;"> Div 5 </div> <br>
<button id="button1">Hide 1</button>
<button id="button2">Hide 2</button>
<button id="button3">Hide 3</button>
<button id="button4">Hide 4</button>
<button id="button5">Hide 5</button>
</body>
</html>
The code will hide the div
with the given speed and swing easing.
Output:
Let’s see how the callback parameter works.
Code:
<!DOCTYPE html>
<html>
<head>
<title>jQuery Hide() Method</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("#button1").click(function(){
$("#box1").hide("slow", function(){
alert("Div 1 is hidden");
});
});
$("#button2").click(function(){
$("#box2").hide("fast", function(){
alert("Div 2 is hidden");
});
});
$("#button3").click(function(){
$("#box3").hide(500, function(){
alert("Div 3 is hidden");
});
});
$("#button4").click(function(){
$("#box4").hide(800, function(){
alert("Div 4 is hidden");
});
});
$("#button5").click(function(){
$("#box5").hide(1000, function(){
alert("Div 5 is hidden");
});
});
});
</script>
</head>
<body>
<h1>jQuery Hide() Method</h1>
<div id="box1" style="width : 50px; height : 50px; display : block; background-color : pink;"> Div 1 </div> <br>
<div id="box2" style="width : 50px; height : 50px; display : block; background-color : green;"> Div 2 </div> <br>
<div id="box3" style="width : 50px; height : 50px; display : block; background-color : blue;"> Div 3 </div> <br>
<div id="box4" style="width : 50px; height : 50px; display : block; background-color : violet;"> Div 4 </div> <br>
<div id="box5" style="width : 50px; height : 50px; display : block; background-color : yellow;"> Div 5 </div> <br>
<button id="button1">Hide 1</button>
<button id="button2">Hide 2</button>
<button id="button3">Hide 3</button>
<button id="button4">Hide 4</button>
<button id="button5">Hide 5</button>
</body>
</html>
The code above will alert the div
number each time the div
is hidden.
Output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook