隱藏 jQuery 中的 div 元素
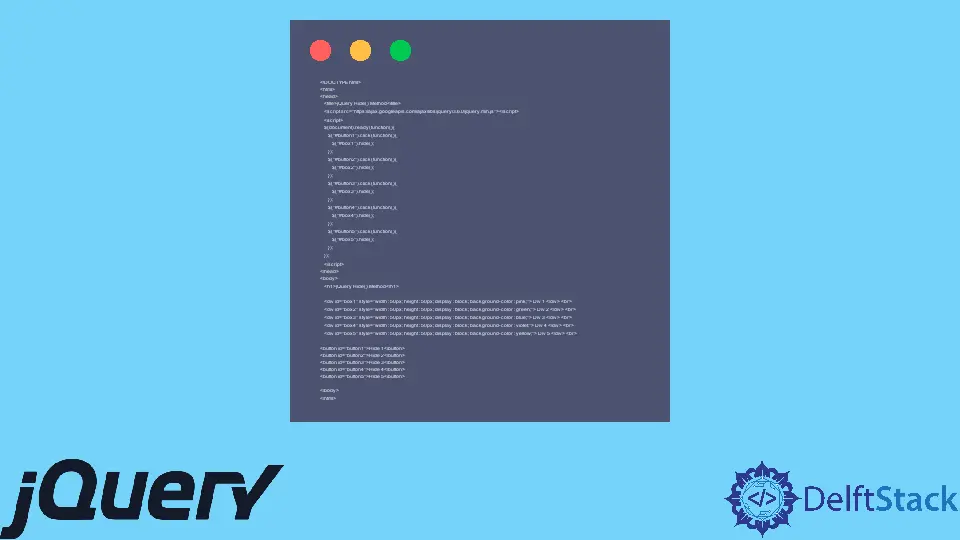
hide()
是一個內建的 jQuery 函式,用於隱藏 HTML 元素。本教程演示瞭如何在 jQuery 中使用 hide()
方法。
使用 jQuery 中的 hide()
方法隱藏 div
元素
hide()
方法用於隱藏 HTML 元素,其工作原理類似於 CSS display:none
。一旦使用 hide 隱藏元素,在我們更改 CSS 或使用 show()
方法之前就無法再次看到它們。
語法:
$(selector).hide(speed,easing,callback)
selector
是 id、類或元素名稱。Speed
是一個可選引數,用於指定隱藏效果的時間。
該值可以是 slow
、fast
或 milliseconds
;預設值為 400 毫秒。easing
也是一個可選引數,用於指定元素在動畫不同點的速度;緩動的預設值為 swing
;它可以更改為線性
;這兩個值有不同的效果,callback
引數也是一個可選函式,一旦 hide()
方法被執行,就會被執行。
讓我們舉一個不帶任何引數使用 hide()
函式的示例。
程式碼:
<!DOCTYPE html>
<html>
<head>
<title>jQuery Hide() Method</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("#button1").click(function(){
$("#box1").hide();
});
$("#button2").click(function(){
$("#box2").hide();
});
$("#button3").click(function(){
$("#box3").hide();
});
$("#button4").click(function(){
$("#box4").hide();
});
$("#button5").click(function(){
$("#box5").hide();
});
});
</script>
</head>
<body>
<h1>jQuery Hide() Method</h1>
<div id="box1" style="width : 50px; height : 50px; display : block; background-color : pink;"> Div 1 </div> <br>
<div id="box2" style="width : 50px; height : 50px; display : block; background-color : green;"> Div 2 </div> <br>
<div id="box3" style="width : 50px; height : 50px; display : block; background-color : blue;"> Div 3 </div> <br>
<div id="box4" style="width : 50px; height : 50px; display : block; background-color : violet;"> Div 4 </div> <br>
<div id="box5" style="width : 50px; height : 50px; display : block; background-color : yellow;"> Div 5 </div> <br>
<button id="button1">Hide 1</button>
<button id="button2">Hide 2</button>
<button id="button3">Hide 3</button>
<button id="button4">Hide 4</button>
<button id="button5">Hide 5</button>
</body>
</html>
上面的程式碼將通過單擊相應的按鈕隱藏 div
。
輸出:
讓我們嘗試使用速度引數的相同示例。
程式碼:
<!DOCTYPE html>
<html>
<head>
<title>jQuery Hide() Method</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("#button1").click(function(){
$("#box1").hide("slow");
});
$("#button2").click(function(){
$("#box2").hide("fast");
});
$("#button3").click(function(){
$("#box3").hide(500);
});
$("#button4").click(function(){
$("#box4").hide(800);
});
$("#button5").click(function(){
$("#box5").hide(1000);
});
});
</script>
</head>
<body>
<h1>jQuery Hide() Method</h1>
<div id="box1" style="width : 50px; height : 50px; display : block; background-color : pink;"> Div 1 </div> <br>
<div id="box2" style="width : 50px; height : 50px; display : block; background-color : green;"> Div 2 </div> <br>
<div id="box3" style="width : 50px; height : 50px; display : block; background-color : blue;"> Div 3 </div> <br>
<div id="box4" style="width : 50px; height : 50px; display : block; background-color : violet;"> Div 4 </div> <br>
<div id="box5" style="width : 50px; height : 50px; display : block; background-color : yellow;"> Div 5 </div> <br>
<button id="button1">Hide 1</button>
<button id="button2">Hide 2</button>
<button id="button3">Hide 3</button>
<button id="button4">Hide 4</button>
<button id="button5">Hide 5</button>
</body>
</html>
該程式碼將隱藏具有給定速度和擺動緩動的 div
。
輸出:
讓我們看看回撥引數是如何工作的。
程式碼:
<!DOCTYPE html>
<html>
<head>
<title>jQuery Hide() Method</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("#button1").click(function(){
$("#box1").hide("slow", function(){
alert("Div 1 is hidden");
});
});
$("#button2").click(function(){
$("#box2").hide("fast", function(){
alert("Div 2 is hidden");
});
});
$("#button3").click(function(){
$("#box3").hide(500, function(){
alert("Div 3 is hidden");
});
});
$("#button4").click(function(){
$("#box4").hide(800, function(){
alert("Div 4 is hidden");
});
});
$("#button5").click(function(){
$("#box5").hide(1000, function(){
alert("Div 5 is hidden");
});
});
});
</script>
</head>
<body>
<h1>jQuery Hide() Method</h1>
<div id="box1" style="width : 50px; height : 50px; display : block; background-color : pink;"> Div 1 </div> <br>
<div id="box2" style="width : 50px; height : 50px; display : block; background-color : green;"> Div 2 </div> <br>
<div id="box3" style="width : 50px; height : 50px; display : block; background-color : blue;"> Div 3 </div> <br>
<div id="box4" style="width : 50px; height : 50px; display : block; background-color : violet;"> Div 4 </div> <br>
<div id="box5" style="width : 50px; height : 50px; display : block; background-color : yellow;"> Div 5 </div> <br>
<button id="button1">Hide 1</button>
<button id="button2">Hide 2</button>
<button id="button3">Hide 3</button>
<button id="button4">Hide 4</button>
<button id="button5">Hide 5</button>
</body>
</html>
每次隱藏 div
時,上面的程式碼都會提醒 div
編號。
輸出:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook