使用 jQuery 移動元素
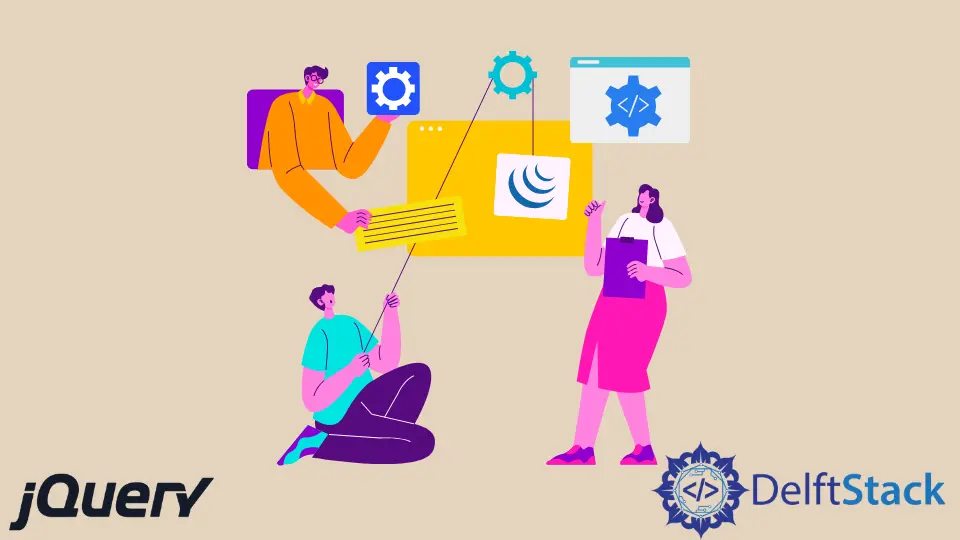
本教程演示瞭如何使用不同的方法在 jQuery 中移動元素。
jQuery 中有不同的方法可用於在 HTML 文件的特定位置移動或插入元素。這些方法包括 appendTo()
、prependTo()
、insertBefore()
、insertAfter()
和其他一些方法。
讓我們討論這些方法並展示一些示例。
使用 appendTo
和 prependTo
使用 jQuery 移動元素
appendTo()
和 prependTo()
方法用於將元素移動到 jQuery 中的另一個元素中。
例如,假設一個 div
包含三個段落,我們想將一個段落移到 div
中。在這種情況下,appendTo()
方法會將新段落移動到三個段落之後的 div
中,而 prependTo()
方法會將段落移動到三個段落之前的 div
。
這兩種方法的語法如下所示:
$("content").appendTo("target");
$("content").prependTo("target");
其中,
content
是要移動的內容。target
是元素將被移動的位置。
讓我們嘗試一下 appendTo()
方法的示例:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery appendTo</title>
<style>
#TargetDiv{
padding: 30px;
background: lightblue;
}
#ContentDiv{
padding: 30px;
margin: 40px 0;
border: 3px solid;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#ContentDiv").appendTo("#TargetDiv");
$(this).hide();
});
});
</script>
</head>
<body>
<div id="ContentDiv">
<h1>Hello Delftstack</h1>
<p>This is delftstack.com the best site for tutorials.</p>
<button type="button">Move Element</button>
</div>
<div id="TargetDiv">
<h1>DELFTSTACK</h1>
</div>
</body>
</html>
上面的程式碼會將 ContentDiv
移動到 div
中其他元素之後的 TargetDiv
。見輸出:
現在,讓我們為 prependTo()
方法實現相同的示例。參見示例:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery prependTo</title>
<style>
#TargetDiv{
padding: 30px;
background: lightblue;
}
#ContentDiv{
padding: 30px;
margin: 40px 0;
border: 3px solid;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#ContentDiv").prependTo("#TargetDiv");
$(this).hide();
});
});
</script>
</head>
<body>
<div id="ContentDiv">
<h1>Hello Delftstack</h1>
<p>This is delftstack.com the best site for tutorials.</p>
<button type="button">Move Element</button>
</div>
<div id="TargetDiv">
<h1>DELFTSTACK</h1>
</div>
</body>
</html>
上面的程式碼會將 ContentDiv
移動到 TargetDiv
在 div
中的其他元素之前。見輸出:
在 jQuery 中使用 insertBefore()
和 insertAfter()
移動元素
insertBefore()
和 insertAfter()
方法用於在其他元素之前和之後移動元素。這些方法的語法如下所示:
$("content").insertBefore("target");
$("content").insertAfter("target");
其中,
content
是要移動的內容。target
是內容將被移動的位置之後或之前的位置。
讓我們嘗試一個 insertBefore()
方法的示例:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery insertBefore</title>
<style>
#TargetDiv{
padding: 30px;
background: lightblue;
}
#ContentDiv{
padding: 30px;
margin: 40px 0;
border: 3px solid;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#ContentDiv").insertBefore("#TargetDiv");
$(this).hide();
});
});
</script>
</head>
<body>
<div id="TargetDiv">
<h1>DELFTSTACK</h1>
</div>
<div id="ContentDiv">
<h1>Hello Delftstack</h1>
<p>This is delftstack.com the best site for tutorials.</p>
<button type="button">Move Element</button>
</div>
</body>
</html>
上面的程式碼會將內容元素移動到目標元素之前。見輸出:
現在,讓我們試試 insertAfter()
方法的示例:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery insertAfter</title>
<style>
#TargetDiv{
padding: 30px;
background: lightblue;
}
#ContentDiv{
padding: 30px;
margin: 40px 0;
border: 3px solid;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#ContentDiv").insertAfter("#TargetDiv");
$(this).hide();
});
});
</script>
</head>
<body>
<div id="ContentDiv">
<h1>Hello Delftstack</h1>
<p>This is delftstack.com the best site for tutorials.</p>
<button type="button">Move Element</button>
</div>
<div id="TargetDiv">
<h1>DELFTSTACK</h1>
</div>
</body>
</html>
上面的程式碼會將內容移動到目標之後。見輸出:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook