jQuery を使用して要素を移動する
-
appendTo
とprependTo
を使用して、jQuery を使用して要素を移動する -
jQuery を使用して要素を移動するには、
insertBefore()
およびinsertAfter()
を使用する
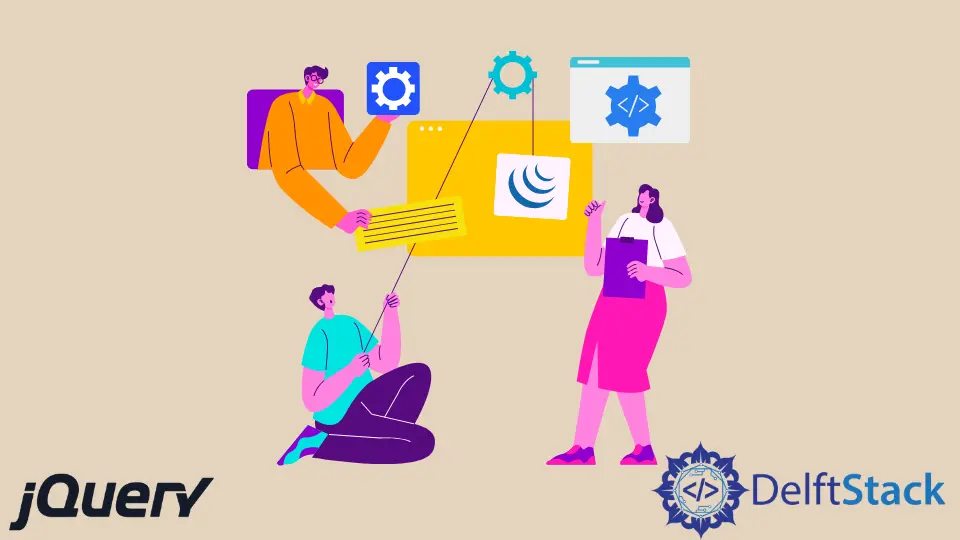
このチュートリアルでは、さまざまなメソッドを使用して jQuery で要素を移動する方法を示します。
jQuery には、HTML ドキュメントの特定の位置に要素を移動または挿入するために使用できるさまざまなメソッドがあります。これらのメソッドには、appendTo()
、prependTo()
、insertBefore()
、insertAfter()
、およびその他のメソッドが含まれます。
方法について説明し、いくつかの例を示しましょう。
appendTo
と prependTo
を使用して、jQuery を使用して要素を移動する
appendTo()
および prependTo()
メソッドは、要素を jQuery の別の要素に移動するために使用されます。
たとえば、div
に 3つの段落が含まれていて、もう 1つの段落を div
に移動するとします。その場合、appendTo()
メソッドは新しい段落を 3つの段落の後に div
に移動し、prependTo()
メソッドは段落を 3つの段落の前の div
に移動します。
両方のメソッドの構文を以下に示します。
$("content").appendTo("target");
$("content").prependTo("target");
ここで:
コンテンツ
は移動するコンテンツです。ターゲット
は、要素が移動される位置です。
appendTo()
メソッドの例を試してみましょう。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery appendTo</title>
<style>
#TargetDiv{
padding: 30px;
background: lightblue;
}
#ContentDiv{
padding: 30px;
margin: 40px 0;
border: 3px solid;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#ContentDiv").appendTo("#TargetDiv");
$(this).hide();
});
});
</script>
</head>
<body>
<div id="ContentDiv">
<h1>Hello Delftstack</h1>
<p>This is delftstack.com the best site for tutorials.</p>
<button type="button">Move Element</button>
</div>
<div id="TargetDiv">
<h1>DELFTSTACK</h1>
</div>
</body>
</html>
上記のコードは、div
の他の要素の後に ContentDiv
を TargetDiv
に移動します。出力を参照してください:
それでは、prependTo()
メソッドに同じ例を実装しましょう。例を参照してください:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery prependTo</title>
<style>
#TargetDiv{
padding: 30px;
background: lightblue;
}
#ContentDiv{
padding: 30px;
margin: 40px 0;
border: 3px solid;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#ContentDiv").prependTo("#TargetDiv");
$(this).hide();
});
});
</script>
</head>
<body>
<div id="ContentDiv">
<h1>Hello Delftstack</h1>
<p>This is delftstack.com the best site for tutorials.</p>
<button type="button">Move Element</button>
</div>
<div id="TargetDiv">
<h1>DELFTSTACK</h1>
</div>
</body>
</html>
上記のコードは、ContentDiv
を div
の他の要素の前に TargetDiv
に移動します。出力を参照してください:
jQuery を使用して要素を移動するには、insertBefore()
および insertAfter()
を使用する
insertBefore()
および insertAfter()
メソッドは、他の要素の前後に要素を移動するために使用されます。これらのメソッドの構文を以下に示します。
$("content").insertBefore("target");
$("content").insertAfter("target");
ここで:
コンテンツ
は移動するコンテンツです。ターゲット
は、コンテンツが移動される前後の位置です。
insertBefore()
メソッドの例を試してみましょう。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery insertBefore</title>
<style>
#TargetDiv{
padding: 30px;
background: lightblue;
}
#ContentDiv{
padding: 30px;
margin: 40px 0;
border: 3px solid;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#ContentDiv").insertBefore("#TargetDiv");
$(this).hide();
});
});
</script>
</head>
<body>
<div id="TargetDiv">
<h1>DELFTSTACK</h1>
</div>
<div id="ContentDiv">
<h1>Hello Delftstack</h1>
<p>This is delftstack.com the best site for tutorials.</p>
<button type="button">Move Element</button>
</div>
</body>
</html>
上記のコードは、コンテンツ要素をターゲット要素の前に移動します。出力を参照してください:
それでは、insertAfter()
メソッドの例を試してみましょう。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery insertAfter</title>
<style>
#TargetDiv{
padding: 30px;
background: lightblue;
}
#ContentDiv{
padding: 30px;
margin: 40px 0;
border: 3px solid;
}
</style>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script>
$(document).ready(function(){
$("button").click(function(){
$("#ContentDiv").insertAfter("#TargetDiv");
$(this).hide();
});
});
</script>
</head>
<body>
<div id="ContentDiv">
<h1>Hello Delftstack</h1>
<p>This is delftstack.com the best site for tutorials.</p>
<button type="button">Move Element</button>
</div>
<div id="TargetDiv">
<h1>DELFTSTACK</h1>
</div>
</body>
</html>
上記のコードは、コンテンツをターゲットの後に移動します。出力を参照してください:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook