jQuery에서 요소 만들기
- jQuery에서 요소 만들기
-
append()
메소드를 사용하여 jQuery에서 요소 생성 -
prepend()
메서드를 사용하여 jQuery에서 요소 생성 -
after()
및before()
메서드를 사용하여 jQuery에서 요소 생성
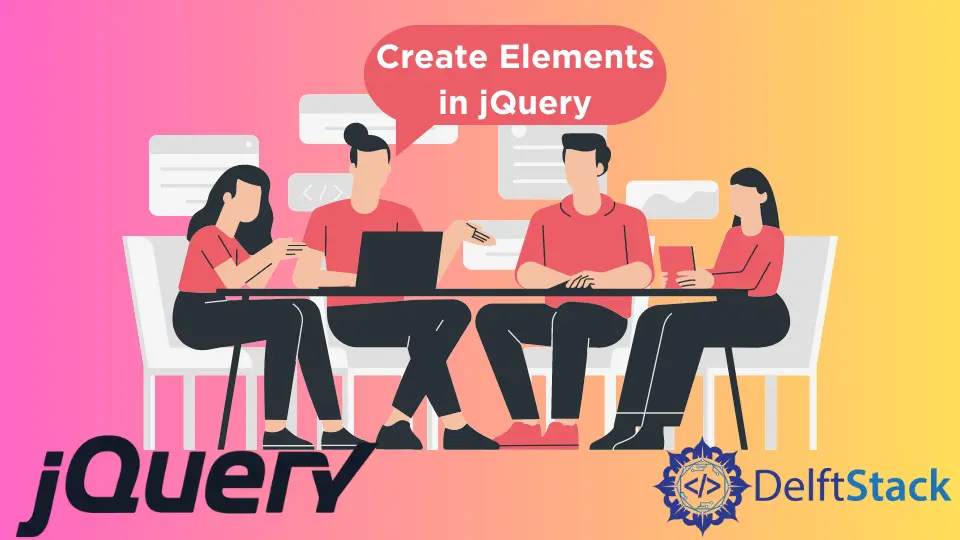
createElement()
는 핵심 JavaScript에서 HTML 요소를 생성하는 메소드입니다. jQuery에는 유사한 작업을 수행하는 몇 가지 방법이 있습니다.
이 튜토리얼은 jQuery에서 HTML 요소를 생성하는 방법을 보여줍니다.
jQuery에서 요소 만들기
jQuery에는 HTML 요소를 만드는 네 가지 방법이 있습니다.
append()
- 이 메서드는 선택한 요소의 끝에 요소를 추가합니다.prepend()
- 이 메서드는 선택한 요소의 시작 부분에 요소를 추가합니다.after()
- 이 메서드는 선택한 요소 뒤에 요소를 추가합니다.before()
- 이 메서드는 선택한 요소 앞에 요소를 추가합니다.
각 방법에 대한 예를 논의하고 시도합시다.
append()
메소드를 사용하여 jQuery에서 요소 생성
append()
메소드는 선택한 요소의 끝에 요소를 추가합니다. 구문은 하나의 HTML 요소를 추가하는 것으로 간단합니다.
예를 참조하십시오.
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("#DemoButton").click(function(){
$("#two").append(" <p> This is paragraph three </p>");
});
});
</script>
</head>
<body>
<p>This is paragraph One.</p>
<p id="two">This is paragraph two.</p>
<button id="DemoButton">Append paragraph</button>
</body>
</html>
위의 코드는 한 번에 하나의 HTML 단락 요소를 추가합니다. 출력 참조:
append()
메서드를 사용하여 여러 요소를 만들 수도 있습니다. 예를 참조하십시오.
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
function append_paragraphs() {
var ELement1 = "<p>This is a paragraph two. </p>"; // paragraph with HTML
var ELement2 = $("<p></p>").text("This is a paragraph three."); // paragraph with jQuery
var ELement3 = document.createElement("p");
ELement3.innerHTML = "This is a paragraph four"; // paragraph with DOM
$("body").append(ELement1, ELement2, ELement3); // Append all paragraph elements
}
</script>
</head>
<body>
<p>This is a paragraph one.</p>
<button onclick="append_paragraphs()">Append Paragraphs</button>
</body>
</html>
위의 코드는 append()
메서드를 사용하여 세 개의 새 단락을 만듭니다. 출력 참조:
prepend()
메서드를 사용하여 jQuery에서 요소 생성
prepend()
메서드는 선택한 요소의 시작 부분에 요소를 추가합니다. 구문은 하나의 HTML 요소에 대해 간단합니다.
예를 참조하십시오.
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("#DemoButton").click(function(){
$("#two").prepend(" <p> This is the new paragraph</p>");
});
});
</script>
</head>
<body>
<p>This is paragraph One.</p>
<p id="two">This is paragraph two.</p>
<button id="DemoButton">Append paragraph</button>
</body>
</html>
코드는 두 번째 단락 앞에 단락을 추가합니다. 출력 참조:
마찬가지로 여러 요소를 앞에 추가합니다. 예를 참조하십시오.
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
function Prepend_paragraphs() {
var ELement1 = "<p>This is a paragraph one. </p>"; // paragraph with HTML
var ELement2 = $("<p></p>").text("This is a paragraph two."); // paragraph with jQuery
var ELement3 = document.createElement("p");
ELement3.innerHTML = "This is a paragraph three"; // paragraph with DOM
$("body").prepend(ELement1, ELement2, ELement3); // Prepend all paragraph elements
}
</script>
</head>
<body>
<p>This is a the last paragraph.</p>
<button onclick="Prepend_paragraphs()">Prepend Paragraphs</button>
</body>
</html>
위의 코드는 주어진 요소 앞에 여러 요소를 생성합니다. 출력 참조:
after()
및 before()
메서드를 사용하여 jQuery에서 요소 생성
after()
메소드는 주어진 요소 뒤에 요소를 생성하고 유사하게 before()
메소드는 주어진 요소 앞에 요소를 생성합니다.
아래 두 가지 방법에 대한 예를 참조하십시오.
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
$(document).ready(function(){
$("#button1").click(function(){
$("h1").before("<b> Element Before </b>");
});
$("#button2").click(function(){
$("h1").after("<b> Element After </b>");
});
});
</script>
</head>
<body>
<h1>Delfstack</h1>
<br><br>
<button id="button1">Insert element before</button>
<button id="button2">Insert element after</button>
</body>
</html>
위의 코드는 주어진 요소 앞과 뒤에 요소를 생성합니다. 출력 참조:
마찬가지로 after()
및 before()
메서드를 사용하여 여러 요소를 만들 수 있습니다. 예를 참조하십시오.
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
function After_Element() {
var Element1 = "<b>This </b>"; // element with HTML
var Element2 = $("<b></b>").text("is "); //with jQuery
var Element3 = document.createElement("b"); // with DOM
Element3.innerHTML = "Delftstack.com.";
$("h1").after(Element1, Element2, Element3); // Insert new elements after h1
}
function Before_Element() {
var Element1 = "<b>This </b>"; // element with HTML
var Element2 = $("<b></b>").text("is "); //with jQuery
var Element3 = document.createElement("b"); // with DOM
Element3.innerHTML = "Delftstack.com.";
$("h1").before(Element1, Element2, Element3); // Insert new elements before h1
}
</script>
</head>
<body>
<h1>Delfstack</h1>
<br><br>
<p>Create Elements after the h1.</p>
<button onclick="After_Element()">Insert after</button>
<p>Create Elements before the h1.</p>
<button onclick="Before_Element()">Insert before</button>
</body>
</html>
위의 코드는 주어진 요소 앞뒤에 여러 요소를 추가합니다. 출력 참조:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook