How to Replace a String in JavaScript
-
Use
split()
&join()
Methods to Replace a String in JavaScript -
Replicate PHP
str_replace()
Function in JavaScript to Replace a String
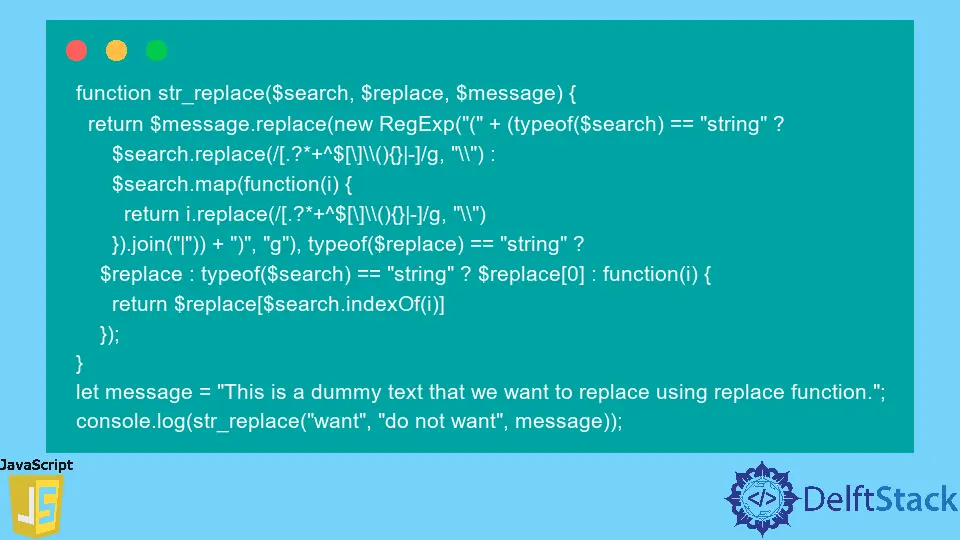
There are multiple ways to replace a string that includes replace()
method, regular expression
, split()
& join()
together, and a replica of PHP str_replace()
method.
You can find a detailed article here that explains how to replace a string in JavaScript by using the replace()
method with and without regex
.
This tutorial talks about how to replicate PHP str_replace()
function in JavaScript and the use of split()
& join()
methods together to replace a string in JavaScript. You can find more on split()
and join()
here.
Use split()
& join()
Methods to Replace a String in JavaScript
The split()
method splits the original string based on the separator
. It outputs the new array of substrings without changing the original string.
The join()
function joins all array’s elements based on the separator
. It returns a new string without updating the original string.
JavaScript Code:
let message =
'This is a dummy text that we want to replace using replace function.';
let new_message = message.split('want').join('do not want');
console.log(new_message);
Output:
"This is a dummy text that we do not want to replace using replace function."
The split()
function splits the message
where it finds want
and returns two substrings "This is a dummy text that we "
and " to replace using replace function."
. Remember that it does not remove white spaces when it breaks the string.
The join()
method joins these two substrings with do not want
and outputs as "This is a dummy text that we do not want to replace using replace function."
.
The code below demonstrates how each function operates and provides a more detailed output.
JavaScript Code:
let message =
'This is a dummy text that we want to replace using replace function.';
let split_message = message.split('want')
let new_message = split_message.join('do not want');
console.log(message);
console.log(split_message);
console.log(new_message);
Output:
"This is a dummy text that we want to replace using replace function."
["This is a dummy text that we ", " to replace using replace function."]
"This is a dummy text that we do not want to replace using replace function."
Replicate PHP str_replace()
Function in JavaScript to Replace a String
JavaScript Code:
function str_replace($searchString, $replaceString, $message) {
// We create regext to find the occurrences
var regex;
// If the $searchString is a string
if (typeof ($searchString) == 'string') {
// Escape all the characters used by regex
$searchString = $searchString.replace(/[.?*+^$[\]\\(){}|-]/g, '\\');
regex = new RegExp('(' + $searchString + ')', 'g');
} else {
// Escape all the characters used by regex
$searchString = $searchString.map(function(i) {
return i.replace(/[.?*+^$[\]\\(){}|-]/g, '\\');
});
regex = new RegExp('(' + $searchString.join('|') + ')', 'g');
}
// we create the replacement
var replacement;
// If the $searchString is a string
if (typeof ($replaceString) == 'string') {
replacement = $replaceString;
} else {
// If the $searchString is a string and the $replaceString an array
if (typeof ($searchString) == 'string') {
replacement = $replaceString[0];
} else {
// If the $searchString and $replaceString are arrays
replacement = function(i) {
return $replaceString[$searchString.indexOf(i)];
}
}
}
return $message.replace(regex, replacement);
}
let message =
'This is a dummy text that we want to replace using replace function.';
console.log(str_replace('want', 'do not want', message));
Output:
"This is a dummy text that we do not want to replace using replace function."
For the example above, the str_replace()
method takes three parameters: $searchString
, $replaceString
, and $message
.
It creates the regex
first (/[want]/g
), considering if the $searchString
is of type string
and not. Then, we create the replacement
considering various situations.
For instance, if $searchString
is a string
or not. If not, check if the $searchString
is a string
and the $replaceString
an array or the $searchString
and $replaceString
both are arrays.
Lastly, we use this regex
and replacement
inside the replace()
method to get the desired output. We can optimize the long code given above to get the exact output.
JavaScript Code:
function str_replace($search, $replace, $message) {
return $message.replace(
new RegExp(
'(' +
(typeof ($search) == 'string' ?
$search.replace(/[.?*+^$[\]\\(){}|-]/g, '\\') :
$search
.map(function(i) {
return i.replace(/[.?*+^$[\]\\(){}|-]/g, '\\')
})
.join('|')) +
')',
'g'),
typeof ($replace) == 'string' ? $replace :
typeof ($search) == 'string' ? $replace[0] :
function(i) {
return $replace[$search.indexOf(i)]
});
}
let message =
'This is a dummy text that we want to replace using replace function.';
console.log(str_replace('want', 'do not want', message));
Output:
"This is a dummy text that we do not want to replace using replace function."