JavaScript Array Join() Method
-
Syntax of JavaScript Array
join()
Method -
Example 1: Use the
Array.join()
Method to Join an Array of String -
Example 2: Use the
Array.join()
Method With Different Separator Values -
Example 3: Use the
Array.join()
Method With an Empty Array -
Example 4: Use the
Array.join()
Method With Array-Like Objects
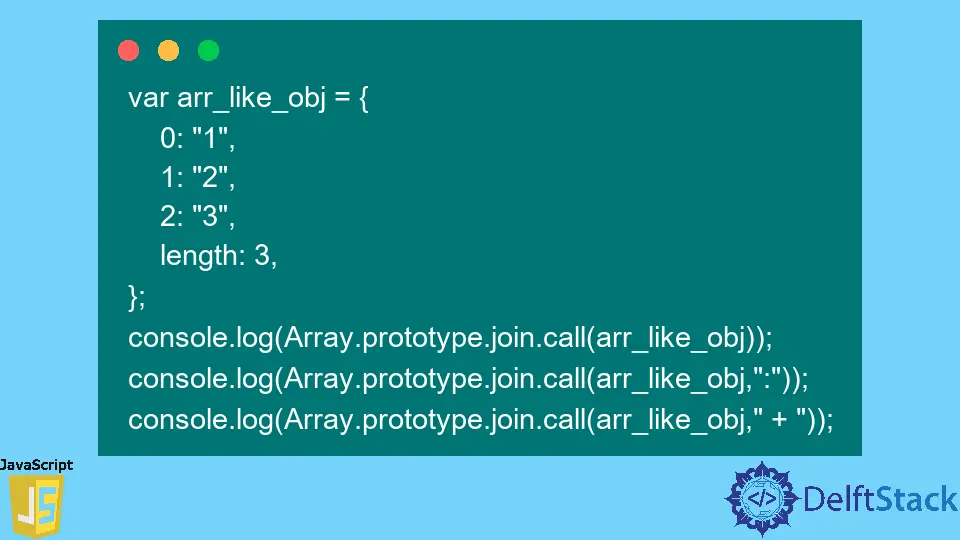
In JavaScript, we can use the array.join()
method to join all the elements of the array in a single string. Also, the array.join()
method doesn’t change the original array and returns the new single string, which contains all the array elements in the respective order.
Syntax of JavaScript Array join()
Method
let values = ["Delft", "Stack"];
values.join();
values.join(separator);
Parameters
separator
- It is an optional parameter. It is a delimiter to separate the adjacent array elements in the returned string when we join them using the array.join()
method.
The default value of separator
is ,
. When we use empty_string
as a separator, the method joins array elements without any space between them.
Return
The array.join()
method returns a single string after joining all array elements by a separator.
Example 1: Use the Array.join()
Method to Join an Array of String
We can use the array.join()
method to join all array elements in a single string.
In the example below, we have created the single array with the different string values and are using the array.join()
method to join all elements in a new single string. Also, in the output, users can see that the original array remains unchanged.
let values = ["Welcome","to","Delft","Stack","!"];
console.log(values.join());
console.log(values);
Output:
Welcome,to,Delft,Stack,!
[ 'Welcome', 'to', 'Delft', 'Stack', '!' ]
Example 2: Use the Array.join()
Method With Different Separator Values
In this section, we will use the optional separator
parameter with the array.join()
method to separate the adjacent array elements with the different separator
values. The value of separator
can be a string or a single character.
In the example below, we have used the different characters and strings as a separator
value, and users can observe the example’s output. When we use the empty_string
as a separator
, it contacts the array elements without any space between them.
let welcome_array = ["Welcome","to","JavaScript","World","!"];
console.log(welcome_array.join());
console.log(welcome_array.join(""));
console.log(welcome_array.join(":"));
console.log(welcome_array.join("+"));
console.log(welcome_array.join(" ab "));
console.log(welcome_array.join("|"));
Output:
Welcome,to,JavaScript,World,!
WelcometoJavaScriptWorld!
Welcome:to:JavaScript:World:!
Welcome+to+JavaScript+World+!
Welcome ab to ab JavaScript ab World ab !
Welcome|to|JavaScript|World|!
Example 3: Use the Array.join()
Method With an Empty Array
When we invoke the array.join()
method on the empty array, it returns the empty_string
.
In the example below, we have created the empty array whose length is 0 and invoked the array.join()
method. In the output, users can see that it has returned the ""
.
const values = [];
console.log(values.join());
Output:
""
Example 4: Use the Array.join()
Method With Array-Like Objects
The meaning of the array-like objects is the objects which have a length
property and contains a positive length value.
In the example below, we have created the arr_like_obj
, which contains the key-value pair and length
property. We have invoked the join()
method on arr_like_obj
using the Array.prototype.join.call()
.
Also, users can see how we have passed the separator
as a parameter. Furthermore, we have used the different separator
with the arr_like_obj
.
var arr_like_obj = {
0: "1",
1: "2",
2: "3",
length: 3,
};
console.log(Array.prototype.join.call(arr_like_obj));
console.log(Array.prototype.join.call(arr_like_obj,":"));
console.log(Array.prototype.join.call(arr_like_obj," + "));
Output:
1,2,3
1:2:3
1 + 2 + 3
We have seen the different examples of the array.join()
method considering the different scenarios in this article.