在 JavaScript 中替換字串
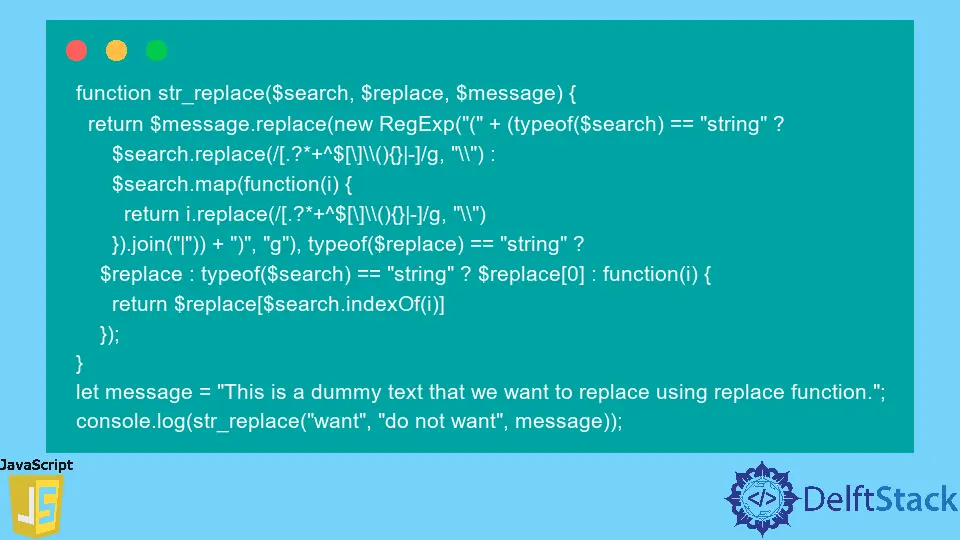
替換字串的方法有多種,包括 replace()
方法、regular expression
、split()
和 join()
一起,以及 PHP str_replace()
方法的副本。
本教程討論瞭如何在 JavaScript 中複製 PHP str_replace()
函式,以及一起使用 split()
和 join()
方法來替換 JavaScript 中的字串。你可以在這裡找到更多關於 split()
和 join()
的資訊。
在 JavaScript 中使用 split()
和 join()
方法替換字串
split()
方法根據 separator
拆分原始字串。它在不更改原始字串的情況下輸出新的子字串陣列。
join()
函式根據 separator
連線所有陣列元素。它返回一個新字串而不更新原始字串。
JavaScript 程式碼:
let message =
'This is a dummy text that we want to replace using replace function.';
let new_message = message.split('want').join('do not want');
console.log(new_message);
輸出:
"This is a dummy text that we do not want to replace using replace function."
split()
函式在找到 want
的位置拆分 message
並返回兩個子字串 "This is a dummy text that we "
和 " to replace using replace function."
。請記住,它在破壞字串時不會刪除空格。
join()
方法將這兩個子字串與 do not want
連線起來,並輸出為 "This is a dummy text, we don't want to replace using replace function."
。
下面的程式碼演示了每個函式如何操作並提供更詳細的輸出。
JavaScript 程式碼:
let message =
'This is a dummy text that we want to replace using replace function.';
let split_message = message.split('want')
let new_message = split_message.join('do not want');
console.log(message);
console.log(split_message);
console.log(new_message);
輸出:
"This is a dummy text that we want to replace using replace function."
["This is a dummy text that we ", " to replace using replace function."]
"This is a dummy text that we do not want to replace using replace function."
在 JavaScript 中複製 PHP str_replace()
函式來替換字串
JavaScript 程式碼:
function str_replace($searchString, $replaceString, $message) {
// We create regext to find the occurrences
var regex;
// If the $searchString is a string
if (typeof ($searchString) == 'string') {
// Escape all the characters used by regex
$searchString = $searchString.replace(/[.?*+^$[\]\\(){}|-]/g, '\\');
regex = new RegExp('(' + $searchString + ')', 'g');
} else {
// Escape all the characters used by regex
$searchString = $searchString.map(function(i) {
return i.replace(/[.?*+^$[\]\\(){}|-]/g, '\\');
});
regex = new RegExp('(' + $searchString.join('|') + ')', 'g');
}
// we create the replacement
var replacement;
// If the $searchString is a string
if (typeof ($replaceString) == 'string') {
replacement = $replaceString;
} else {
// If the $searchString is a string and the $replaceString an array
if (typeof ($searchString) == 'string') {
replacement = $replaceString[0];
} else {
// If the $searchString and $replaceString are arrays
replacement = function(i) {
return $replaceString[$searchString.indexOf(i)];
}
}
}
return $message.replace(regex, replacement);
}
let message =
'This is a dummy text that we want to replace using replace function.';
console.log(str_replace('want', 'do not want', message));
輸出:
"This is a dummy text that we do not want to replace using replace function."
對於上面的示例,str_replace()
方法採用三個引數:$searchString
、$replaceString
和 $message
。
它首先建立 regex
(/[want]/g
),考慮 $searchString
是否屬於 string
型別而不是。然後,我們考慮各種情況建立替換
。
例如,如果 $searchString
是否是 string
。如果不是,請檢查 $searchString
是否是 string
和 $replaceString
是否是陣列或 $searchString
和 $replaceString
是否都是陣列。
最後,我們在 replace()
方法中使用此 regex
和 replacement
來獲得所需的輸出。我們可以優化上面給出的長程式碼以獲得準確的輸出。
JavaScript 程式碼:
function str_replace($search, $replace, $message) {
return $message.replace(
new RegExp(
'(' +
(typeof ($search) == 'string' ?
$search.replace(/[.?*+^$[\]\\(){}|-]/g, '\\') :
$search
.map(function(i) {
return i.replace(/[.?*+^$[\]\\(){}|-]/g, '\\')
})
.join('|')) +
')',
'g'),
typeof ($replace) == 'string' ? $replace :
typeof ($search) == 'string' ? $replace[0] :
function(i) {
return $replace[$search.indexOf(i)]
});
}
let message =
'This is a dummy text that we want to replace using replace function.';
console.log(str_replace('want', 'do not want', message));
輸出:
"This is a dummy text that we do not want to replace using replace function."