How to Read Text File in JavaScript
-
Use the
blob.text()
Function to Read Text Files in JavaScript -
Use the
FileReader
Interface to Read Text Files in JavaScript
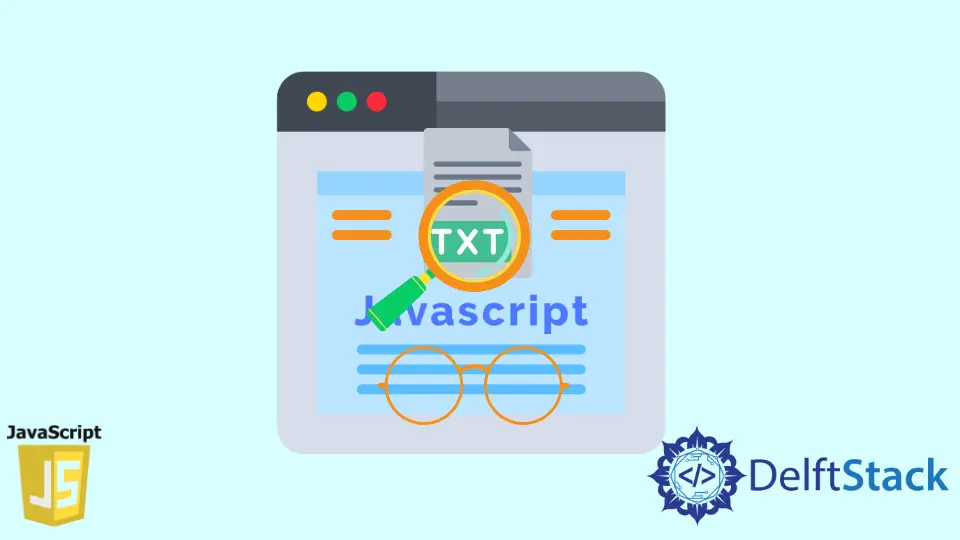
JavaScript allows web pages to read and display elements dynamically. In this tutorial, we will learn how to read a text file in JavaScript.
Use the blob.text()
Function to Read Text Files in JavaScript
The Blob interface’s blob.text()
method delivers a promise that resolves to a string containing the blob’s contents, interpreted as UTF-8. We can use it to read local files.
The <input>
tag can be used to select the required file. After selecting the file, we will read its contents using the blob.text()
function. We display the output on the webpage.
See the following code.
<!DOCTYPE html>
<html>
<head>
<title>Read Text File Tutorial</title>
</head>
<body>
<input type="file" onchange="loadFile(this.files[0])">
<br>
<pre id="output"></pre>
<script>
async function loadFile(file) {
let text = await file.text();
document.getElementById('output').textContent = text;
}
</script>
</body>
</html>
In the above example, we have created an input field of type file
, which lets us give a file as an input. Then specified a <pre>
tag. In HTML, the <pre>
element is used to specify a block of preformatted text that includes text spaces, line breaks, tabs, and other formatting characters that web browsers ignore. The JavaScript code is within the <script>
tags.
Use the FileReader
Interface to Read Text Files in JavaScript
The FileReader
object allows web applications to asynchronously read the contents of files (or raw data buffers) saved on the user’s computer. We will use FileReader.readAsText()
method to read the text file.
For example,
<!DOCTYPE html>
<html>
<head>
<title>Read Text File Tutorial</title>
</head>
<body>
<input type="file" name="inputFile" id="inputFile">
<br>
<pre id="output"></pre>
<script type="text/javascript">
document.getElementById('inputFile').addEventListener('change', function() {
var file = new FileReader();
file.onload = () => {
document.getElementById('output').textContent = file.result;
}
file.readAsText(this.files[0]);
});
</script>
</body>
</html>