Instantiate a File Object in JavaScript
- Instantiate a File for Variety Data Types in JavaScript
- Take an Image for File Instantiation in JavaScript
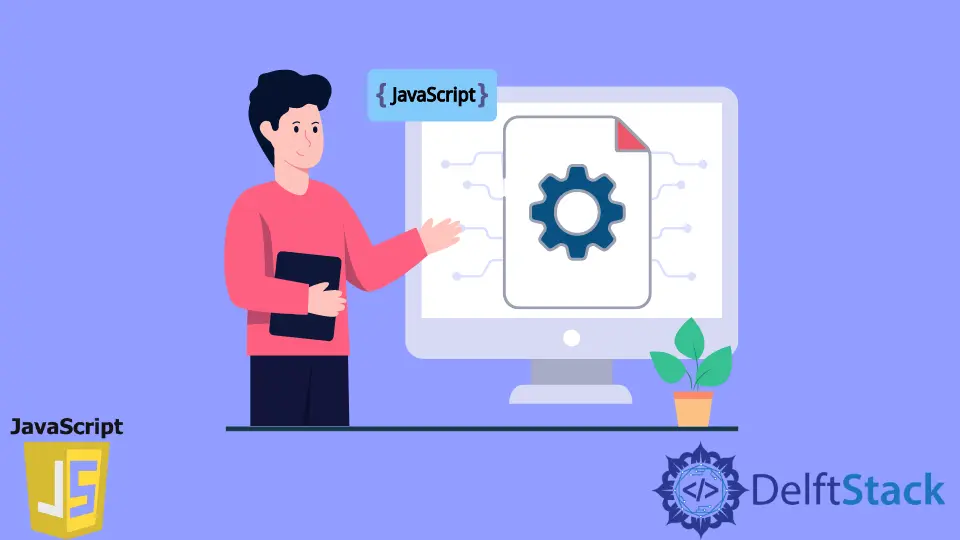
A file consists of multiple categorized data types similar to a blob
. And again, a file can have a specific type of documents.
We can store blob
, string
, array
, and many more in a file. Usually, all these items are defined within an object and later packed as files when instantiating one.
Here we will do something similar, and how this package, aka file’s detail, can be accessed and displayed will be demonstrated. The prior task is to generate an object we want to take as a file instantiation.
Later, we will use some methods to figure out the file’s type, name, and size. We will also use a FileReader()
to access our file stack details.
Instantiate a File for Variety Data Types in JavaScript
The W3C File API specification depicts a file constructor with a minimum of 2 parameters (and the 3rd can be optional). So, the arguments are the data or object in the form of an array, the file name we want to select, and lastly, the file type and modification date are added in an object format.
This example will have fileDetail
, the data pile with two strings and two array specifications. Then we will instantiate the File
constructor and manipulate the DOM to display the file’s size, type, name.
Let’s hop on to the code block.
Code Snippet:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Test</title>
</head>
<body>
</body>
</html>
const fileDetail = [
'It\'s a simple string and each character will be countded in the size',
'Second String',
new Uint8Array([10]),
new Uint32Array([2]),
];
const file = new File(
[fileDetail], 'file.txt',
{lastModified: new Date(2022, 0, 5), type: 'text/string and array'});
const fr = new FileReader();
fr.onload = (evt) => {
document.body.innerHTML = `
<p>file name: ${file.name}</p>
<p>file size: ${file.size}</p>
<p>file type: ${file.type}</p>
<p>file last modified: ${new Date(file.lastModified)}</p> `
} fr.readAsText(file);
Output:
As you can see, the fileDetail
object holds two strings, and each of the characters will occupy a 1-byte space in the memory.
When we have generated the array of 8-bits which will also occupy 1-byte, but in the case of 32-bits array, it will take 4-bytes of spaces. After instantiating the File constructor
, an instance will be created with the name file
.
The next step takes another instance for FileReader
and the onload method
manipulates the DOM
to preview the details. Ultimately, we are getting the file name, the size of the elements inside the file, the type, and the modification date.
Even if you do not use an array format in the first file constructor parameter, it will still work fine.
Take an Image for File Instantiation in JavaScript
Here, we will take an image as our fil element and check its type. In this case, the size of the file will be the characters of the images’ names.
Later we will display the data regarding the file.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
<img src="orange.jpg">
<p id="size"></p>
<p id="type"></p>
<p id="name"></p>
</body>
</html>
var file = new File(['orange'], 'orange.jpg', {type: 'image/jpg'});
document.getElementById('size').innerHTML = 'File size: ' + file.size;
document.getElementById('type').innerHTML = 'File type: ' + file.type;
document.getElementById('name').innerHTML = 'File name: ' + file.name;
Output: