How to Open Local Text File Using JavaScript
-
Use JavaScript
FileReader()
to Open Local Text File -
Use JavaScript
FileReader()
andjQuery
to Open Local Text File -
Use JavaScript
Promise
andFileReader
to Open Local Text File
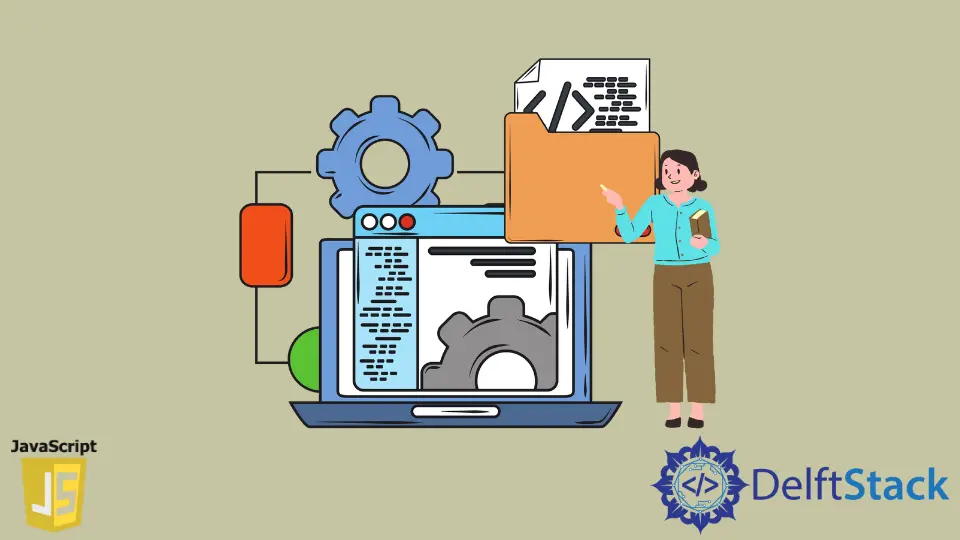
Our objective is to guide you about various techniques and methods that you can use to open a local text file using JavaScript. It also demonstrates the use of FileReader()
, Promise
, and different jQuery functions and events to read a text file from your system.
Use JavaScript FileReader()
to Open Local Text File
HTML Code:
<!DOCTYPE html>
<html>
<head>
<title>Read Text File</title>
</head>
<body>
<input id='selectedfile' type='text' name='selectedfile'>
<input id='inputfile' type='file' name='inputfile' onChange='showSelectedFile()'>
<br><br>
<pre id="output"></pre>
</body>
</html>
CSS Code:
input[type=file]{
width:90px;
color:transparent;
}
JavaScript Code:
function showSelectedFile() {
selectedfile.value = document.getElementById('inputfile').value;
}
document.getElementById('inputfile').addEventListener('change', function() {
var fr = new FileReader();
fr.onload = function() {
document.getElementById('output').textContent = fr.result;
} fr.readAsText(this.files[0]);
})
Output:
This code prints the content of the selected file (input file) the same as it is written in the input file.
The showSelectedFile()
method shows the input file’s path. Then, the element whose id’s value is inputfile
gets selected.
The event listener listens to the input
for changes. Here, it is changed when file(s) are chosen.
As soon as it is changed, an anonymous function runs that creates a FileReader
object using the FileReader()
constructor and names it fr
. Using fr
, we can access different functions and attributes of FileReader()
. This function reads the text content and displays it in the browser.
The .onload
is used to launch a particular function. In our case, this function selects the first element having id as output
and sets its .textContent
property with the value of .result
.
.textContent
reads the content of current element (node) and its descendant elements. .readAsText()
reads the content of the given input file. Once it reads all the content, the result
attribute has this content as a string.
The FileList is returned by the input
element’s files
property. In this example, it is assumed that this
is an input
element that returns the file object at index zero (this.files[0]
).
Use JavaScript FileReader()
and jQuery
to Open Local Text File
HTML Code:
<!DOCTYPE html>
<html>
<head>
<title>Read Text File</title>
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
</head>
<body>
<input type="file" name="inputfile" id="inputfile">
<br><br>
<pre id="output"></pre>
</body>
</html>
JavaScript Code:
$(document).ready(function() {
$('#inputfile').change(function() {
var file = this.files[0];
var fr = new FileReader();
fr.onload = function(data) {
$('#output').html(data.target.result);
} fr.readAsText(file);
});
});
Output:
Hey,
Are you learning about how to open a local text file using JavaScript?
This sample code also prints the content for the input file as it is written in the file (input file).
The ready()
event triggers when the document object model (DOM) is fully loaded. To understand easily, you can say that it makes a method accessible after loading the document.
The change()
event is used to know if the value of the element is changed. We can use change()
event only with input
, textarea
, and select
elements. It can either triggers the change()
event or executes a function on change()
event.
.html()
is very useful if you want to return or set the innerHTML
(content) of the chosen elements.
Now, you might think about how the innerHTML
is being changed? It is due to targeting the result
attribute of FileReader
.
Have a look at the following screenshot (outlined in orange color).
The .onload
, .result
, .readAsText()
are already explained in previous section. You can go there to have a look or check this link to read in detail.
You can use File-System to read a local text file (if you are coding on Node Environment).
Use JavaScript Promise
and FileReader
to Open Local Text File
HTML Code:
<!DOCTYPE html>
<html>
<head>
<title>Read Text File</title>
</head>
<body>
<input type="file" onchange="readInputFile(this)"/>
<h3>File Content:</h3>
<pre id="output"></pre>
</body>
</html>
JavaScript Code:
function readFile(file) {
return new Promise((resolve, reject) => {
let freader = new FileReader();
freader.onload = x => resolve(freader.result);
freader.readAsText(file);
})
}
async function readInputFile(input) {
output.innerText = await readFile(input.files[0]);
}
Output:
Hey,
Are you learning about how to open a local text file using JavaScript?
In this example code, the function readInputFile()
runs as soon as the input file is selected. This function will wait (using the await
operator) for the Promise
to be fulfilled and return the expected value (if resolved).
Further, the content (innerHTML
) of the element having id output
will be replaced with the value returned by Promise
. The await
operator is used to waiting for the Promise
, it is an object that represents pass or fails of an asynchronous function and its respective value. It has three states named resolve
, reject
, and pending
.