How to Write Data to a File in JavaScript
- Store Data on the Client-Side using the HTML 5 Web Storage API
- Delete Web Storage Data for Local Storage
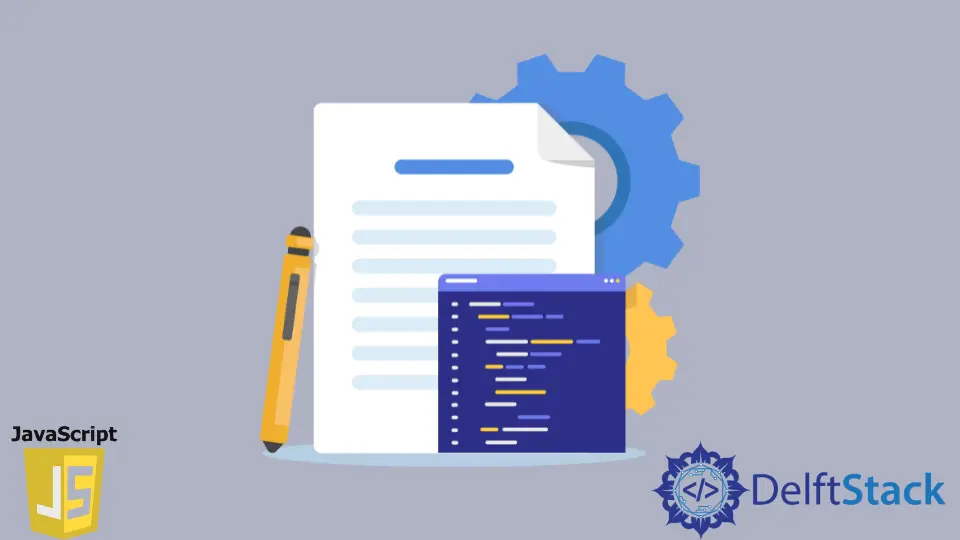
The HTML5 web storage API allows us to store data locally on the client-side. Note that only a small amount of data can be stored locally on the client-side, and its maximum size should be less than or equal to 5MB.
The data is not forwarded or sent back to the server at any given point in time if you use the web storage API. It will always be available locally inside a file.
Store Data on the Client-Side using the HTML 5 Web Storage API
Generally, there are two types of HTML web storage objects for storing data on the client-side.
- Local Storage (
window.localStorage
)
In Local Storage, the data will always be available at any time, even after the browser window is closed. This type is not applicable when the user views the webpage in incognito mode (private browsing). In this case, the data will be cleared soon after the last private window is closed.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="company_name"></div>
<script>
localStorage.setItem('company', 'Google');
const storedValue = localStorage.getItem('company');
let company_name = document.getElementById("company_name");
company_name.innerHTML = storedValue;
</script>
</body>
</html>
First, we have an empty div
element with an id
of company_name
. Inside this div
element, we will display the value, which we will store inside the local storage.
To insert any value inside the localStorage
, we have to pass the value in the form of key-value pair. You can do this process using the setItem
method, provided by the local storage. In this case, company
is the key, and Google
is the value. The setItem
method will not return anything; it will just store the value inside the local storage.
To get the value back, the local storage provides us with the getItem()
method that takes the key
of the value you want to get as an argument. Then, we store the results in the variable called storedValue
. At this point, we have the value Google
inside the storedValue
, and the only thing we have to do is display this value inside the div
tag.
To do this, we first have to get the div
element with the help of its id
attribute by using the document.getElementById
method and store that HTML element inside a new variable called company_name
.
Finally, we will add the value present inside the storedValue
variable to the div
element with the help of the innerHTML
property as company_name.innerHTML = storedValue
.
- Session Storage (
window.sessionStorage
)
Whenever you use the session storage for storing the data, the data will be available until the browser tab is open, and it will be cleared when the page session ends.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="company_name"></div>
<script>
sessionStorage.setItem('company', 'Google');
const storedValue = sessionStorage.getItem('company');
let company_name = document.getElementById("company_name");
company_name.innerHTML = storedValue;
</script>
</body>
</html>
Here, the code is entirely similar to that of Local Storage
. The only difference is that in place of localStorage
, we have used sessionStorage
. Firstly, the value Google
will be stored inside the sessionStorage
. This value can be accessed later simply by passing the key
associated with the value to the getItem()
method. Then, we store this value inside the storedValue
variable. In the end, we are adding this value to div
, which will display the value on the screen.
All modern browsers support both of these types.
Delete Web Storage Data for Local Storage
As we have already seen, the session storage deletes all the data as soon as the browser tab or the entire browser is closed. But what if you also want to delete the local data for security reasons or personal needs? There’s also a way to do this.
Here, there are two cases:
- If you want to delete a particular value from the local storage, you can use the method below. In the
remove
method, we have to pass thekey
for the value you want to delete.localStorage.remove('key')
- If you want to clear the entire local storage, you can do so with this method.
localStorage.clear()
Both of these methods will execute when the browser tab or the browser itself is closed.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn