How to Map Files in JavaScript
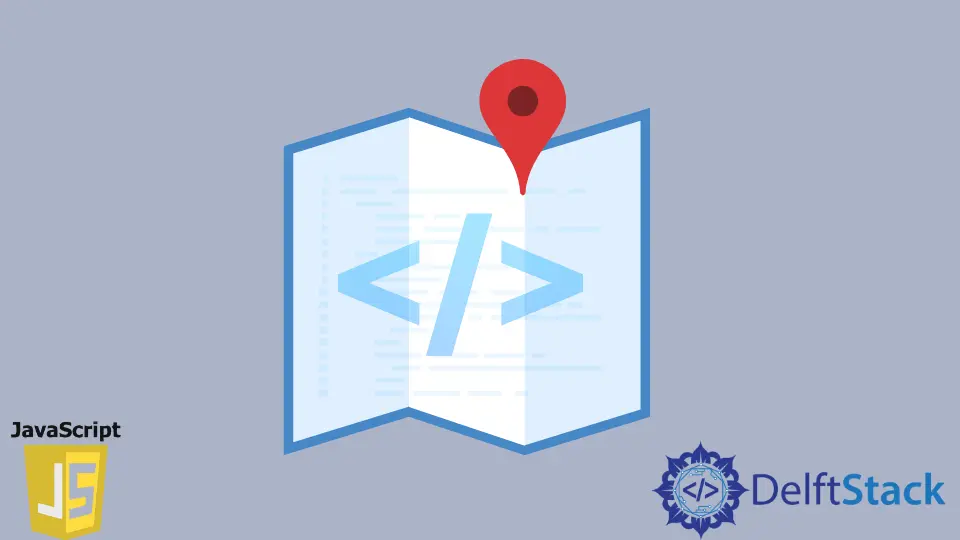
When developing in Angular or similar web frameworks, we use JavaScript files scripted as a developer’s version choice.
Lately, the ES6 and its successors have been a convenient form of scripting in JavaScript, and these modified versions require fewer code lines to define the same function from the prior versions of ES6.
All browsers are not compatible with the recent updates of ECMA Script, making the developing experience unbeatable the .map file
is introduced.
We usually use the minified by uglifying
files of our JavaScript files for browsers for production cases.
The minified files are inherently congested, with reduced white spaces, and more specifically, a compressed format of the functional js
files.
As we stated that the original file and the minified file have a difference in the file dimension and arrangement of the contents, the source map file creates a proper mapping for each line according to the function scopes.
The mapping convention is returned as row and column. Also, if you are coding in ES6 and making it readable by all browsers, the source map file will convert the original file to the preferable coding format.
This is technically how a source map file works, and here we will see how you can generate this map file locally and how your original file will look after conversion.
Install the babel
or webpack
to Generate Our Source Map File in JavaScript
In JavaScript, to enable a source map file, we need to install the babel
or webpack
. We will use babel here to generate our source map file.
Firstly, we will create an index.html
that will import the example.js
file. Our examplpe.js
will have a function that sums up some numbers, and the code lines are in the ES6 convention.
Code Snippet:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script src="example.js"></script>
</body>
</html>
var add = (...arr) => {
return arr.reduce((sum, el) => {
return sum + el;
}, 0)
} console.log(add(1, 2, 3));
Output:
We will keep both of the files index.html
and example.js
in a folder (let’s supposedly name it to work
).
We will open the cmd
and install babel locally in this’ work’ directory. The command is -
npm install @babel/cli @babel/core @babel/preset-env
After tapping the enter, you will get a package.json
file, package-lock.json
file, and a node_modules
folder.
We will require another file, .babelrc
, which will have the following lines for this task.
// The file name would be ".babelrc" in the work directory
{
"presets": [
"@babel/preset-env"
]
}
The next job is to add an npm
script in your package.json
file. There will be some dependencies
already allocated in your package.json
file.
These are added by default when you install the babel
packages in the first place. So the additional npm
scripts will look like this:
{
"dependencies": {
"@babel/cli": "^7.16.8",
"@babel/core": "^7.16.12",
"@babel/preset-env": "^7.16.11"
},
"scripts": {
"start-babel": "babel example.js --out-file main.dist.js --source-maps"
}
}
Soon after adding this portion in your package.json
file, go to the bash
and type up the following command.
npm run start-babel
After this, you will have a main.dist.js
file and a source file named the main.dist.js.map
file. The main.dist.js
file will have an explicit form of the example.js
file.
The terminating line of this file will have a commented part that will say *//# sourceMappingURL=main.dist.js.map*
. This ensures the procedure of mapping is successfully operated.
// main.dist.js
'use strict';
var add = function add() {
for (var _len = arguments.length, arr = new Array(_len), _key = 0;
_key < _len; _key++) {
arr[_key] = arguments[_key];
}
return arr.reduce(function(sum, el) {
return sum + el;
}, 0);
};
console.log(add(1, 2, 3));
// # sourceMappingURL=main.dist.js.map
// main.dist.js.map
{"version":3,"sources":["example.js"],"names":[],"mappings":";;AAAA,IAAI,GAAG,GAAG,SAAN,GAAM,GAAY;AAAA,oCAAR,GAAQ;AAAR,IAAA,GAAQ;AAAA;;AAClB,SAAO,GAAG,CAAC,MAAJ,CAAW,UAAC,GAAD,EAAM,EAAN,EAAY;AAC1B,WAAO,GAAG,GAAC,EAAX;AACH,GAFM,EAEJ,CAFI,CAAP;AAGH,CAJD;;AAKA,OAAO,CAAC,GAAR,CAAY,GAAG,CAAC,CAAD,EAAG,CAAH,EAAK,CAAL,CAAf","file":"main.dist.js","sourcesContent":["var add = (...arr) => {\r\n return arr.reduce((sum, el) =>{\r\n return sum+el;\r\n }, 0)\r\n}\r\nconsole.log(add(1,2,3));"]}
So, after all this task, our mission was to check if the original file example.js
is similar to the transpired file main.dist.js
file.
We will change the script directory of the html
file from example.js
to the main.dist.js
file. And our output will remain the same as before.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script src="main.dist.js"></script>
</body>
</html>
Output:
Conclusion
JavaScript source map files give a breakthrough in debugging JavaScript and CSS files. Specifically transpiring to readable codes and mapping properly the minified and original files.
This is how a .map file
in JavaScript keeps the development segment error-free.