How to Use Array of JSON Objects in JavaScript
- Creating an Array of JSON Objects
- Accessing an Object From an Array of JSON Objects
- Iterating Over an Array of JSON Objects
- Adding an Object to the Array of JSON Objects
- Removing an Object From a JSON Objects Array
- Search for an Element From the Array of JSON Objects
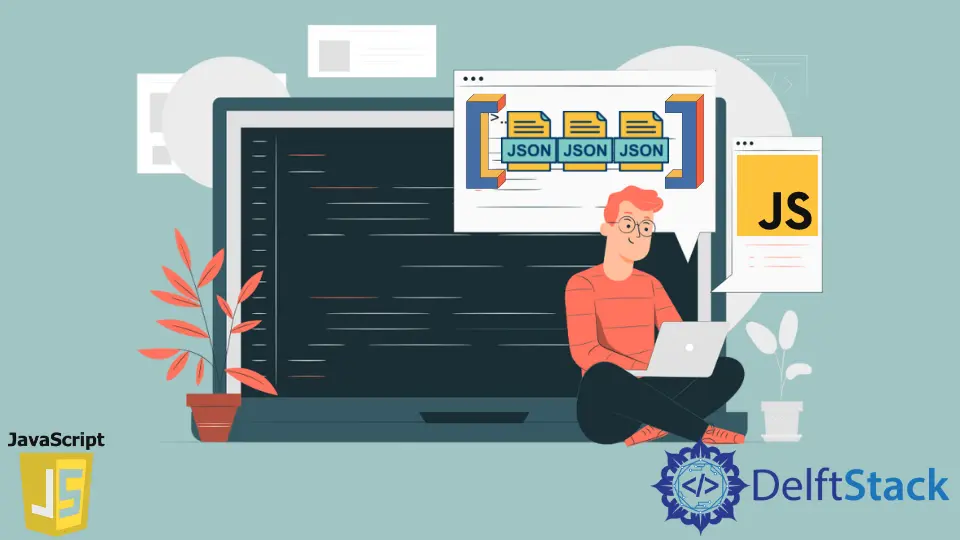
A JSON object is a simple JavaScript object. We can create an array with many similar JSON objects. Unlike the languages like C, C++, Java, etc., in javascript, it is easy to handle JSON objects array. It is comparable to a structure Array in C or an array of a class object in Java. In this article, we will be discussing how to create JSON objects array right through iterating and finding an element in it.
Creating an Array of JSON Objects
We can create an array of JSON object either by assigning a JSON array to a variable or by dynamically adding values in an object array using the .push()
operator or add an object at an index of the array using looping constructs like the for
loop or while
loop. Refer to the following code to understand better.
var months = [{'id': 1, 'name': 'January'}, {'id': 2, 'name': 'February'}];
console.log(JSON.stringify(months));
var monthNames = ['January', 'February'];
var month = {};
var monthsArray = [];
for (let i = 0; i < 2; i++) {
month.id = (i + 1);
month.name = monthNames[i];
monthsArray.push({...month});
}
console.log(JSON.stringify(monthsArray));
Output:
[{"id":1,"name":"January"},{"id":2,"name":"February"}]
[{"id":1,"name":"January"},{"id":2,"name":"February"}]
In the above code, the months
variable holds the array of JSON objects assigned explicitly, whereas the monthsArray
is an array of JSON objects created by assigning values inside a for
loop. We use the .push()
array function to add the generated javascript object to the end of the monthsArray
. Note that both the arrays display the same structure when logged with the JavaScript console.log()
statement. The JSON.stringify()
function converts the JSON array to a string format to make it in a human-readable format.
Accessing an Object From an Array of JSON Objects
We have created a JSON objects array. Let us see how we can access an element of this array. The approach is the same like we do for a simple string array or a number array. We can access an array object using the array Index (which starts from 0
) and manipulate the object value at that index. Refer to the following code.
var months = [{'id': 1, 'name': 'January'}, {'id': 2, 'name': 'February'}];
console.log(months[0].id);
console.log(months[0].name);
months[0].id = 3;
months[0].name = 'March';
console.log(months[0].id);
console.log(months[0].name);
Output:
1
January
3
March
In the code, we changed the value of object parameters by accessing the first index of the months
array using the array Index
. Similarly, we can change the value of any other object of the array using its index.
Iterating Over an Array of JSON Objects
We can iterate the objects array the same way we do for a string array or a number array. We can use a for
loop or a while
loop for our purpose. We can access the elements using the array indices. We iterate the array from the 0th index to the array length. The .length
attribute returns the length of the objects array too. Refer to the following code that shows iterating the array and print value of each object in it.
var months = [{'id': 1, 'name': 'January'}, {'id': 2, 'name': 'February'}];
for (let i = 0; i < months.length; i++) {
console.log(`${i} id:${months[i].id}, name:${months[i].name}`)
}
Output:
0 id:1, name:January
1 id:2, name:February
Adding an Object to the Array of JSON Objects
We have created an array of objects and iterated over them. Let us now look at how to add elements to the JSON array. We can use the .push()
function to add a JSON object to the end of the array. The .unshift()
function adds an element at the beginning of a JSON array. The .splice()
inserts an object at a specified index in an array. Refer to the following code that depicts the usage of each of these functions.
var months = [{'id': 1, 'name': 'January'}, {'id': 2, 'name': 'February'}];
months.push({'id': 4, 'name': 'April'});
console.log(JSON.stringify(months));
months.unshift({'id': 12, 'name': 'December'})
console.log(JSON.stringify(months));
months.splice(3, 0, {'id': 3, 'name': 'March'});
console.log(JSON.stringify(months));
Output:
[{"id":1,"name":"January"},{"id":2,"name":"February"},{"id":4,"name":"April"}]
[{"id":12,"name":"December"},{"id":1,"name":"January"},{"id":2,"name":"February"},{"id":4,"name":"April"}]
[{"id":12,"name":"December"},{"id":1,"name":"January"},{"id":2,"name":"February"},{"id":3,"name":"March"},{"id":4,"name":"April"}]
Note, we can use the .splice()
function to replace objects from an array with newer values passed as parameters. The first parameter of the .splice()
method is the index in which we perform the operations. As the second parameter, we mention the number of elements we wish to replace. The final parameter is the value that we insert into the array.
Removing an Object From a JSON Objects Array
We can remove elements from an object array in a fashion similar to that of simple JavaScript arrays. We can use the .pop()
method of javascript to remove the element from the end of the JSON objects array. The .shift()
removes an object from the beginning of the JSON objects array. The .splice()
function removes an element at a specified index of the JSON array. Refer to the following code.
var months = [
{'id': 12, 'name': 'December'}, {'id': 1, 'name': 'January'},
{'id': 2, 'name': 'February'}, {'id': 3, 'name': 'March'},
{'id': 4, 'name': 'April'}
];
months.shift();
console.log(JSON.stringify(months));
months.pop();
console.log(JSON.stringify(months));
months.splice(1, 1);
console.log(JSON.stringify(months));
Output:
[{"id":1,"name":"January"},{"id":2,"name":"February"},{"id":3,"name":"March"},{"id":4,"name":"April"}]
[{"id":1,"name":"January"},{"id":2,"name":"February"},{"id":3,"name":"March"}]
[{"id":1,"name":"January"},{"id":3,"name":"March"}]
Search for an Element From the Array of JSON Objects
We can use .indexOf()
or includes()
to check if an element is present in a simple string array. But these methods will not work for an array of objects. We can use certain similar functions like .filter()
, .find()
, findIndex()
to check if an element is present in the array. We can manually iterate the array and check if the element exists using looping constructs (like for
, while
loops etc.), but that may be the last option to be considered, as it will lead to bulky code. The javascript inbuilt functions like .filter()
, .find()
, findIndex()
are useful for searching objects in a JSON objects array. The .filter()
function returns an array containing the objects that satisfy a certain condition, .find()
returns the object that satisfies the condition passed as an inline function to it, .findIndex()
will return the index of the object if it can find it in the array, else it returns -1
. Hence, by knowing the return type of the functions, we can design the search functionality in an array of JSON objects. Refer to the following code to understand the usages of .filter()
, .find()
, findIndex()
better.
var months = [
{'id': 12, 'name': 'December'}, {'id': 1, 'name': 'January'},
{'id': 2, 'name': 'February'}, {'id': 3, 'name': 'March'},
{'id': 4, 'name': 'April'}
];
(months.filter(i => i.name === 'April').length) ?
console.log('April month found') :
console.log('Not found');
(months.find(i => i.name === 'January') != {}) ?
console.log('January month found') :
console.log('Not found');
(months.findIndex(i => i.name === 'April') > -1) ?
console.log('April month found') :
console.log('Not found');
Output:
April month found
January month found
April month found