JavaScript String.indexOf() Method
-
Syntax of JavaScript
string.indexOf()
: -
Example 1: Convert a UTF-16 Unicode Into Character Using the
string.indexOf()
Method -
Example 2: JavaScript
string.indexOf()
Method Is Case Sensitive -
Example 3: Find the Index of a Word’s Second Occurrence in a String Using the
start_index
Parameter in thestring.indexOf()
Method -
Example 4: Find the Number of Characters Using the
start_index
Parameter in thestring.indexOf()
Method
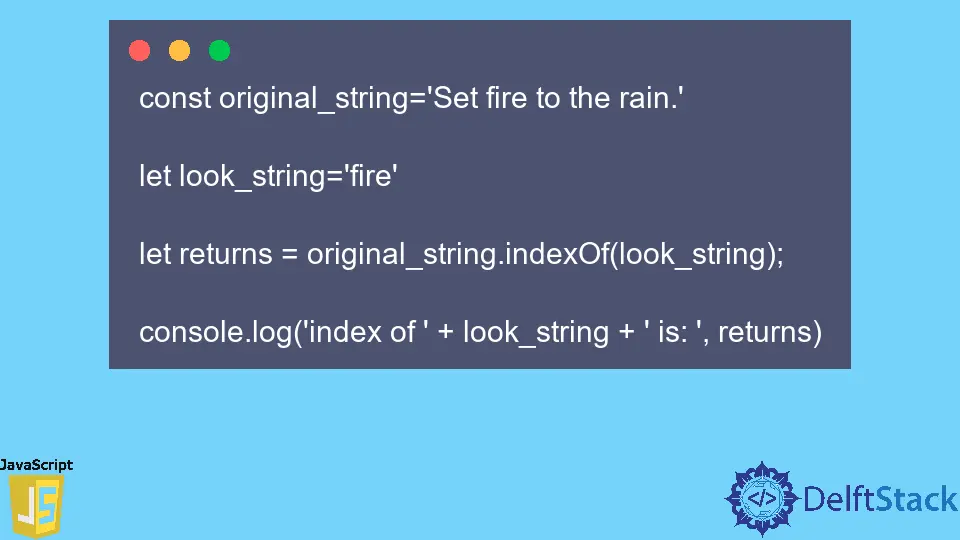
The string.indexOf()
method starts its search of a string from the specified index in the original string and returns the index of the first occurrence of that string in the original string.
Syntax of JavaScript string.indexOf()
:
original_string.indexOf(look_string)
original_string.indexOf(look_string, start_index);
Parameters
look_string |
A string to look for in the original_string starting from the index greater than or equal to the default position. If not provided, its value is considered undefined by default. |
start_index (optional) |
An integer representing the start index of the search; its default value is 0. If start_index is greater than the length of original_string , the method does not look for the string, and if start_index has a negative value, the method considers its value as 0. |
Return
The string.indexOf()
method returns an integer value indicating the index at the first occurrence of look_string
and -1
if look_string
does not occur in the original_string
.
-1
is returned when the look_string
is not present within the start_index
parameter to the length of the original_string
. -1
is also returned when the start_index
is greater than the length of the original_string
.
Example 1: Convert a UTF-16 Unicode Into Character Using the string.indexOf()
Method
Let’s start from a simple example that prints the starting index of the characters in a string in the original_string
calling the JavaScript string.indexOf()
method.
const original_string='Set fire to the rain.'
let look_string='fire'
let returns = original_string.indexOf(look_string);
console.log('index of ' + look_string + ' is: ', returns)
Output:
index of fire is: 4
Example 2: JavaScript string.indexOf()
Method Is Case Sensitive
The below example demonstrates the JavaScript string.indexOf()
method is case sensitive.
const original_string = 'First name';
console.log(original_string.indexOf('first'))
console.log(original_string.indexOf('First'));
Output:
-1
0
Example 3: Find the Index of a Word’s Second Occurrence in a String Using the start_index
Parameter in the string.indexOf()
Method
The string.indexOf()
method is used in this example to find the appearance of a word a second time in the string object original_string
.
const original_string='you either win or you lose.';
const look_string= 'you';
let index_of_first= original_string.indexOf(look_string); //0
index_of_second = original_string.indexOf(look_string, (index_of_first + 1));
console.log(`The index of the second occurrence of the word "${look_string}" is`, index_of_second);
Output:
The index of the second occurrence of the word "you" is 18
Example 4: Find the Number of Characters Using the start_index
Parameter in the string.indexOf()
Method
This example will find the occurrences of a sequence of characters given in the look_string
parameter to the JavaScript string.indexOf()
method and print it on the console.
It will also print the indexes at which it finds the sequence of characters in the original_string
.
const original_string = "It is for forever";
const look_string='for';
let no_of_occurrences = 0;
let start_index = original_string.indexOf(look_string);
console.log('occurs at index = ', start_index);
while (start_index !== -1) {
no_of_occurrences++;
start_index = original_string.indexOf(look_string, start_index + 1);
console.log('occurs at index = ', start_index);
}
console.log(`The string '${look_string}' occurs ${no_of_occurrences} times in "${original_string}".`); // 2
Output:
occurs at index = 6
occurs at index = 10
occurs at index = -1
The string 'for' occurs 2 times in "It is for forever".
The word for
appeared for the first time in the original string at index 6, and the word forever
appeared for the second time at index 10. At last, the indexOf()
method returns -1
because it did not find any other occurrences of for
after index 10.