JavaScript String.replace() Method
-
Syntax of JavaScript
string.replace()
: -
Example Codes: Use the
string.replace()
Method to Replace a String -
Example Codes: Use the
string.replace()
Method to Replace a String With a Regular Expression -
Example Codes: Use the
string.replace()
Method to Replace a String With a Regular Expression Case Sensitivity
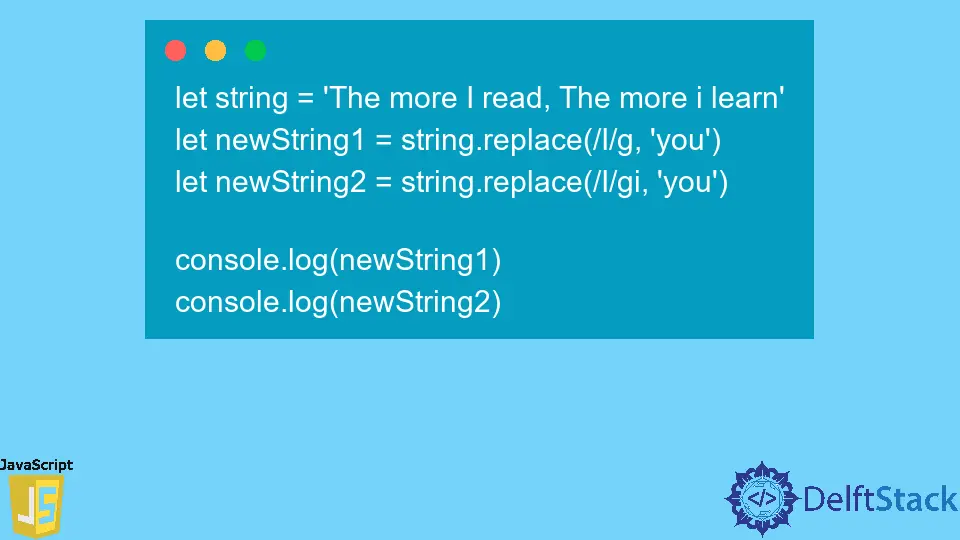
In JavaScript, the string.replace() method returns a new string. It first searches the given value from a string and replaces it with the new value.
A value, substring, or regular expression can be used as searches.
Syntax of JavaScript string.replace()
:
let newString = string.replace(searchValue, newValue)
Parameters
searchValue (required) |
A value that will be replaced. It can be a substring or regular expression. |
newValue (required) |
A value that will take the position of the search value. |
Return
The string.replace()
method returns a new string where the new value replaces the search value.
Important: The string.replace()
method will return the result. That means it does not change the actual string; you must declare a new variable to store the result.
Example Codes: Use the string.replace()
Method to Replace a String
let string = 'The more I read, The more I learn'
let newString = string.replace('I', 'you')
console.log(newString)
Output:
The more you read, The more I learn
We passed the value I
as the search value and you
as the new value. That means I
will replace the string with you
.
This method replaced the first portion of the string but did not replace it globally. We must use the regular expression to replace a value from the string globally.
Example Codes: Use the string.replace()
Method to Replace a String With a Regular Expression
let string = 'The more I read, The more I learn'
let newString = string.replace(/I/g, 'you')
console.log(newString)
Output:
The more you read, The more you learn
We wrapped I
with a regular expression and used the flag -g
to represent the global. As a result, the value I
is replaced everywhere in the string with the value you
.
Example Codes: Use the string.replace()
Method to Replace a String With a Regular Expression Case Sensitivity
let string = 'The more I read, The more i learn'
let newString1 = string.replace(/I/g, 'you')
let newString2 = string.replace(/I/gi, 'you')
console.log(newString1)
console.log(newString2)
Output:
The more you read, The more i learn
The more you read, The more you learn
First, we provided the flag -g
to replace the string globally. But it did not work because of case sensitivity.
Second, we again provided the flag -g
to replace the string globally, but this time we provided another flag -i
to make it case insensitive. As a result, this method replaced all the values.
Niaz is a professional full-stack developer as well as a thinker, problem-solver, and writer. He loves to share his experience with his writings.
LinkedIn