JavaScript String.fromChar() Method
-
Syntax of JavaScript
String.fromCharCode()
: -
Example Codes: Convert the UTF-16 Unicode Value to a Character Using JavaScript
String.fromCharCode()
-
Example Codes: Convert Multiple UTF-16 Code Units Into a Single String Using JavaScript
String.fromCharCode()
-
Example Codes: Behavior of the JavaScript
String.fromCharCode()
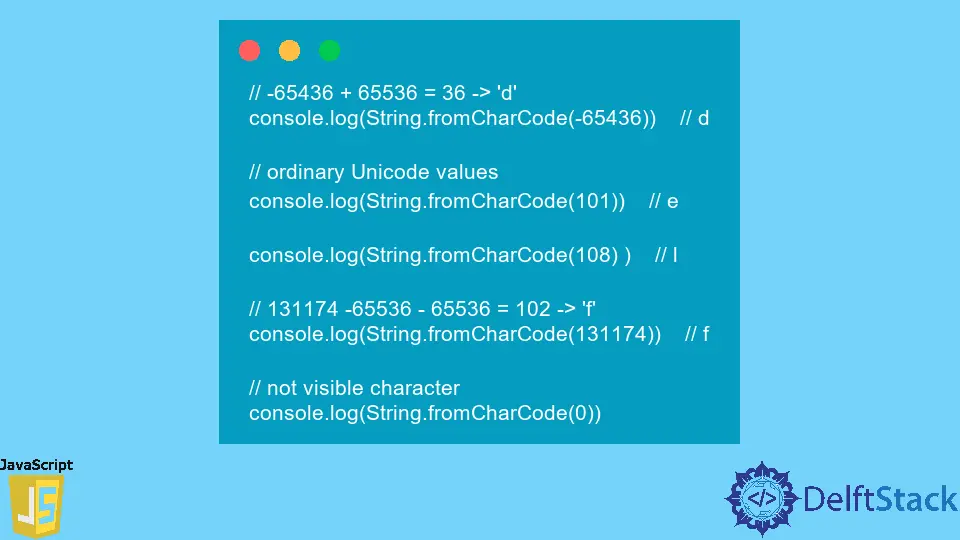
The JavaScript String.fromCharCode()
converts the UTF-16 Unicode values into characters and combines them in a string in the order they were provided in parameters. The String.fromCharCode()
method is a static method of the String.
Therefore, this method does not need a calling object. Instead, the method gets invoked through the string constructor object String
.
Syntax of JavaScript String.fromCharCode()
:
String.fromCharCode(code_1);
String.fromCharCode(code1, code2, ... , codeN)
Parameters
code_1 |
A numerical value representing the UTF-16 Unicode value in the range of 0 to 65535. This is the Code unit that is converted into character. |
code1,code2,...,codeN |
A series of UTF-16 Unicode values to convert into characters combined in a string. N is the number of parameters. |
Return
The JavaScript String.fromCharCode()
method returns a string rather than a string object.
Example Codes: Convert the UTF-16 Unicode Value to a Character Using JavaScript String.fromCharCode()
The code_1
parameter can be provided in decimal, hexadecimal, or any other number system as long as it represents the UTF-16 Unicode value.
const code_1= 68 //decimal value
const code_2 = '0x44' //hexadecimal value
console.log(`Converted ${code_1} to `,String.fromCharCode(code_1));
console.log(`Converted ${code_2} to `,String.fromCharCode(code_2));
No calling object was created here to call the static function but only used it as String.fromCharCode()
.
Output:
Converted 68 to D
Converted 0x44 to D
Above, the String.fromCharCode()
took the UTF-16 code as parameter and converted into character. The returned string is printed on the screen.
Example Codes: Convert Multiple UTF-16 Code Units Into a Single String Using JavaScript String.fromCharCode()
In this example, a series of Unicode values are converted into characters and combined in a single string.
console.log(String.fromCharCode(68,101,108,102,116,83,116,97,99,107))
Unicode values are passed into the function through the code1, code2,..., codeN
parameters. N
is the number of parameters, and its value is 10 in this example.
This example also demonstrates that String.fromCharCode()
is case-sensitive while converting the Unicode values into characters.
Output:
DelftStack
The console displays the returned string DelftStack
. The method converted and combined the Unicode values in the returned string in the order they were given in the parameters.
Example Codes: Behavior of the JavaScript String.fromCharCode()
This example shows the behavior of the JavaScript String.fromCharCode()
method when the numerical value given in the code_1
parameter is:
- Less than the valid range (
code_1
< 0). Then the method keeps adding 65536 until the value is within the valid range of 0 to 65536. - Greater than the valid range (
code_1
> 65536). Then the method keeps subtracting the number 65536 until the value is within the valid range(0 to 65536).
// -65436 + 65536 = 36 -> 'd'
console.log(String.fromCharCode(-65436)) // d
// ordinary Unicode values
console.log(String.fromCharCode(101)) // e
console.log(String.fromCharCode(108) ) // l
// 131174 -65536 - 65536 = 102 -> 'f'
console.log(String.fromCharCode(131174)) // f
// not visible character
console.log(String.fromCharCode(0))
Output:
d
e
l
f
�
The JavaScript String.fromCharCode()
prints a null character or a character that is not visible when it fails to convert the code_1
parameter into a character.