How to Fill an Array With Range in JavaScript
-
Fill an Array With Range Using the
Array.fill()
Function in JavaScript -
Fill an Array With Range Using the
Array.from()
Function in JavaScript - Fill an Array With Range Using the ES6 Spread Operator in JavaScript
-
Fill an Array With Range Using the
Array.map()
Function in JavaScript - More Ways to Fill an Array With Range in JavaScript
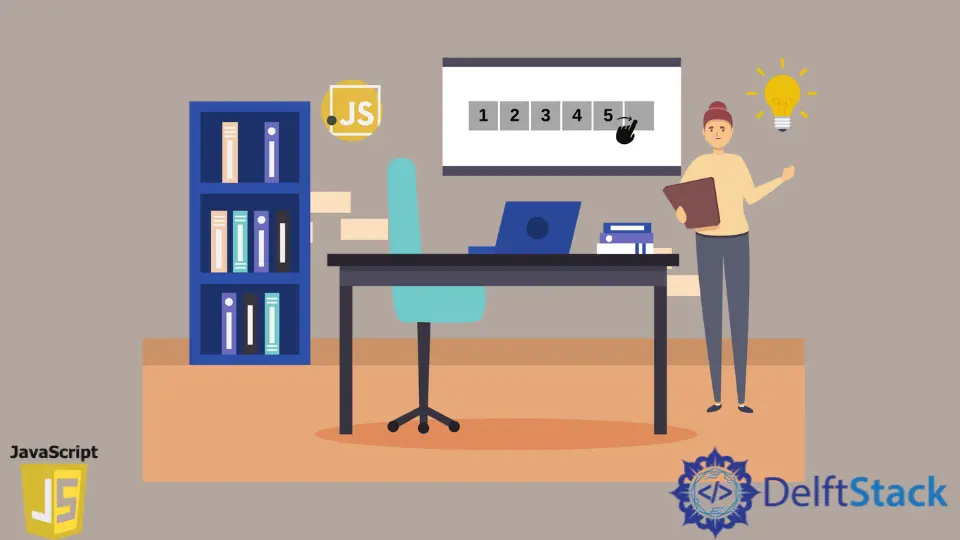
This article will discuss the various methods provided by the Array class that we can use to fill an array with a range in JavaScript.
Fill an Array With Range Using the Array.fill()
Function in JavaScript
The Array.fill()
method fills an existing array with a particular value.
This method takes three parameters: value
, which we will fill into the array, and start
and end
, which describe where we want to add the data inside the array. start
and end
are optional parameters.
Example:
var arr = [1, 2, 3, 4];
arry.fill(6);
console.log(arr);
Output:
[6, 6, 6, 6]
As we can see, all the elements inside the array are successfully replaced and filled with the value 6. If we don’t want to replace all the elements with that value, we can specify the start
and end
indexes.
Fill an Array With Range Using the Array.from()
Function in JavaScript
Introduced in the ES6 version of JavaScript, the Array.from
method creates a shallow-copied instance of an array from an array-like, iterable object.
Syntax:
Array.from(target, mapFunction, thisValue)
Where:
target
: The object to convert to an array.mapFunction
: Calls on every array element. This is an optional parameter.thisValue
: The value to use asthis
when executing themapFunction
. This is also an optional parameter.
To fill the array, we use Array.from()
with Array.keys()
. The Array.keys()
method extracts all the array’s keys and returns an iterator, then the Array.from()
function takes this iterator as a parameter.
In the end, all the indexes are copied as elements of the new array.
Example:
var arr = Array.from(Array(5).keys());
console.log(arr);
Output:
[ 0, 1, 2, 3, 4 ]
Array.from()
function is not supported on Internet Explorer 11 and earlier versions.Fill an Array With Range Using the ES6 Spread Operator in JavaScript
Instead of the Array.from()
function, we can also use a spread operator, represented by three dots ...
.
We will pass Array.keys()
, which will return an iterator and spread the elements present inside this iterator using the spread operator ...
, placed before Array.keys()
.
After that, we have to enclose all of this inside the square brackets []
to represent an array.
The spread operator will generate the same output as the Array.from
function.
Example:
var arr = [...Array(5).keys()];
console.log(arr);
Output:
[ 0, 1, 2, 3, 4 ]
Fill an Array With Range Using the Array.map()
Function in JavaScript
The Array.map()
function will create a new array by running a provided function as a parameter on every element of the calling array. This can take any function as a parameter, such as an arrow, callback, or inline.
Example:
var elements = 5;
var arr = [...Array(elements)].map((item, index) => index);
console.log(arr);
Output:
[ 0, 1, 2, 3, 4 ]
In the example above, the map
function takes the arrow function as a parameter to extract the item
and index
. This arrow function will be called on every element of the array.
In the end, the index value of all the array elements will be extracted and stored inside the new array.
More Ways to Fill an Array With Range in JavaScript
The methods we have discussed to fill an array with a range in JavaScript are widely used by many developers. Apart from these, there are other methods we can use.
- The
Array.prototype.apply()
, which is a pre-built method in JavaScript. - The
range()
method ofUnderscore.js
library.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedInRelated Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript