How to Convert a String Into a Number in JavaScript
-
Use the
Number()
Function to Convert a String to a Number in JavaScript -
Use the
parseInt()
Function to Convert a String to Integer in JavaScript -
Use the
Math
Object to Convert a String to Integer in JavaScript - Conclusion
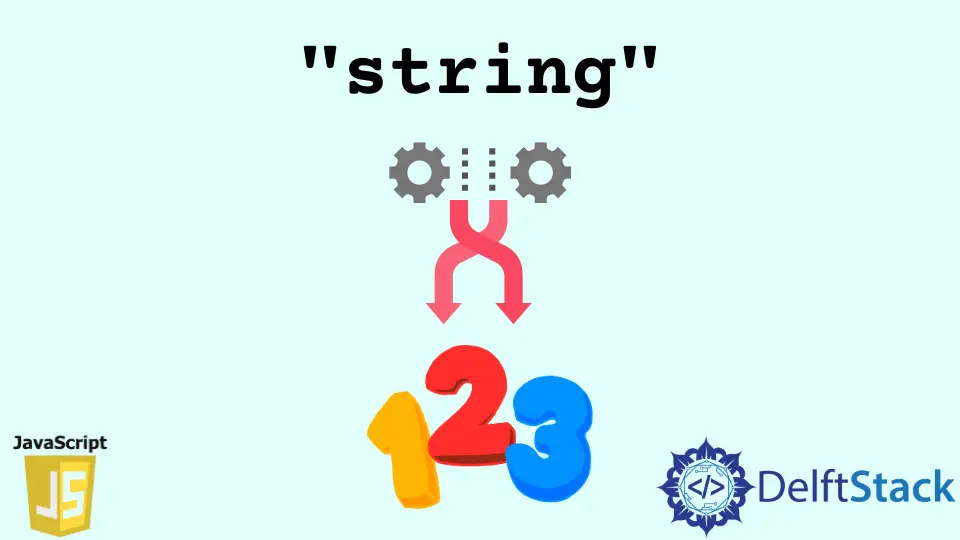
We must have had various scenarios to convert a number. For example, the data fetched from an API or a database query in a string format to a number. As strings are operated upon differently, it may be necessary to convert these strings to a number format to perform arithmetic operations on them with ease. Here are a few ways to do so:
Number("123")
parseInt("123")
Math.floor("123.12")
Math.round("123")
Use the Number()
Function to Convert a String to a Number in JavaScript
The Number()
is a function of the Number
construct which can be used to convert other data types to a number format (as per the MDN Docs). It returns NaN
if the input parameter is either undefined or not convertible to a number. For instance:
console.log(Number('246'))
console.log(Number('246.5'))
console.log(Number(undefined))
console.log(Number('Hello'))
Output:
246
246.5
NaN
NaN
Use the parseInt()
Function to Convert a String to Integer in JavaScript
Another way of dealing with string numbers is using the parseInt()
method of JavaScript. parseInt()
takes two arguments, one is the string that needs to be converted, and the other one is the radix
(means the base). Most numbers that we deal with in our day-to-day life are usually in the base of 10, which signifies a decimal value. In most cases, we don’t need to specify the radix as the default is 10.
console.log(parseInt('123'));
console.log(parseInt('abc'));
console.log(parseInt('-123'));
console.log(parseInt('100.50'));
Output:
123
NaN
-123
100
parseInt("100.50")
returns 100 instead of 100.50, as it converts the input number to an integer. Hence, while using parseInt()
be aware of the fact.
parseInt()
also can convert hexadecimal values and values with the different base number systems, like the binary system, the octal system, etc. For more information, check out the docs. Similarly, we can use parseFloat()
to convert a string to a floating-point number.
Use the Math
Object to Convert a String to Integer in JavaScript
Math
is a built-in object in JavaScript which has methods for complex mathematical operations. It works on the data type number. But few methods of this in-built object are used to convert the string to an integer. For example,
console.log(Math.ceil('123'));
console.log(Math.floor('300'));
console.log(Math.abs('450'));
Output:
123
300
450
However, there is a pitfall when using the Math
methods for conversion. For floating-point values, we cannot use them as they will convert those into an integer. Hence, we will lose the decimal part of the value.
Math.ceil("220.5")
: returns221
. It takes the ceiling value, approximating the decimal number to the next integer value that is221
. Hence, we cannot use it to get220.5
. In such scenarios useparseFloat()
.Math.floor("220.5")
: returns220
. As compared to theceil()
function,floor()
returns the previous integer value. HenceMath.floor()
would not work for floating point number values.Math.abs("450")
: Can be used as it will return450
but would not work out with negative integer values. For instance, the following difference will not serve our purpose when dealing with negative integer values. As per the following scenarios:
console.log(Maths.abs('240.64'))
console.log(Math.abs('-240.25'))
Output:
240.64 // The decimal value of .64 is captured here
240.25 // -240.25 is transformed to 240.25
Conclusion
Depending upon the value that we expect out of the string number, the best option will be to use the Number()
method. If you are playing with only integer values, we recommend using the parseInt()
. Make judicious use of the in-built Math object in JavaScript to convert a string number as it may be tricky based on the method that we use.
Related Article - JavaScript String
- How to Get Last Character of a String in JavaScript
- How to Convert a String Into a Date in JavaScript
- How to Get First Character From a String in JavaScript
- How to Convert Array to String in JavaScript
- How to Check String Equality in JavaScript
- How to Filter String in JavaScript