JavaScript Math.floor() Method
-
Syntax of JavaScript
Math.floor()
Method -
Example 1: Use the
Math.floor()
Method to Round Down Numbers -
Example 2: Use the
Math.floor()
Method on a String Value -
Example 3: Use the
Math.floor()
Method on Addition of Two Values -
Example 4: Use the
Math.floor()
Method on an Empty Value -
Example 5: Use
Math.floor()
andMath.round()
to Get and Compare the Rounded Numbers
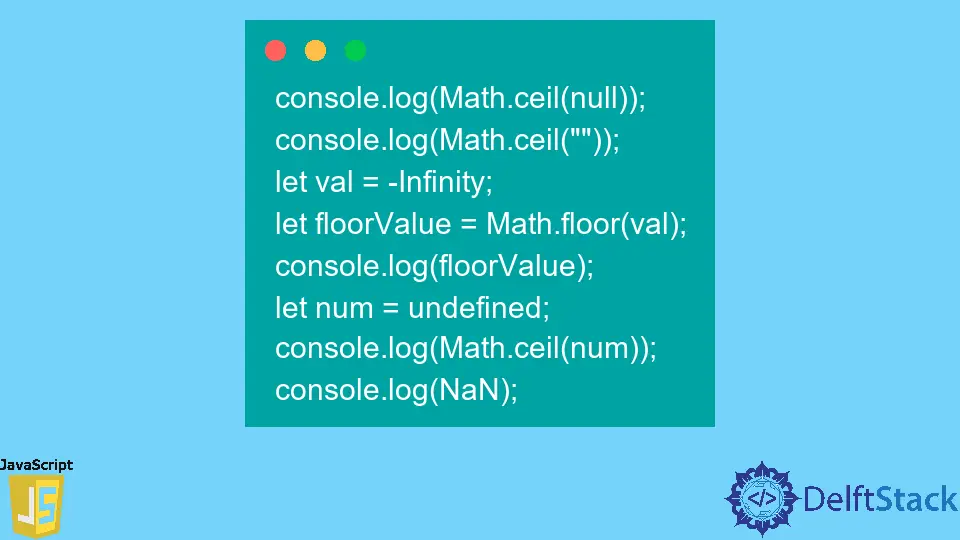
In JavaScript, Math.floor()
is a built-in library method used to round down the numbers to the nearest integer value. In simple terms, the Math.floor()
method removes the decimal part of the number and gives the integer part as an output.
Syntax of JavaScript Math.floor()
Method
Math.floor(x);
Parameters
x
- Any numeric value or expression.
Return
The Math.floor()
method returns the nearest largest integer smaller than or equal to x
.
Example 1: Use the Math.floor()
Method to Round Down Numbers
In the example below, we have taken 4 different numeric values to round down using the Math.floor()
method.
In the example output, users can observe that it returns the integer part of the numeric values. Even if 0.99 is nearest to 1, the method returns 0 as an output as we are rounding down the number.
let num = 1.1;
console.log(Math.floor(num));
console.log(Math.floor(4.51));
console.log(Math.floor(0.99));
console.log(Math.floor(10));
Output:
1
4
0
10
Example 2: Use the Math.floor()
Method on a String Value
In general, Math.floor()
is used with numeric values, but in some cases, if we use it with string values, it returns NaN
(Not a Number). Users can see the output for the different string values below.
let string = "Delft";
Math.ceil(string);
console.log(Math.floor(string));
console.log(Math.floor("Hello"));
Output:
NaN
NaN
Example 3: Use the Math.floor()
Method on Addition of Two Values
We can use the Math.floor()
method to round down numbers after performing different operations on multiple numbers.
In the example below, we will add two numbers and round them down using the Math.floor()
method. Also, we will round down both numbers separately first, add them, and observe the output.
let number1 = 3.2;
let number2 = 5.99;
let sum1 = Math.floor(number1+number2);
let sum2 = Math.floor(number1) + Math.floor(number2);
console.log(sum1);
console.log(sum2);
Output:
9
8
Example 4: Use the Math.floor()
Method on an Empty Value
Users can apply the Math.floor()
method to empty values. We will take the different types of empty values and observe the output of Math.floor()
using the below example.
In the output, users can see that for the null
and empty string ""
, the method always returns 0 instead of NaN
. When we pass the positive or negative Infinity
as a parameter of the Math.floor()
method, it always returns the same value.
For the undefined
, and NaN
values, Math.floor()
method returns NaN
(Not a Number) value.
console.log(Math.ceil(null));
console.log(Math.ceil(""));
let val = -Infinity;
let floorValue = Math.floor(val);
console.log(floorValue);
let num = undefined;
console.log(Math.ceil(num));
console.log(NaN);
Output:
0
0
-Infinity
NaN
NaN
Example 5: Use Math.floor()
and Math.round()
to Get and Compare the Rounded Numbers
In this example, we will use the Math.floor()
and Math.round()
methods to compare the output and see the difference between both.
In the example output, users can see that the Math.floor()
method always rounds down the number, but the Math.round()
method either rounds up or down the number according to the nearest integer value.
let number = 1.99;
console.log(Math.floor(number));
console.log(Math.round(number));
console.log(Math.floor(-1.49));
console.log(Math.round(-1.49));
Output:
1
2
-2
-1
The Math.floor()
method is supported in all browsers. This article has explored different use cases of the Math.floor()
method.