JavaScript Math.random() Method
-
Syntax of
Math.random()
-
Example 1: Use the
Math.random()
Method in JavaScript -
Example 2: Use the
Math.random()
Method in Real-World Scenario
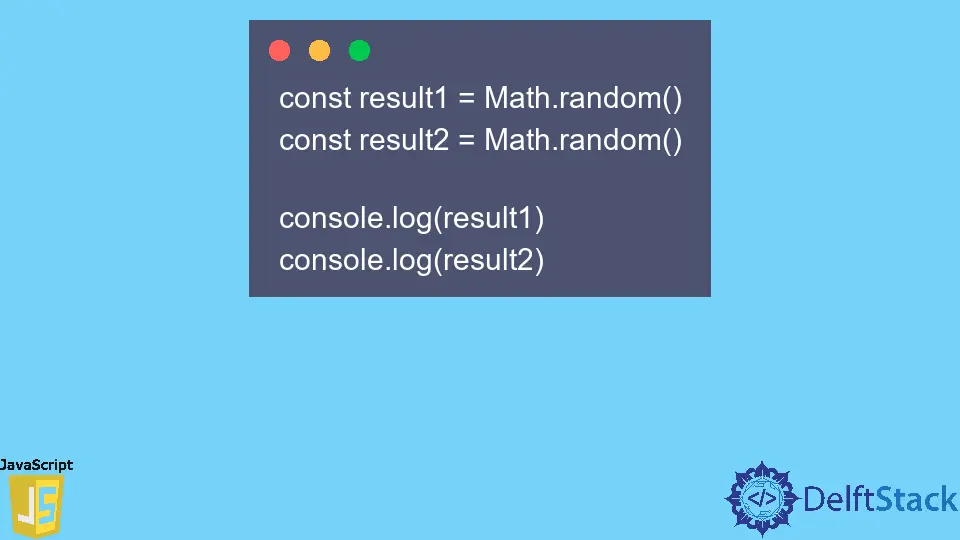
The Math.random()
method generates a random number in floating points between 0 and 1. Here, 0 is inclusive, and 1 is exclusive.
That means this method does not return a random number greater than 1.
Syntax of Math.random()
Math.random()
Parameters
This method doesn’t have any parameters.
Return
The Math.random()
method returns a floating point random number from 0 to 1 where 0 is inclusive, and 1 is exclusive.
Example 1: Use the Math.random()
Method in JavaScript
const result1 = Math.random()
const result2 = Math.random()
console.log(result1)
console.log(result2)
Output:
0.7238581808516886
0.9130175453865705
We use this method two times in the above program. This method returns two different floating point numbers in the range of 0 to 1.
It will return different numbers each time we call it; the length will be less than 1 and greater than 0.
Example 2: Use the Math.random()
Method in Real-World Scenario
function getRandomNum(min, max) {
return Math.floor(Math.random() * (max - min) ) + min
}
console.log(getRandomNum(5, 25))
console.log(getRandomNum(1, 100))
Output:
16
46
Let’s say we need to generate a program for the lottery that can generate any random number from a given range.
We create a function that accepts two numbers according to our requirement specification. We use Math.floor()
to round the floating numbers into integers.
Finally, use the Math.random()
method to generate a random number from the given ranges.
We tested our program two times with different ranges, and our program successfully generated random numbers with different ranges.
Niaz is a professional full-stack developer as well as a thinker, problem-solver, and writer. He loves to share his experience with his writings.
LinkedIn