JavaScript Math.PI Property
-
Syntax of
Math.PI
-
Example 1: Use
Math.PI
Property to Get Circumference of a Circle -
Example 2: Use
Math.PI
Property to Get Surface Area of a Circle -
Example 3:
TypeError
Shows WhenMath.PI
Is Used as a Function
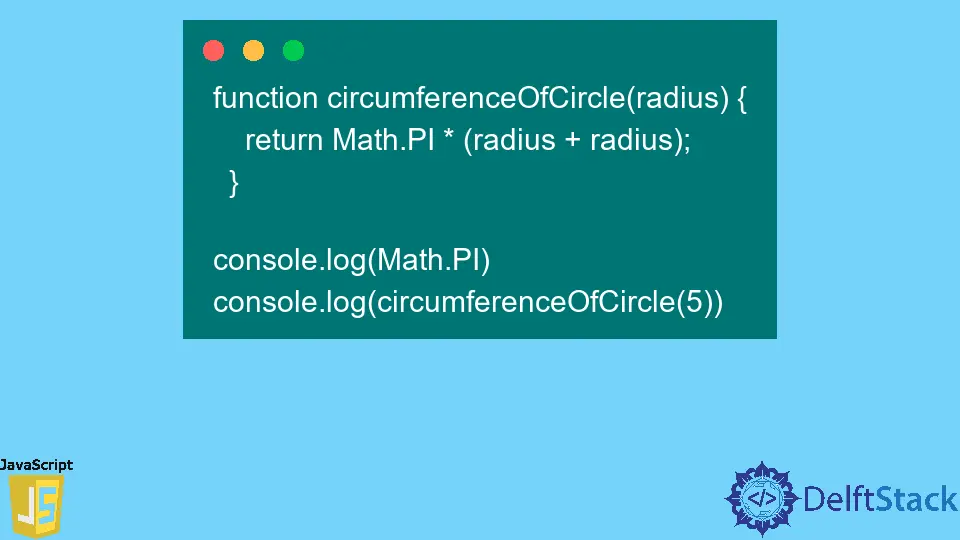
In Mathematics, PI is considered the constant and is presented in the Greek letter P as π. It represents the ratio of the circumference of a circle to its diameter, whose approximate value is 3.1415.
JavaScript provides Math.PI
property to work with PI.
Syntax of Math.PI
Math.PI
Parameters
The Math.PI
property does not accept any parameter.
Return
The Math.PI
property returns PI’s value of approximately 3.1415.
Example 1: Use Math.PI
Property to Get Circumference of a Circle
function circumferenceOfCircle(radius) {
return Math.PI * (radius + radius);
}
console.log(Math.PI)
console.log(circumferenceOfCircle(5))
Output:
3.141592653589793
31.41592653589793
First, we print the value of PI with the help of the Math.PI
property.
Second, one common task often performed with PI is calculating the circumference of a circle. It is the linear distance of a circle.
To calculate it, we create a function that accepts radius
as the parameter and then uses the PI * (radius + radius)
formula to return the calculated result. Finally, when we call this function with the radius
, it gives us the circumference of a circle.
Example 2: Use Math.PI
Property to Get Surface Area of a Circle
function areaOfCircle(radius) {
return Math.PI * (radius * radius)
}
console.log(areaOfCircle(5))
Output:
78.53981633974483
The surface area of a circle means the amount of area a circle has covered. The formula for calculating it is PI * (radius * radius)
.
We declare a function that takes the radius of a circle as the parameter and returns the surface area of a circle after calculating it.
Example 3: TypeError
Shows When Math.PI
Is Used as a Function
valueOfPI = Math.PI()
console.log(valueOfPI)
Output:
TypeError: Math.PI is not a function
The property of an object stores values; on the other hand, a function is something that can perform a certain action. A function is known as the method when it is used in an object.
If we use this property as a function, a TypeError
will show.
Niaz is a professional full-stack developer as well as a thinker, problem-solver, and writer. He loves to share his experience with his writings.
LinkedIn