JavaScript Math.max() Method
-
Syntax of
Math.max()
-
Example 1: Use the
Math.max()
Method in JavaScript -
Example 2: Use the
Array.reduce()
With theMath.max()
Method -
Example 3: Use the
Function.prototype.apply()
With theMath.max()
Method -
Example 4: Use the Spread Operator With the
Math.max()
Method -
Example 5: Use the
Math.max()
Method With Exception
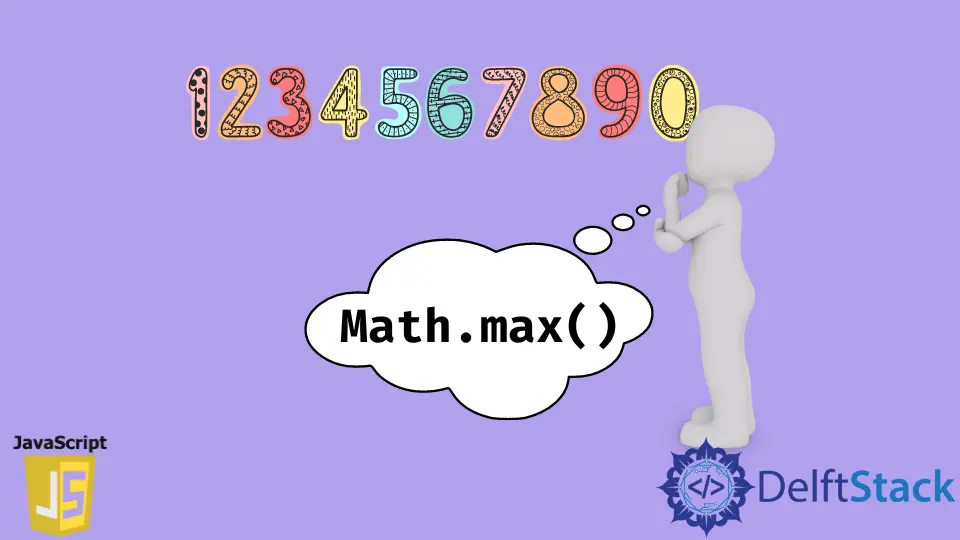
The Math.max()
method finds out the largest number from the given numbers. We can pass multiple numbers as the parameter.
But in general, it expects at least two numbers as the parameter so that it can compare them and return the largest one.
Syntax of Math.max()
Math.max(num1, num2, ....., numN)
Parameters
num |
The parameter num refers to a numeric value. |
Return
The Math.max()
method returns the highest value from the given values as the parameter.
If any of the values passed in the parameter is non-numeric that can be converted as NaN
, this method will return NaN
.
This method will return -Infinity
if nothing is passed as the parameter.
Example 1: Use the Math.max()
Method in JavaScript
const result1 = Math.max(1, 21, 11, 40, 5)
const result2 = Math.max(-10, -32, -33, -3, -6)
console.log(result1)
console.log(result2)
Output:
40
-3
First, we take some positive numbers and use this method to find the maximum value. This method returns 40
as it is the highest number among the given numbers.
Second, we take some negative numbers to find out the maximum value. It returns -3
, which is the largest number among all given negative numbers.
Example 2: Use the Array.reduce()
With the Math.max()
Method
const arr1 = [10, 50, 1, 33, 20]
const arr2 = []
const result1 = arr1.reduce((x, y) => Math.max(x, y), -Infinity)
const result2 = arr2.reduce((x, y) => Math.max(x, y), -Infinity)
console.log(result1)
console.log(result2)
Output:
50
-Infinity
The Array.reduce()
method iterates the whole array and applies the Math.max()
method. It compares the numeric values of the array and returns the largest one.
It will return -Infinity
if the array does not contain any value.
Example 3: Use the Function.prototype.apply()
With the Math.max()
Method
const arr = [10, 50, 1, 40, 11]
function getMaxNumFromArray(arr){
return Math.max.apply(null, arr)
}
console.log(getMaxNumFromArray(arr))
Output:
50
The Math.max()
method does not work with an array. The Function.prototype.apply()
is used with Math.max()
to get the maximum number from an array.
Example 4: Use the Spread Operator With the Math.max()
Method
const arr = [10, 50, 31, 17, 26]
const result = Math.max(...arr)
console.log(result)
Output:
50
The spread operator ...
converts an array into individual elements. The Math.max()
method finds the largest number among those elements of an array.
Example 5: Use the Math.max()
Method With Exception
const result1 = Math.max(11, 22, 'a')
const result2 = Math.max()
console.log(result1)
console.log(result2)
Output:
NaN
-Infinity
First, we pass some numbers and a string as the parameter of this method. This method returns NaN
as it cannot deal with non-numeric numbers that can be converted into NaN
.
Second, we pass nothing in the parameter of this method, and it returns -Infinity
.
Niaz is a professional full-stack developer as well as a thinker, problem-solver, and writer. He loves to share his experience with his writings.
LinkedIn