How to Convert a Float Number to Int in JavaScript
-
Convert Float to Int Using the
parseInt()
Function in JavaScript -
Convert Float to Int Using
Number.toFixed()
in JavaScript - Convert Float to Int With Bitwise Operators in JavaScript
-
Convert Float to Int Using
Math
Library Functions in JavaScript - Conclusion
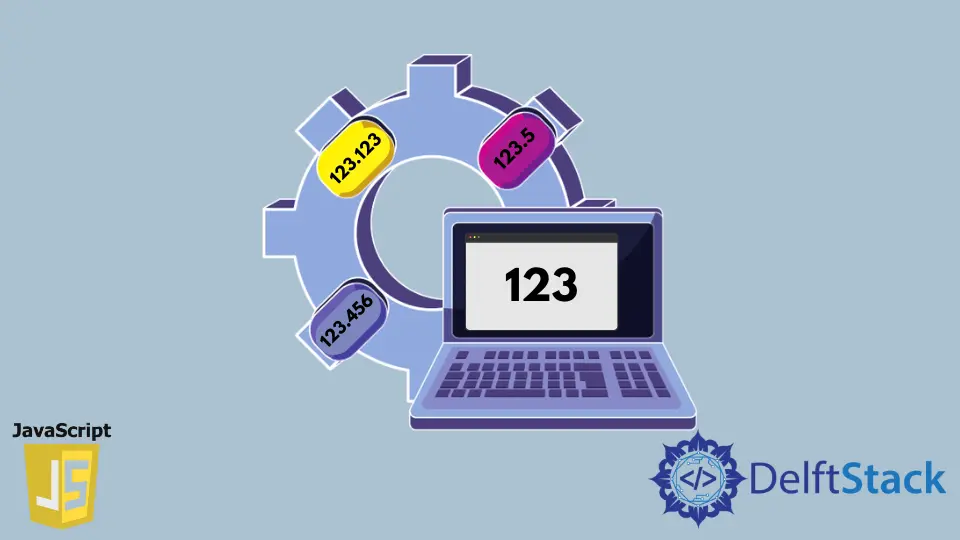
In JavaScript, we have many ways of converting float to int, as shown below.
- The
parseInt()
function - The
Number.toFixed()
method - Conversion with bitwise operators
- Applying
OR
by 0 - Using the double
NOT
operator - Right
SHIFT
by 0
- Applying
- Using
Math
library functions in javascriptMath.floor()
Math.round()
Math.ceil()
Math.trunc()
Convert Float to Int Using the parseInt()
Function in JavaScript
parseInt()
is widely used in JavaScript. With this function, we can convert the values of different data types to the integer type. In this section, we will see the conversion of a float number to an integer number. The syntax of parseInt()
is parseInt(<value>)
. The following code shows the behavior of the function.
console.log(parseInt(123.321));
console.log(parseInt(123.998));
Output:
123
123
Remarks
- For more information, refer to the MSN docs.
- While converting float values,
parseInt()
discards the decimals and doesn’t consider them while returning the whole number part of the float value. parseInt()
returnsNaN
in case conversion is not possible. Hence, it is good to include anisNaN()
check while converting values so that the code is safe and doesn’t break.
Convert Float to Int Using Number.toFixed()
in JavaScript
Number.toFixed()
behaves a bit differently from the parseInt()
. It rounds off the number to the nearest integer value. Hence, beware of the rounding fact. Number.toFixed()
rounds of the decimal value of float less than .5
to the lower integer value. If the decimal value is 0.5
or greater, then the float number will be rounded off to the next higher integer value. The syntax for Number.toFixed()
function is as follows.
numberObject.toFixed(<number of digits>);
Usually, we need to pass a parameter to this function that specifies the number of digits after the decimal in the output. For our purpose, we need not mention any argument as, by default, it takes the parameter to be 0
, hence returning an integer value. Refer to the following sample codes.
console.log( (123.123).toFixed(2) );
console.log( (123.123).toFixed() );
console.log( (123.98).toFixed() );
console.log( (123.49).toFixed() );
console.log( (123.5).toFixed() );
Output:
123.12
123
124
123
124
Remarks
- Unlike the
parseInt()
method,Number.toFixed()
is not that famous for extracting the integer part, as it does a round-up of the float value passed as a parameter. - One can use this function to convert only float values. Unlike the
parseInt()
function, string value conversion to float is not supported byNumber.toFixed()
.
Convert Float to Int With Bitwise Operators in JavaScript
We have seen the methods of parseInt()
and the Number.toFixed()
. Both of these execute some internal operations to get the desired results. The bitwise operators are efficient, fast, and per-formant in converting the float values into an integer when compared to the two methods. We can use the following bitwise operators for the conversion.
Applying OR
by 0
We can use the bitwise OR
operator to get the whole number part of a floating-point number. The bitwise operators function at the binary level. It converts the operand number to binary, and then the bit by bit OR
operation is executed. Applying OR
with 0
to any floating-point number in the acceptable range will return the whole number part of the floating-point value. Refer to the examples below.
console.log(123.321 | 0);
console.log(123.5432 | 0);
console.log(123.921 | 0);
console.log(216 | 0);
console.log(-321.456 | 0)
Output:
123
123
123
216
-321
Using the Double NOT
Operator
Another bitwise operator is the NOT
operator, represented by the ~
sign. Being a unary operator, we can use the bitwise NOT
operator to shed off the decimal part in a float number. The NOT
operator, at the binary level, inverts the binary bit values (returning 0
for a 1
bit and 1
for a 0
bit value). It means that if a number is represented in binary by 10110
, then applying NOT
to it gives the inverted value 01001
. Again applying the NOT
functionality returns back the original bits (10110
) of the number. Hence, applying the NOT
operator twice returns the numeric value, and during the process, it does not change the number if it is an integer. But for a float value, applying the NOT
operator twice will return just the whole number part of the float number. The following examples clarify this fact.
console.log(~~(123.321));
console.log(~~(123.53));
console.log(~~(23.97));
console.log(~~(-23.97));
console.log(~~(-0.97));
Output:
123
123
23
-23
0
Right Shift by 0
We can apply the bitwise right shift operator (represented by >>
characters). It will convert the float value to an integer. Underneath at the binary level, the bitwise right SHIFT operator shifts the binary bits of the operand to the right by a count specified by the second operand. Hence, it ignores the bits that overflow to the right. In doing so, the function preserves the sign value of the number. The following codes represent the working of the right SHIFT operator.
console.log(123.321 >> 0);
console.log(123.53 >> 0);
console.log(23.97 >> 0);
console.log(-23.97 >> 0);
console.log(-0.97 >> 0);
Output:
123
123
23
-23
0
Remarks
- Bitwise operators are faster as it is closest to the machine language and doesn’t need to perform additional check or operations as would be done internally by the
parseInt()
orNumber.toFixed()
. - Bitwise operators like the
OR
,NOT
etc., when applied on large numbers, may give unexpected results. The maximum value supported for getting the decimal value truncated is2147483647
(231-1). Any number more than2147483647
will give unexpected results. - In JavaScript, floating-point numbers are represented by 64 bits, with one bit reserved for preserving the sign value (positive or negative), 32 bits for the whole number part. The bitwise operators operate on the signed 32-bit numbers ignoring the decimal value. Hence, we get the integer number part of the float value.
Convert Float to Int Using Math
Library Functions in JavaScript
We can use the JavaScript Math
library, which has various functions to get integers from floating-point numbers. Unlike the bitwise operators, these offer additional features based on requirements like rounding off values to the nearest numbers, truncating values, etc. We can use the following methods for this purpose.
Conversion With Math.floor()
The Math.floor()
function rounds off the value passed as a parameter to the next lower integer value. Syntax of Math.floor()
is Math.floor(<float value>)
. Following are a few examples that showcase the usage and how it converts a float to a whole number.
console.log(Math.floor(123.321));
console.log(Math.floor(123.53));
console.log(Math.floor(23.97));
console.log(Math.floor(-23.97));
console.log(Math.floor(-0.97));
Output:
123
123
23
-24
-1
The case of negative float numbers like Math.floor(-23.97)
may seem confusing, but the function rightly converts the value to the next lower integer, -24
. Recollect that the higher the numeric value of a negative number, the lesser is its actual value.
Conversion With Math.round()
Unlike the Math.floor()
function, Math.round()
approximates the value passed in parameters. If the value after the decimal is 5
or greater, then the number is rounded off to the next higher integer. Else, if the value after the decimal is lesser than 5
, it is rounded to the lower integer. Hence, based on the value post the decimal point, Math.round()
function can behave similar to Math.floor()
or Math.ceil()
functions. Following are a few examples with Math.round()
.
console.log(Math.round(123.321));
console.log(Math.round(123.53));
console.log(Math.round(23.97));
console.log(Math.round(-23.97));
console.log(Math.round(-0.97));
Output:
123
124
24
-24
-1
Conversion With Math.ceil()
The Math.ceil()
function behaves opposite to the Math.floor()
function. Instead of rounding off to the next lower integer as in Math.floor()
, Math.ceil()
returns the next higher integer value. Following are a few conversions with Math.ceil()
.
console.log(Math.ceil(123.321));
console.log(Math.ceil(123.53));
console.log(Math.ceil(23.97));
console.log(Math.ceil(-23.97));
console.log(Math.ceil(-0.97));
Output:
124
124
24
-23
-0
Conversion With Math.trunc()
As the name hints, the Math.trunc()
function truncates the decimal value and returns the whole number part of the float value. This method can be considered similar to the bitwise operators discussed earlier. Here, there is no rounding off to the nearest integers. Instead, it returns the whole number part of the float value as it is. Following are a few use cases with Math.trunc()
.
console.log(Math.trunc(123.321));
console.log(Math.trunc(123.53));
console.log(Math.trunc(23.97));
console.log(Math.trunc(-23.97));
console.log(Math.trunc(-0.97));
Output:
123
123
23
-23
-0
Conclusion
There are various ways to get the whole number part out of a float value. Bitwise operators are faster in execution as they perform operations on the binary-level without the requirements of conversion or any other processing. parseInt()
, Math.trunc()
and the bitwise operators (OR
by 0
, Double NOT
, right shift by 0
) give the exact whole number as if they tear the float value at the decimal point and return just the whole number part out of it. If we are interested in processing the float number to round it or get the nearest integer based on the business requirement, it would be good to go for Number.toFixed()
, Math.round()
, Math.ceil()
and the Math.floor()
functions.