JavaFX setFill() Method
-
Introduction to the
setFill()
Method in JavaFX -
Basic Examples of the
setFill()
Method in JavaFX -
Use
setFill()
With Different Shapes - Working With Colors
- Best Practices and Tips
- Conclusion
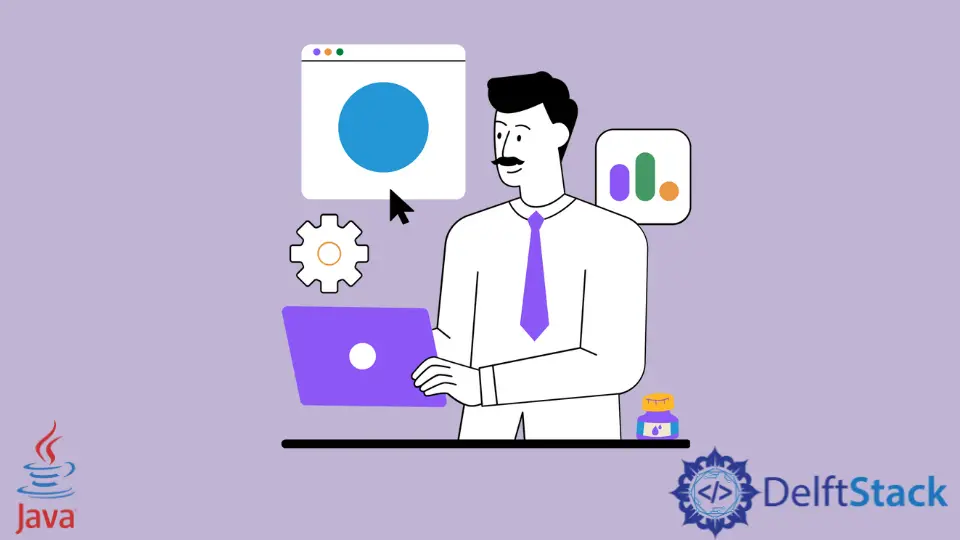
JavaFX is a powerful framework for building rich, interactive user interfaces for desktop, mobile, and embedded applications. One of the fundamental aspects of creating visually appealing JavaFX applications is the ability to work with shapes and colors.
The setFill()
method is a crucial tool in this regard, allowing developers to set the fill color of various shapes. This method is used to fill the colors in shapes and other elements in JavaFX.
In this article, we will explore the setFill()
method in detail, covering its syntax, usage, and examples to help you harness its full potential in your JavaFX applications.
Introduction to the setFill()
Method in JavaFX
The setFill()
method is part of the JavaFX Shape
class, which is the base class for all geometric shapes in JavaFX. It is used to specify the fill color of a shape, allowing developers to customize the visual appearance of the application.
The setFill()
method can fill uniform image patterns and gradient patterns to the shapes in JavaFX. To use the setFill()
method, we need the JavaFX.scene.paint package
. The setFill()
can be used to fill the colors to the classes like Shape
, Text
etc.
Syntax:
// Setting color to the text
Color color = new Color.Red text.setFill(color);
The above syntax uses to Color
class from the paint
package to specify the color and fill it to the text using the setFill()
method.
The setFill()
method takes a single parameter, which is of type Paint
. Paint
is an abstract class in JavaFX that represents a generic way of filling shapes, including color gradients and images.
Basic Examples of the setFill()
Method in JavaFX
Filling a Rectangle With a Gradient
This code creates a Rectangle
object positioned at (100, 100
) with a width of 200
units and a height of 150
units. It then defines a linear gradient that transitions from red to yellow, spanning from left to right.
Finally, it sets the fill of the rectangle to this gradient, resulting in a visually appealing color transition within the rectangle.
Rectangle rectangle = new Rectangle(100, 100, 200, 150);
LinearGradient gradient = new LinearGradient(
0, 0, 1, 0, true, CycleMethod.NO_CYCLE, new Stop(0, Color.RED), new Stop(1, Color.YELLOW));
rectangle.setFill(gradient);
Using an Image as Fill
This code creates a Rectangle
object positioned at (100, 100
) with a width of 200
units and a height of 150
units. It then defines an ImagePattern
using an image file located at "path/to/image.jpg"
.
Finally, it sets the fill of the rectangle to this ImagePattern
, resulting in the rectangle being filled with the specified image. This is a way to customize the appearance of the shape using an image pattern.
Rectangle rectangle = new Rectangle(100, 100, 200, 150);
ImagePattern pattern = new ImagePattern(new Image("path/to/image.jpg"));
rectangle.setFill(pattern);
Example 1: Fill Color Into the Scene Using the setFill()
Method in JavaFX
The following are the steps to fill color into the scene using the setFill()
method.
-
Create a class that extends the
Application
class and implements thestart()
method. -
Create a group by instantiating the
Group
class. -
Create a scene by instantiating the
Scene
class and passing thegroup
to it. -
Fill the colors to the scene using the
setFill
method. -
Create a shape, circle, rectangle, etc., and add the shape to the
group
. -
Pass the
scene
to the stage and display the stage by theShow
method. -
Launch the application in the
main
method.
Let’s have an example based on the steps above. This code is a simple JavaFX application that creates a window with a light blue background and a red circle in the center.
package delftstack;
import javafx.application.Application;
import javafx.scene.*;
import javafx.scene.paint.Color;
import javafx.scene.shape.*;
import javafx.stage.Stage;
public class JavaFX_SetFill extends Application {
@Override
public void start(Stage DemoStage) {
Group DemoGroup = new Group();
Scene DemoScene = new Scene(DemoGroup, 200, 150);
DemoScene.setFill(Color.LIGHTBLUE);
Circle DemoCircle = new Circle(100, 100, 80, Color.RED);
DemoGroup.getChildren().add(DemoCircle);
DemoStage.setScene(DemoScene);
DemoStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
The code above will create a scene with a circle shape. It uses the setFill()
method to fill color into the scene.
It creates a simple JavaFX application that displays a window with a light blue background and a red circle in the center. The application uses JavaFX’s scene graph, which involves creating nodes (like shapes) and adding them to a scene, which is then displayed on a stage.
Output:
Example 2: Fill Color to Shape and Text Using the setFill()
Method in JavaFX
Let’s try to fill the color to the shape and text using the setFill()
method. This Java program is an example of a JavaFX application that creates a window with a colored square and some text.
package delftstack;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.scene.text.Font;
import javafx.scene.text.Text;
import javafx.stage.Stage;
public class JavaFX_SetFill extends Application {
@Override
public void start(Stage DemoStage) {
// Draw a Square
Rectangle Square = new Rectangle();
// Set the properties of the Square
Square.setX(200.0f);
Square.setY(200.0f);
Square.setWidth(300.0f);
Square.setHeight(300.0f);
// Set a color to the Square
Square.setFill(Color.LIGHTBLUE);
// Set the stroke width
Square.setStrokeWidth(3);
// Set color to the stroke
Square.setStroke(Color.LIGHTGREEN);
// Draw a text
Text DemoText = new Text("This is a colored Square");
// Set the font of the text
DemoText.setFont(Font.font("Edwardian Script ITC", 60));
// Set the position of the text
DemoText.setX(155);
DemoText.setY(50);
// Set color to the text
DemoText.setFill(Color.BEIGE);
DemoText.setStrokeWidth(2);
DemoText.setStroke(Color.LIGHTBLUE);
// Create a Group object
Group Group_Root = new Group(Square, DemoText);
// Create a scene object
Scene DemoScene = new Scene(Group_Root, 600, 300);
// Set title to the Stage
DemoStage.setTitle("SetFill Example");
// Add scene to the stage
DemoStage.setScene(DemoScene);
// Display the contents of the stage
DemoStage.show();
}
public static void main(String args[]) {
launch(args);
}
}
The code above will create a square and a text and then use the setfill
method to fill the square with color. It also uses the setStroke
method for the borders.
This code creates a JavaFX window with a colored square and a text message. The square has a light blue fill and a light green border. The text displays the message "This is a colored Square"
in a custom font, with a beige fill color and a light blue border.
Output:
Example 3: Fill Image Gradient to Shape Using the setFill()
Method in JavaFX
The setFill
method can also fill an image gradient to the shape or text. This Java program is an example of a JavaFX application that creates a window with a square filled using an image pattern.
package delftstack;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.image.Image;
import javafx.scene.paint.Color;
import javafx.scene.paint.ImagePattern;
import javafx.scene.shape.Rectangle;
import javafx.scene.text.Font;
import javafx.scene.text.Text;
import javafx.stage.Stage;
public class JavaFX_SetFill extends Application {
@Override
public void start(Stage DemoStage) throws FileNotFoundException {
// Draw a Square
Rectangle Square = new Rectangle();
// Set the properties of the Square
Square.setX(200.0f);
Square.setY(200.0f);
Square.setWidth(300.0f);
Square.setHeight(300.0f);
// Draw a text
Text DemoText = new Text("This is a Gradient Square");
// Set the font of the text
DemoText.setFont(Font.font("Edwardian Script ITC", 60));
// Set the position of the text
DemoText.setX(155);
DemoText.setY(50);
// Set the image pattern
Image DemoImage = new Image(new FileInputStream("Delftstack.png"));
ImagePattern Image_Gradient = new ImagePattern(DemoImage, 80, 80, 160, 160, false);
// Set the linear gradient to the Square
Square.setFill(Image_Gradient);
// Create a Group object
Group Group_Root = new Group(Square, DemoText);
// Create a scene object
Scene DemoScene = new Scene(Group_Root, 600, 300);
// Set title to the Stage
DemoStage.setTitle("SetFill Example");
// Add scene to the stage
DemoStage.setScene(DemoScene);
// Display the contents of the stage
DemoStage.show();
}
public static void main(String args[]) {
launch(args);
}
}
The code above will fill the image gradient to the square shape. It creates a JavaFX application with a window containing a square filled with the image specified, along with a text element. The square’s position, size, and fill are customized, and everything is displayed in a window with a specified size.
Output:
Use setFill()
With Different Shapes
Rectangle
This code snippet creates a Rectangle
object with a specified position and size. It then sets the fill color of the rectangle to blue using the setFill()
method provided by JavaFX.
The resulting rectangle will be positioned at (100, 100
) with a width of 200
units and a height of 150
units, and it will be filled with a solid blue color.
Rectangle rectangle = new Rectangle(100, 100, 200, 150);
rectangle.setFill(Color.BLUE);
Circle
This code snippet creates a Circle
object with a specified center position and radius. It then sets the fill color of the circle to green using the setFill()
method provided by JavaFX.
The resulting circle will have its center at (150, 150
) and a radius of 50
units, and it will be filled with a solid green color.
Circle circle = new Circle(150, 150, 50);
circle.setFill(Color.GREEN);
Polygon
This code snippet creates a Polygon
object and defines its vertices to form a triangle. It then sets the fill color of the triangle to red using the setFill()
method provided by JavaFX.
The resulting triangle has vertices at (100.0, 100.0
), (200.0, 200.0
), and (300.0, 100.0
), and it is filled with a solid red color.
Polygon polygon = new Polygon();
polygon.getPoints().addAll(new Double[] {100.0, 100.0, 200.0, 200.0, 300.0, 100.0});
polygon.setFill(Color.RED);
Ellipse
This code creates an Ellipse
object with a specified center position with horizontal and vertical radii. It then sets the fill color of the ellipse to yellow using the setFill()
method provided by JavaFX.
The resulting ellipse will have its center at (150, 150
), a horizontal radius of 50
units, and a vertical radius of 30
units, and it will be filled with a solid yellow color.
Ellipse ellipse = new Ellipse(150, 150, 50, 30);
ellipse.setFill(Color.YELLOW);
Line
This code creates a Line
object with specific starting and ending coordinates. It then sets the stroke width of the line to 5
units, making it thicker.
However, attempting to set the fill color to orange has no effect because lines do not have a fill property. The setFill()
method is applicable to shapes but not to lines.
Line line = new Line(50, 50, 200, 200);
line.setStrokeWidth(5);
line.setFill(Color.ORANGE); // This won't affect the appearance of the line
Working With Colors
The setFill()
method accepts any subclass of the Paint
class. This includes the Color
class, which is commonly used for solid color fills.
You can create colors in JavaFX using predefined constants from the Color
class or by specifying RGB values:
Color color = Color.rgb(255, 0, 0); // Red
Best Practices and Tips
- Avoid setting the fill color of a
Line
object. ThesetFill()
method does not affect lines. - Experiment with different
Paint
subclasses, such as gradients and image patterns, to create unique visual effects. - Remember that the order of shapes in a JavaFX scene determines which shapes are drawn on top of others.
Conclusion
The setFill()
method is a fundamental tool in JavaFX for customizing the visual appearance of shapes. By understanding its usage and exploring different examples, you can create visually appealing and dynamic user interfaces in your JavaFX applications. Experiment with different colors, gradients, and patterns to achieve the desired visual effects.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook