JavaFX setFill() 方法
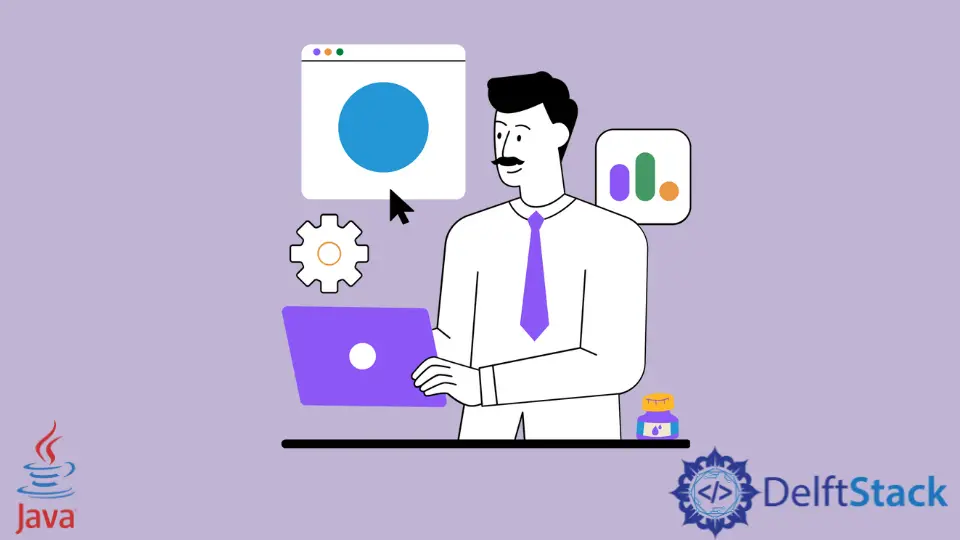
setFill()
方法用於在 JavaFX 中填充形狀和其他元素的顏色。本教程演示了在 JavaFX 中使用 setFill()
方法。
JavaFX setFill()
方法
setFill()
方法可以將統一的影象圖案和漸變圖案填充到 JavaFX 中的形狀中。要使用 setFill()
方法,我們需要 JavaFX.scene.paint 包
。
setFill()
可用於將顏色填充到 Shape
、Text
等類中。
語法:
//Setting color to the text
Color color = new Color.Red
text.setFill(color);
上面的語法使用 paint 包中的 Color
類來指定顏色並使用 setFill()
方法將其填充到文字中。以下是使用 setFill
方法將顏色填充到場景中的步驟。
- 建立一個擴充套件 Application 類並實現
start()
方法的類。 - 通過例項化
Group
類來建立一個組。 - 通過例項化
Scene
類並將group
傳遞給它來建立一個場景。 - 使用
setFill
方法將顏色填充到場景中。 - 建立一個形狀、圓形、矩形等,並將該形狀新增到
組
。 - 將
scene
傳遞給舞臺,並通過Show
方法顯示舞臺。 - 在
main
方法中啟動應用程式。
讓我們有一個基於上述步驟的示例。
示例程式碼:
package delftstack;
import javafx.application.Application;
import javafx.scene.*;
import javafx.scene.paint.Color;
import javafx.scene.shape.*;
import javafx.stage.Stage;
public class JavaFX_SetFill extends Application {
@Override
public void start(Stage DemoStage) {
Group DemoGroup = new Group();
Scene DemoScene = new Scene(DemoGroup, 200, 150);
DemoScene.setFill(Color.LIGHTBLUE);
Circle DemoCircle = new Circle(100, 100, 80, Color.RED);
DemoGroup.getChildren().add(DemoCircle);
DemoStage.setScene(DemoScene);
DemoStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
上面的程式碼將建立一個圓形的場景。它使用 setFill
方法為場景填充顏色。
輸出:
讓我們嘗試使用 setFill()
方法為形狀和文字填充顏色。
示例程式碼:
package delftstack;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.scene.text.Font;
import javafx.scene.text.Text;
import javafx.stage.Stage;
public class JavaFX_SetFill extends Application {
@Override
public void start(Stage DemoStage) {
// Draw a Square
Rectangle Square = new Rectangle();
// Set the properties of the Square
Square.setX(200.0f);
Square.setY(200.0f);
Square.setWidth(300.0f);
Square.setHeight(300.0f);
// Set color to the Square
Square.setFill(Color.LIGHTBLUE);
// Set the stroke width
Square.setStrokeWidth(3);
// Set color to the stroke
Square.setStroke(Color.LIGHTGREEN);
// Draw a text
Text DemoText = new Text("This is a colored Square");
// Set the font of the text
DemoText.setFont(Font.font("Edwardian Script ITC", 60));
// Set the position of the text
DemoText.setX(155);
DemoText.setY(50);
// Set color to the text
DemoText.setFill(Color.BEIGE);
DemoText.setStrokeWidth(2);
DemoText.setStroke(Color.LIGHTBLUE);
// Create a Group object
Group Group_Root = new Group(Square, DemoText);
// Create a scene object
Scene DemoScene = new Scene(Group_Root, 600, 300);
// Set title to the Stage
DemoStage.setTitle("SetFill Example");
// Add scene to the stage
DemoStage.setScene(DemoScene);
// Display the contents of the stage
DemoStage.show();
}
public static void main(String args[]) {
launch(args);
}
}
上面的程式碼將建立一個正方形和一個文字,然後使用 setfill
方法為正方形填充顏色。它還對邊框使用 setStroke
方法。
輸出:
setFill
方法還可以將影象漸變填充到形狀或文字中。
示例程式碼:
package delftstack;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.image.Image;
import javafx.scene.paint.Color;
import javafx.scene.paint.ImagePattern;
import javafx.scene.shape.Rectangle;
import javafx.scene.text.Font;
import javafx.scene.text.Text;
import javafx.stage.Stage;
public class JavaFX_SetFill extends Application {
@Override
public void start(Stage DemoStage) throws FileNotFoundException {
// Draw a Square
Rectangle Square = new Rectangle();
// Set the properties of the Square
Square.setX(200.0f);
Square.setY(200.0f);
Square.setWidth(300.0f);
Square.setHeight(300.0f);
// Draw a text
Text DemoText = new Text("This is a Gradient Square");
// Set the font of the text
DemoText.setFont(Font.font("Edwardian Script ITC", 60));
// Set the position of the text
DemoText.setX(155);
DemoText.setY(50);
// Set the image pattern
Image DemoImage = new Image(new FileInputStream("Delftstack.png"));
ImagePattern Image_Gradient = new ImagePattern(DemoImage, 80, 80, 160, 160, false);
// Set the linear gradient to the Square
Square.setFill(Image_Gradient);
// Create a Group object
Group Group_Root = new Group(Square, DemoText);
// Create a scene object
Scene DemoScene = new Scene(Group_Root, 600, 300);
// Set title to the Stage
DemoStage.setTitle("SetFill Example");
// Add scene to the stage
DemoStage.setScene(DemoScene);
// Display the contents of the stage
DemoStage.show();
}
public static void main(String args[]) {
launch(args);
}
}
上面的程式碼將影象漸變填充為方形。
輸出:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook