Méthode JavaFX setFill()
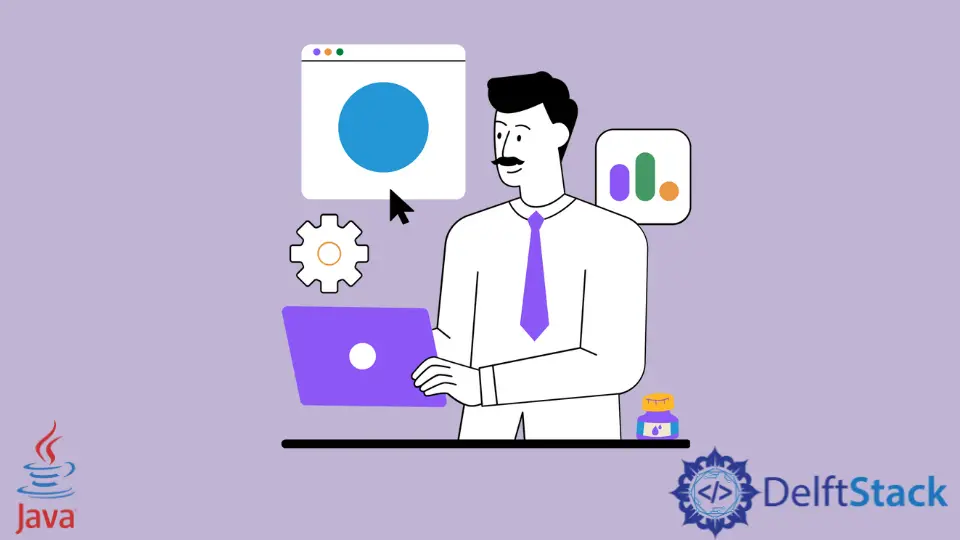
La méthode setFill()
est utilisée pour remplir les couleurs des formes et d’autres éléments dans JavaFX. Ce didacticiel illustre l’utilisation de la méthode setFill()
dans JavaFX.
JavaFX Méthode setFill()
La méthode setFill()
peut remplir des motifs d’image uniformes et des motifs de dégradé aux formes dans JavaFX. Pour utiliser la méthode setFill()
, nous avons besoin du paquet JavaFX.scene.paint
.
Le setFill()
peut être utilisé pour remplir les couleurs des classes comme Shape
, Text
etc.
Syntaxe:
//Setting color to the text
Color color = new Color.Red
text.setFill(color);
La syntaxe ci-dessus utilise la classe Color
du package de peinture pour spécifier la couleur et la remplir avec le texte à l’aide de la méthode setFill()
. Voici les étapes pour remplir la scène en couleur à l’aide de la méthode setFill()
.
- Créez une classe qui étend la classe Application et implémente la méthode
start()
. - Créez un groupe en instanciant la classe
Group
. - Créez une scène en instanciant la classe
Scene
et en lui passant legroup
. - Remplissez les couleurs de la scène en utilisant la méthode
setFill
. - Créez une forme, un cercle, un rectangle, etc., et ajoutez la forme au
group
. - Passez la
scene
à la scène et affichez la scène par la méthodeAfficher
. - Lancez l’application dans la méthode
main
.
Prenons un exemple basé sur les étapes ci-dessus.
Exemple de code :
package delftstack;
import javafx.application.Application;
import javafx.scene.*;
import javafx.scene.paint.Color;
import javafx.scene.shape.*;
import javafx.stage.Stage;
public class JavaFX_SetFill extends Application {
@Override
public void start(Stage DemoStage) {
Group DemoGroup = new Group();
Scene DemoScene = new Scene(DemoGroup, 200, 150);
DemoScene.setFill(Color.LIGHTBLUE);
Circle DemoCircle = new Circle(100, 100, 80, Color.RED);
DemoGroup.getChildren().add(DemoCircle);
DemoStage.setScene(DemoScene);
DemoStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Le code ci-dessus créera une scène avec une forme de cercle. Il utilise la méthode setFill
pour colorer la scène.
Production:
Essayons de remplir la couleur de la forme et du texte en utilisant la méthode setFill()
.
Exemple de code :
package delftstack;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.scene.text.Font;
import javafx.scene.text.Text;
import javafx.stage.Stage;
public class JavaFX_SetFill extends Application {
@Override
public void start(Stage DemoStage) {
// Draw a Square
Rectangle Square = new Rectangle();
// Set the properties of the Square
Square.setX(200.0f);
Square.setY(200.0f);
Square.setWidth(300.0f);
Square.setHeight(300.0f);
// Set color to the Square
Square.setFill(Color.LIGHTBLUE);
// Set the stroke width
Square.setStrokeWidth(3);
// Set color to the stroke
Square.setStroke(Color.LIGHTGREEN);
// Draw a text
Text DemoText = new Text("This is a colored Square");
// Set the font of the text
DemoText.setFont(Font.font("Edwardian Script ITC", 60));
// Set the position of the text
DemoText.setX(155);
DemoText.setY(50);
// Set color to the text
DemoText.setFill(Color.BEIGE);
DemoText.setStrokeWidth(2);
DemoText.setStroke(Color.LIGHTBLUE);
// Create a Group object
Group Group_Root = new Group(Square, DemoText);
// Create a scene object
Scene DemoScene = new Scene(Group_Root, 600, 300);
// Set title to the Stage
DemoStage.setTitle("SetFill Example");
// Add scene to the stage
DemoStage.setScene(DemoScene);
// Display the contents of the stage
DemoStage.show();
}
public static void main(String args[]) {
launch(args);
}
}
Le code ci-dessus créera un carré et un texte, puis utilisera la méthode setfill
pour remplir le carré de couleur. Il utilise également la méthode setStroke
pour les bordures.
Production:
La méthode setFill
peut également remplir un dégradé d’image à la forme ou au texte.
Exemple de code :
package delftstack;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.image.Image;
import javafx.scene.paint.Color;
import javafx.scene.paint.ImagePattern;
import javafx.scene.shape.Rectangle;
import javafx.scene.text.Font;
import javafx.scene.text.Text;
import javafx.stage.Stage;
public class JavaFX_SetFill extends Application {
@Override
public void start(Stage DemoStage) throws FileNotFoundException {
// Draw a Square
Rectangle Square = new Rectangle();
// Set the properties of the Square
Square.setX(200.0f);
Square.setY(200.0f);
Square.setWidth(300.0f);
Square.setHeight(300.0f);
// Draw a text
Text DemoText = new Text("This is a Gradient Square");
// Set the font of the text
DemoText.setFont(Font.font("Edwardian Script ITC", 60));
// Set the position of the text
DemoText.setX(155);
DemoText.setY(50);
// Set the image pattern
Image DemoImage = new Image(new FileInputStream("Delftstack.png"));
ImagePattern Image_Gradient = new ImagePattern(DemoImage, 80, 80, 160, 160, false);
// Set the linear gradient to the Square
Square.setFill(Image_Gradient);
// Create a Group object
Group Group_Root = new Group(Square, DemoText);
// Create a scene object
Scene DemoScene = new Scene(Group_Root, 600, 300);
// Set title to the Stage
DemoStage.setTitle("SetFill Example");
// Add scene to the stage
DemoStage.setScene(DemoScene);
// Display the contents of the stage
DemoStage.show();
}
public static void main(String args[]) {
launch(args);
}
}
Le code ci-dessus remplira le dégradé de l’image à la forme carrée.
Production:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook