Método JavaFX setFill()
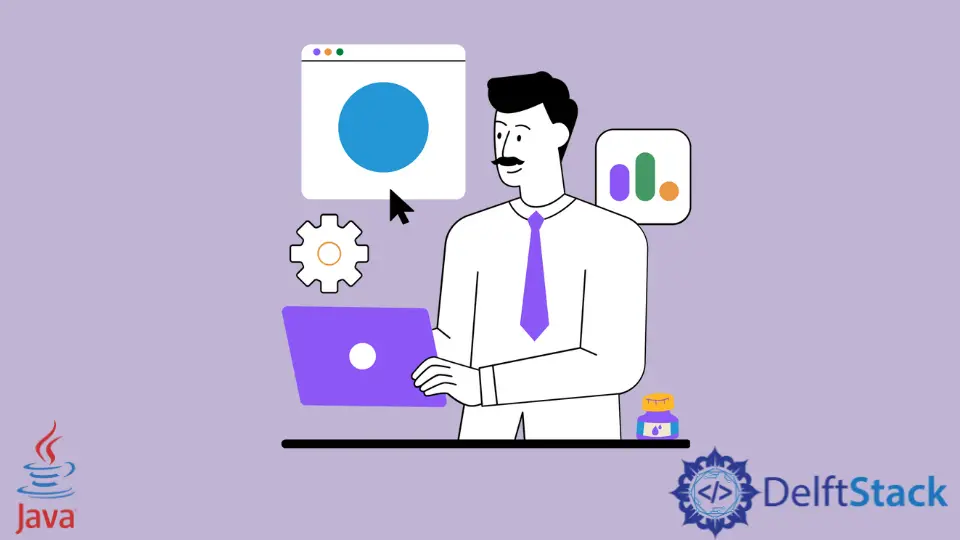
El método setFill()
se usa para rellenar los colores en formas y otros elementos en JavaFX. Este tutorial demuestra el uso del método setFill()
en JavaFX.
Método setFill()
de JavaFX
El método setFill()
puede rellenar patrones de imagen uniformes y patrones de degradado en las formas en JavaFX. Para usar el método setFill()
necesitamos el paquete JavaFX.scene.paint
.
El setFill()
se puede utilizar para rellenar los colores de las clases como Shape
, Text
etc.
Sintaxis:
//Setting color to the text
Color color = new Color.Red
text.setFill(color);
La sintaxis anterior utiliza la clase Color
del paquete de pintura para especificar el color y rellenarlo con el texto utilizando el método setFill()
. Los siguientes son los pasos para llenar de color la escena usando el método setFill
.
- Cree una clase que amplíe la clase Aplicación e implemente el método
start()
. - Cree un grupo instanciando la clase
group
. - Cree una escena instanciando la clase
Scene
y pasándole elgroup
. - Rellena los colores de la escena usando el método
setFill
. - Cree una forma, círculo, rectángulo, etc., y agregue la forma al
group
. - Pase la
Scene
al escenario y muestre el escenario por el métodoShow
. - Inicie la aplicación en el método
main
.
Veamos un ejemplo basado en los pasos anteriores.
Código de ejemplo:
package delftstack;
import javafx.application.Application;
import javafx.scene.*;
import javafx.scene.paint.Color;
import javafx.scene.shape.*;
import javafx.stage.Stage;
public class JavaFX_SetFill extends Application {
@Override
public void start(Stage DemoStage) {
Group DemoGroup = new Group();
Scene DemoScene = new Scene(DemoGroup, 200, 150);
DemoScene.setFill(Color.LIGHTBLUE);
Circle DemoCircle = new Circle(100, 100, 80, Color.RED);
DemoGroup.getChildren().add(DemoCircle);
DemoStage.setScene(DemoScene);
DemoStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
El código anterior creará una escena con forma de círculo. Utiliza el método setFill
para llenar de color la escena.
Producción:
Intentemos rellenar el color de la forma y el texto usando el método setFill()
.
Código de ejemplo:
package delftstack;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.scene.text.Font;
import javafx.scene.text.Text;
import javafx.stage.Stage;
public class JavaFX_SetFill extends Application {
@Override
public void start(Stage DemoStage) {
// Draw a Square
Rectangle Square = new Rectangle();
// Set the properties of the Square
Square.setX(200.0f);
Square.setY(200.0f);
Square.setWidth(300.0f);
Square.setHeight(300.0f);
// Set color to the Square
Square.setFill(Color.LIGHTBLUE);
// Set the stroke width
Square.setStrokeWidth(3);
// Set color to the stroke
Square.setStroke(Color.LIGHTGREEN);
// Draw a text
Text DemoText = new Text("This is a colored Square");
// Set the font of the text
DemoText.setFont(Font.font("Edwardian Script ITC", 60));
// Set the position of the text
DemoText.setX(155);
DemoText.setY(50);
// Set color to the text
DemoText.setFill(Color.BEIGE);
DemoText.setStrokeWidth(2);
DemoText.setStroke(Color.LIGHTBLUE);
// Create a Group object
Group Group_Root = new Group(Square, DemoText);
// Create a scene object
Scene DemoScene = new Scene(Group_Root, 600, 300);
// Set title to the Stage
DemoStage.setTitle("SetFill Example");
// Add scene to the stage
DemoStage.setScene(DemoScene);
// Display the contents of the stage
DemoStage.show();
}
public static void main(String args[]) {
launch(args);
}
}
El código anterior creará un cuadrado y un texto y luego usará el método setfill
para llenar el cuadrado con color. También utiliza el método setStroke
para los bordes.
Producción:
El método setFill
también puede rellenar un degradado de imagen con la forma o el texto.
Código de ejemplo:
package delftstack;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.image.Image;
import javafx.scene.paint.Color;
import javafx.scene.paint.ImagePattern;
import javafx.scene.shape.Rectangle;
import javafx.scene.text.Font;
import javafx.scene.text.Text;
import javafx.stage.Stage;
public class JavaFX_SetFill extends Application {
@Override
public void start(Stage DemoStage) throws FileNotFoundException {
// Draw a Square
Rectangle Square = new Rectangle();
// Set the properties of the Square
Square.setX(200.0f);
Square.setY(200.0f);
Square.setWidth(300.0f);
Square.setHeight(300.0f);
// Draw a text
Text DemoText = new Text("This is a Gradient Square");
// Set the font of the text
DemoText.setFont(Font.font("Edwardian Script ITC", 60));
// Set the position of the text
DemoText.setX(155);
DemoText.setY(50);
// Set the image pattern
Image DemoImage = new Image(new FileInputStream("Delftstack.png"));
ImagePattern Image_Gradient = new ImagePattern(DemoImage, 80, 80, 160, 160, false);
// Set the linear gradient to the Square
Square.setFill(Image_Gradient);
// Create a Group object
Group Group_Root = new Group(Square, DemoText);
// Create a scene object
Scene DemoScene = new Scene(Group_Root, 600, 300);
// Set title to the Stage
DemoStage.setTitle("SetFill Example");
// Add scene to the stage
DemoStage.setScene(DemoScene);
// Display the contents of the stage
DemoStage.show();
}
public static void main(String args[]) {
launch(args);
}
}
El código anterior llenará el degradado de la imagen hasta la forma cuadrada.
Producción:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook