How to Play a Video Using JavaFX
- Setting Up Your JavaFX Environment
- Building the Video Player Interface
- Adding Video Controls
- Enhancing User Experience with Fullscreen Mode
- Conclusion
- FAQ
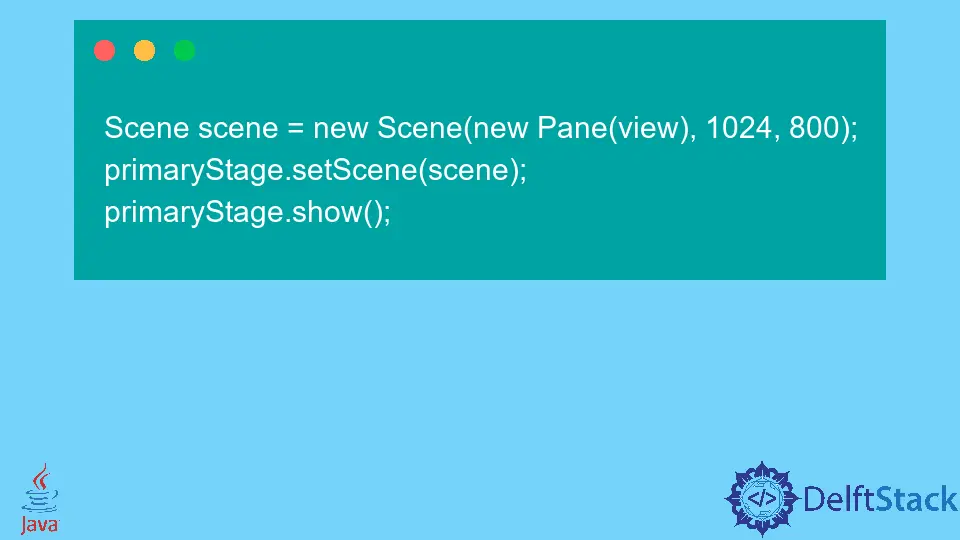
Creating a simple video player in Java can be an exciting project, especially if you’re looking to delve into multimedia applications. JavaFX, with its rich set of features, allows developers to create visually appealing and functional applications.
In this article, we will walk you through the steps to build a basic video player using JavaFX. By the end, you’ll have a better understanding of how to handle video playback, manage user interactions, and enhance your JavaFX skills. Whether you’re a beginner or an experienced developer, this guide will provide you with the knowledge you need to create your own video player.
Setting Up Your JavaFX Environment
Before diving into coding, it’s essential to set up your JavaFX environment correctly. You will need the Java Development Kit (JDK) and the JavaFX SDK. Follow these steps to get started:
- Download and install the latest version of the JDK from the official Oracle website.
- Download the JavaFX SDK from the official Gluon website.
- Configure your IDE (like IntelliJ IDEA or Eclipse) to include the JavaFX libraries in your project.
Once you have everything set up, you’re ready to start coding your video player.
Building the Video Player Interface
The first step in creating your video player is designing the user interface. JavaFX provides a straightforward way to build GUIs using FXML or directly in Java code. Here’s a simple example of how to set up a basic video player interface:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.BorderPane;
import javafx.stage.Stage;
import javafx.scene.media.MediaView;
import javafx.scene.media.Media;
public class VideoPlayer extends Application {
@Override
public void start(Stage primaryStage) {
String videoPath = "file:///path/to/your/video.mp4";
Media media = new Media(videoPath);
MediaView mediaView = new MediaView(media);
BorderPane root = new BorderPane();
root.setCenter(mediaView);
Scene scene = new Scene(root, 800, 600);
primaryStage.setTitle("Simple Video Player");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In this code, we create a basic JavaFX application with a MediaView
to display the video. The Media
class is used to load the video file, and we set the video path accordingly. The BorderPane
layout allows us to center the video within the window. Finally, we launch the application using the launch(args)
method.
Adding Video Controls
Now that we have a basic interface, it’s crucial to add controls for play, pause, and stop functionalities. This enhances user interaction, making your video player more functional. Here’s how you can implement these controls:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Hyperlink;
import javafx.scene.control.ToolBar;
import javafx.scene.layout.BorderPane;
import javafx.scene.media.MediaView;
import javafx.scene.media.Media;
import javafx.scene.media.MediaPlayer;
import javafx.stage.Stage;
public class VideoPlayerWithControls extends Application {
@Override
public void start(Stage primaryStage) {
String videoPath = "file:///path/to/your/video.mp4";
Media media = new Media(videoPath);
MediaPlayer mediaPlayer = new MediaPlayer(media);
MediaView mediaView = new MediaView(mediaPlayer);
Button playButton = new Button("Play");
playButton.setOnAction(e -> mediaPlayer.play());
Button pauseButton = new Button("Pause");
pauseButton.setOnAction(e -> mediaPlayer.pause());
Button stopButton = new Button("Stop");
stopButton.setOnAction(e -> mediaPlayer.stop());
ToolBar toolBar = new ToolBar(playButton, pauseButton, stopButton);
BorderPane root = new BorderPane();
root.setCenter(mediaView);
root.setBottom(toolBar);
Scene scene = new Scene(root, 800, 600);
primaryStage.setTitle("Video Player with Controls");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Output:
The video player will now include buttons for play, pause, and stop functionalities.
In this enhanced version, we introduce buttons for controlling video playback. Each button is linked to a specific action in the MediaPlayer
class—play, pause, and stop. The ToolBar
is used to organize these buttons at the bottom of the window, improving usability.
Enhancing User Experience with Fullscreen Mode
To make your video player even more appealing, you can add a fullscreen option. This feature allows users to enjoy videos without distractions. Here’s how to implement fullscreen functionality in your JavaFX video player:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.ToolBar;
import javafx.scene.layout.BorderPane;
import javafx.scene.media.MediaView;
import javafx.scene.media.Media;
import javafx.scene.media.MediaPlayer;
import javafx.stage.Stage;
public class VideoPlayerWithFullscreen extends Application {
@Override
public void start(Stage primaryStage) {
String videoPath = "file:///path/to/your/video.mp4";
Media media = new Media(videoPath);
MediaPlayer mediaPlayer = new MediaPlayer(media);
MediaView mediaView = new MediaView(mediaPlayer);
Button playButton = new Button("Play");
playButton.setOnAction(e -> mediaPlayer.play());
Button pauseButton = new Button("Pause");
pauseButton.setOnAction(e -> mediaPlayer.pause());
Button stopButton = new Button("Stop");
stopButton.setOnAction(e -> mediaPlayer.stop());
Button fullscreenButton = new Button("Fullscreen");
fullscreenButton.setOnAction(e -> primaryStage.setFullScreen(!primaryStage.isFullScreen()));
ToolBar toolBar = new ToolBar(playButton, pauseButton, stopButton, fullscreenButton);
BorderPane root = new BorderPane();
root.setCenter(mediaView);
root.setBottom(toolBar);
Scene scene = new Scene(root, 800, 600);
primaryStage.setTitle("Video Player with Fullscreen");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Output:
The video player now includes a fullscreen button to enhance viewing experience.
In this code, we add a fullscreen button that toggles the fullscreen mode of the Stage
. When clicked, the button checks the current fullscreen state and switches it accordingly. This feature allows users to immerse themselves in the video content fully.
Conclusion
Creating a simple video player using JavaFX is an excellent way to explore multimedia capabilities within Java applications. By following the steps outlined in this article, you can develop a functional video player that includes essential features like playback controls and fullscreen mode. As you become more comfortable with JavaFX, consider adding more features such as a volume control, a progress bar, or even playlist capabilities. The possibilities are endless, and with practice, you can create a robust multimedia application that enhances user experiences.
FAQ
-
What is JavaFX?
JavaFX is a framework for building rich internet applications in Java, providing a modern UI toolkit for desktop applications. -
Can I play different video formats using JavaFX?
Yes, JavaFX supports various video formats, but the availability depends on the underlying media framework. -
Do I need to install additional libraries for JavaFX?
Yes, you need to download the JavaFX SDK separately and configure it in your IDE. -
How can I improve the performance of my JavaFX video player?
Optimizing video playback settings and ensuring your application runs on a capable machine can improve performance. -
Is JavaFX suitable for mobile applications?
While primarily designed for desktop applications, JavaFX can also be used for mobile development with additional frameworks.