InvocationTargetException in JavaFX
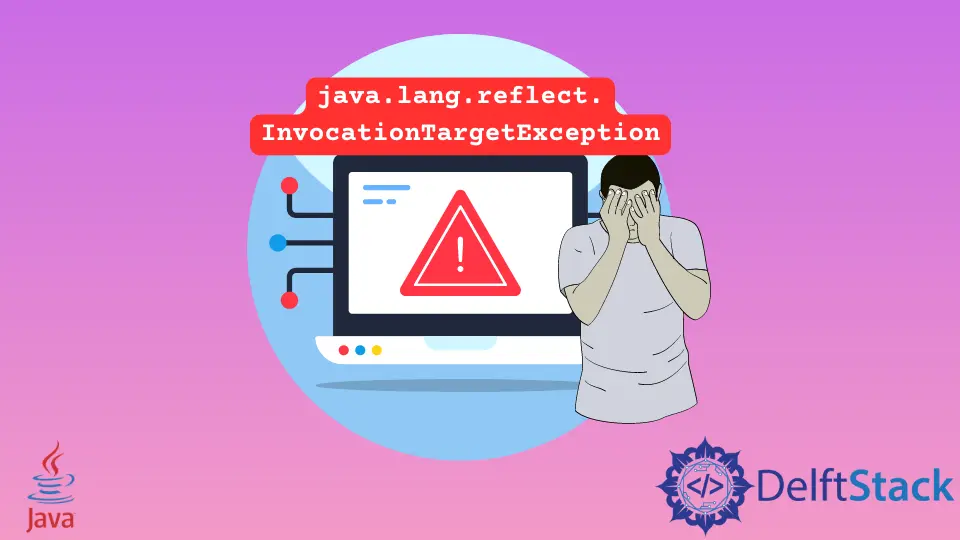
This tutorial demonstrates how to solve the InvocationTargetException
in JavaFX.
Fix InvocationTargetException
in JavaFX
The exception InvocationTargetException
occurs when we are using JavaFX. The reasons this exception occurs are:
- When we write a forward slash at the start of the path name of the FXML file or the path given is not correct or complete.
- When we try to use dynamic root but haven’t set the root on the FXML Loader.
- When we miss a JavaFX Module.
Let’s try an example that will throw the InvocationTargetException
in JavaFX.
package delftstack;
import java.io.File;
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.stage.Stage;
public class Example extends Application {
@Override
public void start(Stage DemoStage) throws Exception {
System.out.println("Start()");
Parent DemoRoot = FXMLLoader.load(getClass().getResource("delftstack.fxml"));
DemoStage.setTitle("Demo");
Scene DemoScene = new Scene(DemoRoot, 200, 150);
DemoStage.setScene(DemoScene);
DemoStage.show();
}
}
The delftstack.fxml
file is:
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.control.ScrollPane?>
<?import javafx.scene.control.TableColumn?>
<?import javafx.scene.control.TableView?>
<?import javafx.scene.layout.AnchorPane?>
<AnchorPane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="400.0" prefWidth="600.0" xmlns="http://javafx.com/javafx/8.0.65" xmlns:fx="http://javafx.com/fxml/1">
<children>
<ScrollPane layoutX="178.0" layoutY="68.0" prefHeight="400.0" prefWidth="600.0" AnchorPane.bottomAnchor="0.0" AnchorPane.leftAnchor="0.0" AnchorPane.rightAnchor="0.0" AnchorPane.topAnchor="0.0">
<content>
<TableView prefHeight="350.0" prefWidth="590.0">
<columns>
<TableColumn prefWidth="130.0" text="First Name" />
<TableColumn prefWidth="130.0" text="Last Name" />
<TableColumn prefWidth="330.0" text="ID" />
</columns>
</TableView>
</content>
</ScrollPane>
</children>
</AnchorPane>
The code above will throw the InvocationTargetException
because it couldn’t find the delftstack.fxml
file. See output:
Start()
Exception in Application start method
Exception in thread "main" java.lang.reflect.InvocationTargetException
at java.base/jdk.internal.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at java.base/jdk.internal.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:77)
at java.base/jdk.internal.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.base/java.lang.reflect.Method.invoke(Method.java:568)
at java.base/sun.launcher.LauncherHelper$FXHelper.main(LauncherHelper.java:1071)
Caused by: java.lang.RuntimeException: Exception in Application start method
at javafx.graphics@18.0.1/com.sun.javafx.application.LauncherImpl.launchApplication1(LauncherImpl.java:901)
at javafx.graphics@18.0.1/com.sun.javafx.application.LauncherImpl.lambda$launchApplication$2(LauncherImpl.java:196)
at java.base/java.lang.Thread.run(Thread.java:833)
Caused by: java.lang.NullPointerException: Location is required.
at javafx.fxml@18.0.1/javafx.fxml.FXMLLoader.loadImpl(FXMLLoader.java:3324)
at javafx.fxml@18.0.1/javafx.fxml.FXMLLoader.loadImpl(FXMLLoader.java:3287)
at javafx.fxml@18.0.1/javafx.fxml.FXMLLoader.loadImpl(FXMLLoader.java:3255)
at javafx.fxml@18.0.1/javafx.fxml.FXMLLoader.loadImpl(FXMLLoader.java:3227)
at javafx.fxml@18.0.1/javafx.fxml.FXMLLoader.loadImpl(FXMLLoader.java:3203)
at javafx.fxml@18.0.1/javafx.fxml.FXMLLoader.load(FXMLLoader.java:3196)
at delftstack.Example.start(Example.java:15)
at javafx.graphics@18.0.1/com.sun.javafx.application.LauncherImpl.lambda$launchApplication1$9(LauncherImpl.java:847)
at javafx.graphics@18.0.1/com.sun.javafx.application.PlatformImpl.lambda$runAndWait$12(PlatformImpl.java:484)
at javafx.graphics@18.0.1/com.sun.javafx.application.PlatformImpl.lambda$runLater$10(PlatformImpl.java:457)
at java.base/java.security.AccessController.doPrivileged(AccessController.java:399)
at javafx.graphics@18.0.1/com.sun.javafx.application.PlatformImpl.lambda$runLater$11(PlatformImpl.java:456)
at javafx.graphics@18.0.1/com.sun.glass.ui.InvokeLaterDispatcher$Future.run(InvokeLaterDispatcher.java:96)
at javafx.graphics@18.0.1/com.sun.glass.ui.win.WinApplication._runLoop(Native Method)
at javafx.graphics@18.0.1/com.sun.glass.ui.win.WinApplication.lambda$runLoop$3(WinApplication.java:184)
... 1 more
Now, if we try to solve it by giving the absolute path for the file delftstack.fxml
, it may solve the problem, but for some systems, it can still cause the same problem. The best solution for this error is given below:
- Copy the
delftstack.fxml
file in the same directory of the class. For example, if the class file is in thedelftstack
package, the FXML file should be copied to theproject/src/delftstack
folder. - Refresh the project in the IDE. For example, in Eclipse, right-click on the project name and select Refresh.
- Run the same program, and the error will be solved.
The output after following the above solution is:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Java JavaFX
- How to Play a Video Using JavaFX
- How to Fix Error: JavaFX FXML Load Exception
- How to Create JavaFX Message Box
- JavaFX Media Player
- How to Create Square in JavaFX
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack