How to Create Square in JavaFX
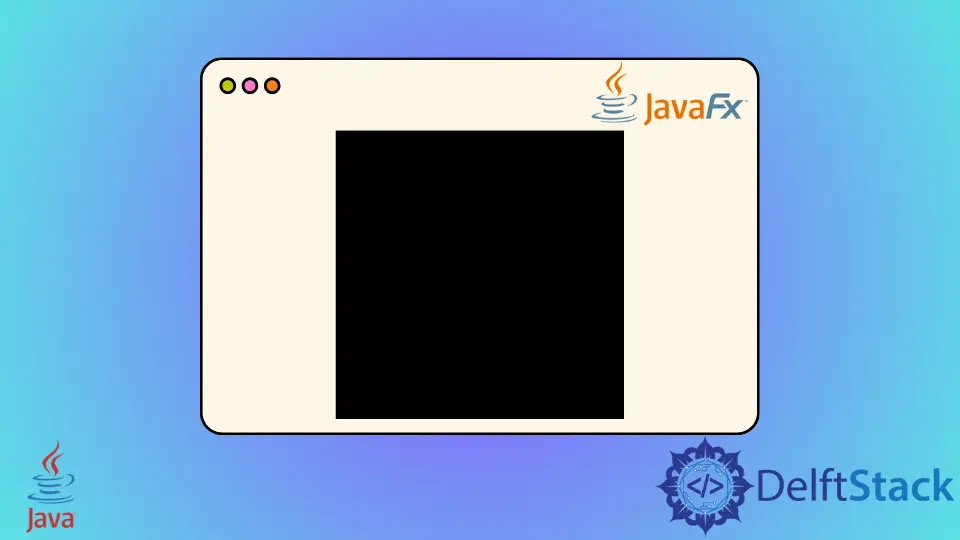
A square has all the sides equal to each other; we can use the Rectangle
functionality in JavaFX to create a square. This tutorial demonstrates how to create a square in JavaFX.
Create a Square in JavaFX
The Rectangle
functionality creates four side shapes in JavaFX. The Rectangle class belongs to JavaFX.scene.shape
.
We can instantiate the Rectangle
class and create Rectangle Node in JavaFX.
The syntax for rectangle and its properties:
Rectangle rectangle = new Rectangle(x, y, width, height);
setX(value of x);
setY(value of y);
setWidth(width);
setHeight(height);
The Rectangle
class has four basic properties:
x
: This is the x coordinate of the start point of the rectangle.y
: This is the y coordinate of the start point of the rectangle.width
: Width of the rectangle.height
: Height of the rectangle.
To draw a square in JavaFX, do the following steps.
-
Create a class by extending the Application class.
-
Start Implementing the
start()
method. -
Create a square by instantiating the class
Rectangle
. -
Set the properties of the rectangle. Make sure the
X
is equal toY
, and thewidth
is equal to theheight
of the square. -
Create a group object, instantiate the group class, and pass the
rectangle
to it. -
Create a scene object, instantiate the
scene
class, and pass the group object to the scene. -
Set the title using the
setTitle()
method. -
Add a scene to the stage using the
setScene()
method. -
Display the stage using the
show
method and launch the application.
Let’s try to implement an example based on the above steps.
Example Code:
package delftstack;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.shape.Rectangle;
import javafx.stage.Stage;
public class JavaFX_Square extends Application {
@Override
public void start(Stage Demo_Stage) {
Rectangle Square = new Rectangle();
Square.setX(150.0f);
Square.setY(150.0f);
Square.setWidth(300.0f);
Square.setHeight(300.0f);
Group Demo_Root = new Group(Square);
Scene Demo_Scene = new Scene(Demo_Root, 600, 600);
Demo_Stage.setTitle("Drawing Sqaure");
Demo_Stage.setScene(Demo_Scene);
Demo_Stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
In this JavaFX code, we first define a package called delftstack
and import necessary JavaFX classes. The main class, JavaFX_Square
, extends the Application
class and overrides the start()
method, serving as the entry point for the JavaFX application.
Inside this method, a Rectangle
object named Square
is instantiated to represent the square, and its properties, such as position and size, are set. A Group
object, Demo_Root
, is created to hold the square, allowing multiple objects to be treated as a single unit.
Subsequently, a Scene
object, Demo_Scene
, is formed with a specified width
and height
associated with the root group. The title of the stage, representing the top-level container, is set to Drawing Square
.
The created scene is then assigned to the stage, and the stage is displayed, presenting the square on the screen. The program’s entry point is the main()
method, where the JavaFX application is launched by invoking the launch()
method with command-line arguments.
Output:
In the output, we see a circle with a height
of 300px
and a width
of 300px
.
Create a Square in JavaFX With Round Corners
Let’s try to create a square with round corners. For round corners, we use the properties setArcHeight()
and setArcWidth()
.
Example Code:
package delftstack;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.stage.Stage;
public class JavaFX_Square extends Application {
@Override
public void start(Stage Demo_Stage) {
Rectangle Square = new Rectangle();
Square.setX(150.0f);
Square.setY(150.0f);
Square.setWidth(300.0f);
Square.setHeight(300.0f);
Square.setArcHeight(35);
Square.setArcWidth(35);
Square.setFill(Color.LIGHTBLUE);
Group Demo_Root = new Group(Square);
Scene Demo_Scene = new Scene(Demo_Root, 600, 600);
Demo_Stage.setTitle("Drawing Sqaure");
Demo_Stage.setScene(Demo_Scene);
Demo_Stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
In this JavaFX code, we start by defining a package, importing necessary JavaFX classes, and creating a class named JavaFX_Square
that extends the Application
class. Within the overridden start()
method, we instantiate a Rectangle
object, representing the square, and set its properties such as position, size, rounded corners, and fill color.
We then create a Group
named Demo_Root
to encapsulate the square, facilitating the collective treatment of multiple objects. A Scene
, Demo_Scene
, is formed with specified dimensions and associated with the root group.
The stage’s title is set to "Drawing Square"
, and the scene is displayed, showcasing the styled square. The entry point of the program is the main()
method, where we launch the JavaFX application.
Output:
In the output, it shows a round corner square with a height
and width
of 300px
.
Conclusion
In conclusion, this JavaFX tutorial demonstrates the creation of a square using the Rectangle
functionality. The code involves defining a package, importing JavaFX classes, and extending the Application
class to implement the square drawing.
The process includes setting properties such as position, size, and, optionally, rounded corners. The square is encapsulated in a Group
and associated with a Scene
for display.
The title of the stage is set, and the application is launched. Two examples are provided: one for a standard square and another for a square with rounded corners.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook