在 JavaFX 中建立正方形
Sheeraz Gul
2024年2月15日
Java
Java JavaFX
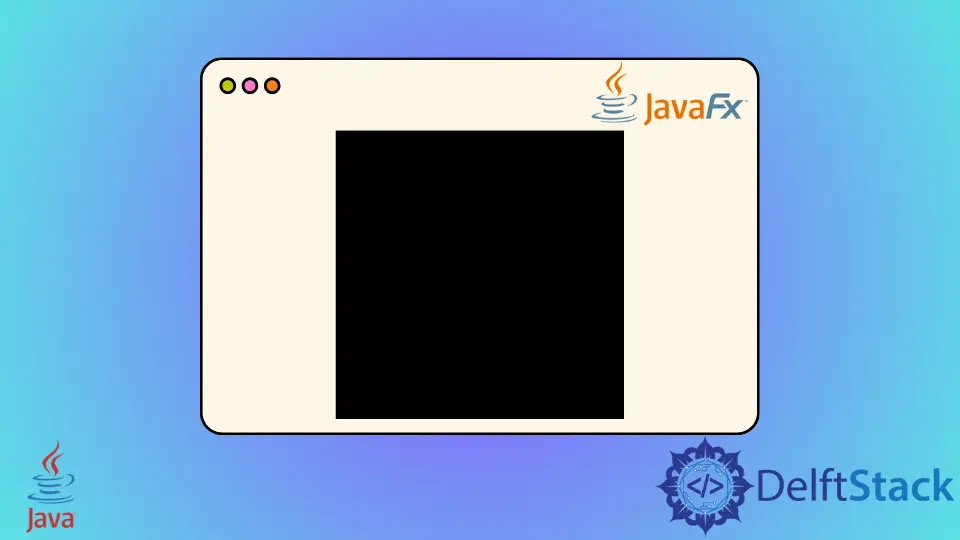
正方形的所有邊彼此相等;我們可以使用 JavaFX 中的 Rectangle
功能來建立一個正方形。本教程演示瞭如何在 JavaFX 中建立正方形。
在 JavaFX 中建立正方形
矩形
功能在 JavaFX 中建立四個側面形狀。Rectangle 類屬於 JavaFX.scene.shape
。
我們可以例項化 Rectangle
類並在 JavaFX 中建立 Rectangle 節點。Rectangle
類有四個基本屬性:
x
- 這是矩形起點的 x 座標。y
- 這是矩形起點的 y 座標。width
- 矩形的寬度。height
- 矩形的高度。
矩形及其屬性的語法:
Rectangle rectangle = new Rectangle(x, y, width, height);
setX(value of x);
setY(value of y);
setWidth(width);
setHeight(height);
要在 JavaFX 中繪製正方形,請執行以下步驟。
- 通過擴充套件 Application 類建立一個類。
- 開始實現
start()
方法。 - 通過例項化類
Rectangle
建立一個正方形。 - 設定矩形的屬性。確保 X 等於 Y,寬度等於正方形的高度。
- 建立一個組物件,例項化組類並將
矩形
傳遞給它。 - 建立一個場景物件,例項化
scene
類,並將組物件傳遞給場景。 - 使用
setTitle()
方法設定標題。 - 使用
setScene()
方法將場景新增到舞臺。 - 使用
show
方法顯示舞臺並啟動應用程式。
讓我們嘗試根據上述步驟實現一個示例。
示例程式碼:
package delftstack;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.shape.Rectangle;
import javafx.stage.Stage;
public class JavaFX_Square extends Application {
@Override
public void start(Stage Demo_Stage) {
// Drawing a Square
Rectangle Square = new Rectangle();
// Setting the properties of the Square
Square.setX(150.0f);
Square.setY(150.0f);
Square.setWidth(300.0f);
Square.setHeight(300.0f);
// Create a Group object
Group Demo_Root = new Group(Square);
// Create a scene object
Scene Demo_Scene = new Scene(Demo_Root, 600, 600);
// Set title to the Stage
Demo_Stage.setTitle("Drawing Sqaure");
// Add scene to the stage
Demo_Stage.setScene(Demo_Scene);
// Display the stage
Demo_Stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
上面的程式碼將建立一個高度為 300 畫素、寬度為 300 畫素的圓圈。見輸出:
讓我們嘗試建立一個帶圓角的正方形。對於圓角,我們使用屬性 setArcHeight()
和 setArcWidth()
。參見示例:
package delftstack;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.stage.Stage;
public class JavaFX_Square extends Application {
@Override
public void start(Stage Demo_Stage) {
// Drawing a Square
Rectangle Square = new Rectangle();
// Setting the properties of the Square
Square.setX(150.0f);
Square.setY(150.0f);
Square.setWidth(300.0f);
Square.setHeight(300.0f);
Square.setArcHeight(35);
Square.setArcWidth(35);
Square.setFill(Color.LIGHTBLUE);
// Create a Group object
Group Demo_Root = new Group(Square);
// Create a scene object
Scene Demo_Scene = new Scene(Demo_Root, 600, 600);
// Set title to the Stage
Demo_Stage.setTitle("Drawing Sqaure");
// Add scene to the stage
Demo_Stage.setScene(Demo_Scene);
// Display the stage
Demo_Stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
上面的程式碼將建立一個高度和寬度為 300 畫素的圓角正方形。見輸出:
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook