How to Convert String to InputStream in Java
-
Use
ByteArrayInputStream()
to Convert a String toInputStream
in Java -
Use
StringReader
andReaderInputStream
to Convert a String to anInputStream
in Java -
Use
org.apache.commons.io.IOUtils
to Convert a String to anInputStream
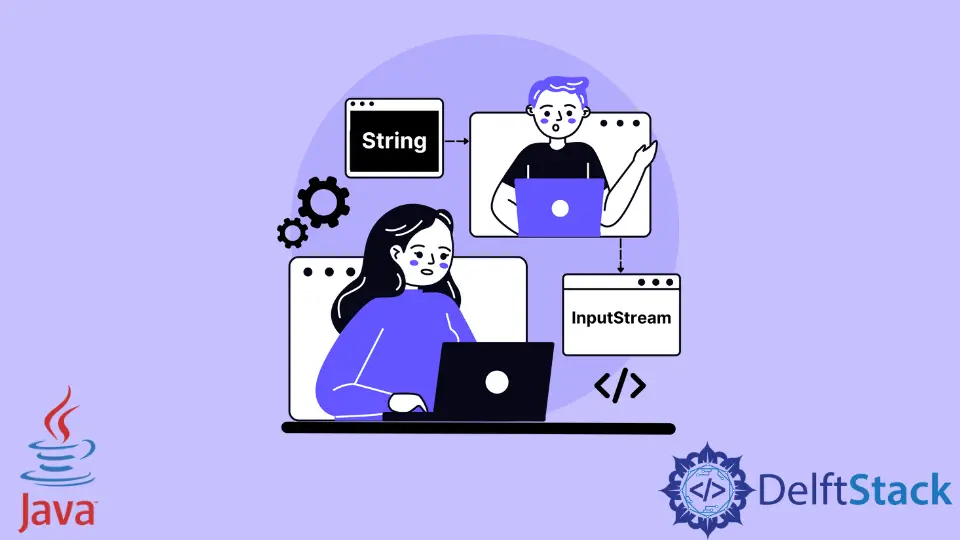
We will talk about how to convert a string to an InputStream
in Java using several methods. A string is a set of characters, while an InputStream
is a set of bytes. Let’s see how we can convert string to InputStream
in Java.
Use ByteArrayInputStream()
to Convert a String to InputStream
in Java
Java’s Input/Output package has the class ByteArrayInputStream
that reads the byte arrays as InputStream
. First, we use getBytes()
to get the bytes from exampleString
with the charset UTF_8, and then pass it to ByteArrayInputStream
.
To check if we succeed in our goal, we can read the inputStream
using read()
, and convert every byte
to a char
. This will return our original string.
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.nio.charset.StandardCharsets;
public class Main {
public static void main(String[] args) throws IOException {
String exampleString = "This is a sample string";
InputStream inputStream =
new ByteArrayInputStream(exampleString.getBytes(StandardCharsets.UTF_8));
// To check if we can read the string back from the inputstream
int i;
while ((i = inputStream.read()) != -1) {
char getSingleChar = (char) i;
System.out.print(getSingleChar);
}
}
}
Output:
This is a sample string
Use StringReader
and ReaderInputStream
to Convert a String to an InputStream
in Java
The second technique to convert the string to InputStream
uses two methods, StringReader
and ReaderInputStream
. The former is used to read the string and wrap it into a reader
while the latter takes two arguments, a reader
and the charsets
. At last, we get the InputStream
.
import java.io.IOException;
import java.io.InputStream;
import java.io.StringReader;
import java.nio.charset.StandardCharsets;
import org.apache.commons.io.input.ReaderInputStream;
public class Main {
public static void main(String[] args) throws IOException {
String exampleString = "This is a sample string";
StringReader stringReader = new StringReader(exampleString);
InputStream inputStream = new ReaderInputStream(stringReader, StandardCharsets.UTF_8);
// To check if we can read the string back from the inputstream
int i;
while ((i = inputStream.read()) != -1) {
char getSingleChar = (char) i;
System.out.print(getSingleChar);
}
}
}
Output:
This is a sample string
Use org.apache.commons.io.IOUtils
to Convert a String to an InputStream
We can also use the Apache Commons library to make our task easier. The IOUtls
class of this Apache Commons library has a toInputStream()
method that takes a string and the charset to use. This method is easiest of all as we only have to call a single method to convert the Java string to InputStream
.
import java.io.IOException;
import java.io.InputStream;
import java.nio.charset.StandardCharsets;
import org.apache.commons.io.IOUtils;
public class Main {
public static void main(String[] args) throws IOException {
String exampleString = "This is a sample string";
InputStream is = IOUtils.toInputStream(exampleString, StandardCharsets.UTF_8);
// To check if we can read the string back from the inputstream
int i;
while ((i = is.read()) != -1) {
char getSingleChar = (char) i;
System.out.print(getSingleChar);
}
}
}
Output:
This is a sample string
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java String
- How to Perform String to String Array Conversion in Java
- How to Remove Substring From String in Java
- How to Convert Byte Array in Hex String in Java
- How to Convert Java String Into Byte
- How to Generate Random String in Java
- The Swap Method in Java