How to Convert InputStream to the File Object in Java
-
Use Plain Java to Convert
InputStream
to theFile
Object -
Use the
Files.copy()
Method to ConvertInputStream
to theFile
Object in Java -
Use the
InputStream.transferTo()
Method to ConvertInputStream
to theFile
Object in Java -
Use Apache Commons IO API to Convert
InputStream
to theFile
Object in Java
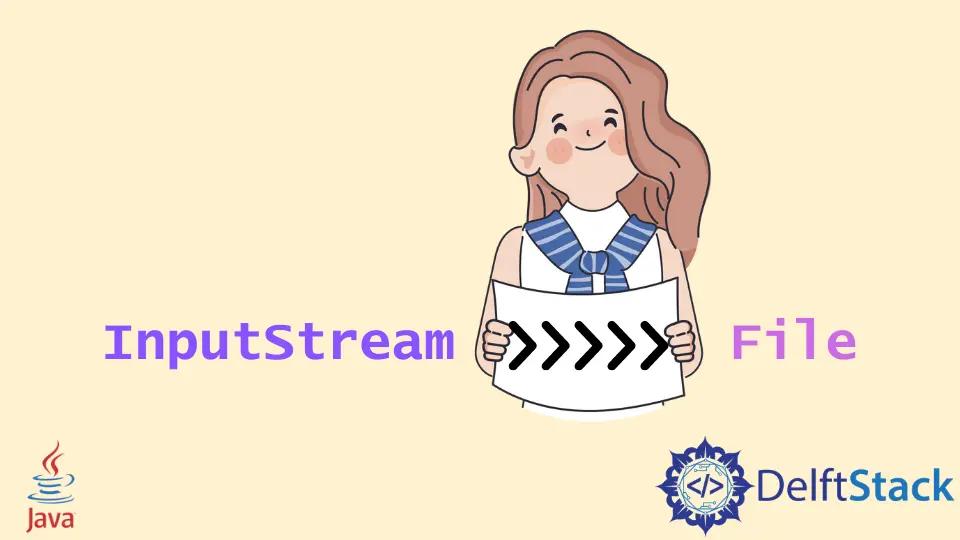
We frequently have to make files and add content to them. For instance, we need to save the bytes received over the network or String elements to a file.
In each case, the data is present as InputStream
and must be written to a file. Today’s tutorial discusses how to convert InputStream
to the File
object in Java.
For that, we will write different example codes using different ways to convert InputStream
to the File
object, depending on our Java version. Some of the approaches are given below:
Use Plain Java to Convert InputStream
to the File
Object
Example Code:
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
public class plainJava {
public static void main(String[] args) {
try {
InputStream inputStream = new FileInputStream(new File("./files/test.txt"));
byte[] buffer = new byte[inputStream.available()];
inputStream.read(buffer);
File destinationFile = new File("./files/test-plainJava-output.txt");
OutputStream outputStream = new FileOutputStream(destinationFile);
outputStream.write(buffer);
} catch (FileNotFoundException exception) {
exception.printStackTrace();
} catch (IOException exception) {
exception.printStackTrace();
}
}
}
We instantiate the FileInputStream
in the above Java program by specifying the file path. It will generate a FileNotFoundException
exception if the file does not exist or we have misspelled it.
We are using FileInputStream
to retrieve the input bytes from the given file. Then, we create an array named buffer
of type byte
where the inputStream.available()
method tells the number of bytes that we can read until the read()
call blocks the execution flow of the program.
After that, we instantiate the File
class to create the test-plainJava-output.txt
file and save its reference in the destinationFile
variable. Next, we create an object of FileOutputStream
, pass the reference of the destination file we created in the previous step, and write the byte
code.
Now, open the test.txt
and test-plainJava-output.txt
files and see both have the same content. Remember, we may get the IOException
if we have any issues while reading or writing information.
Use the Files.copy()
Method to Convert InputStream
to the File
Object in Java
Example Code:
import java.io.IOException;
import java.io.InputStream;
import java.net.URI;
import java.nio.file.Files;
import java.nio.file.Paths;
public class filesCopy {
private static final String OUTPUT_FILE = "./files/test_filesCopy-output.txt";
public static void main(String[] args) {
URI u = URI.create("https://www.google.com/");
try (InputStream inputStream = u.toURL().openStream()) {
// Java 1.7 onwards
Files.copy(inputStream, Paths.get(OUTPUT_FILE));
} catch (IOException e) {
e.printStackTrace();
}
}
}
We can use this approach in Java 7 or higher. In the above code fence, we are using Files.copy()
of Java NIO API which is used to copy the InputStream
object to the File
Object as demonstrated above.
In the filesCopy
program, we first create a String-type object to contain the path for the output file.
In the main()
method, we create a URI
object using the create()
method and save its reference in the u
variable, which will be used in the next step. Remember, we use create()
when we are sure that the specified string value will be parsed as a URI
object; otherwise, it would be considered a programmer’s fault.
Next, we use the URI
object u
to call the toURL()
method to construct a URL from this URI
and use the openStream()
method to get java.io.InputSteam
object. After that, we use the Files.copy()
method, which takes two parameters, the inputStream
object and a Path
of the file, where we want to copy the data.
Now, we will see the parsed data in our output file, test_filesCopy-output.txt
in our case. We use the try-catch
block to handle the exception if any occurs; otherwise, the program will terminate execution.
Use the InputStream.transferTo()
Method to Convert InputStream
to the File
Object in Java
Example Code:
import java.io.*;
import java.net.URI;
public class inputStreamTransferTo {
public static void main(String[] args) {
URI u = URI.create("https://www.google.com/");
try (InputStream inputStream = u.toURL().openStream()) {
File outputFile = new File("./files/test_transferTo-output.txt");
OutputStream outputStream = new FileOutputStream(outputFile, false);
inputStream.transferTo(outputStream); // Java 9
} catch (IOException e) {
e.printStackTrace();
}
}
}
We can use this approach if we have Java 9 or later. This code example is similar to the previous one where we used the Files.copy()
method except for a few differences in the try
block.
Here, we create an object of the File
class by specifying the output file, test_transferTo-output.txt
in our case. Then, we use this File
object to instantiate the FileOutputStream
class, which we will use to write data to our output file (test_transferTo_output.txt
).
Finally, we use the inputStream.transferTo()
method, which takes an output stream as a parameter and directly copies inputStream
to the specified outputSteam
. Now, you will have the parsed data in the output file, test_transferTo_output.txt
.
We also enclosed this code within the try-catch
block to avoid the program’s termination.
Use Apache Commons IO API to Convert InputStream
to the File
Object in Java
Example Code:
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.net.URI;
import org.apache.commons.io.FileUtils;
public class apacheCommons {
public static void main(String[] args) throws IOException {
URI u = URI.create("https://www.google.com/");
try (InputStream inputStream = u.toURL().openStream()) {
File outputFile = new File("./files/test_apache_output.txt");
// commons-io
FileUtils.copyInputStreamToFile(inputStream, outputFile);
}
}
}
This example code is similar to the previous ones. We create a URI
object to call toURL
, which will build a URL via this URI
object, get the java.io.InputStream
object using the openStream()
method, and instantiate the File
class pointing to a path for our output file which is test_apache_output.txt
here.
But, we are using Apache Commons IO API to convert the input stream to the file object in Java. This API is very handy and provides various functionalities to perform different File input/output operations.
To use this library, we first have to add the commons-io
dependency in the pom.xml
file as follows:
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.7</version>
</dependency>
Then, we can use the copyInputStreamToFile()
method to read bytes from an input stream and copy them into the specified file. Now, you can see the parsed data in the test_apache_output.txt
file, which is an output file.