Java에서 문자열을 InputStream으로 변환
-
Java에서
ByteArrayInputStream()
을 사용하여 문자열을InputStream
으로 변환 -
StringReader
및ReaderInputStream
을 사용하여 Java에서 문자열을InputStream
으로 변환 -
org.apache.commons.io.IOUtils
를 사용하여 문자열을InputStream
으로 변환
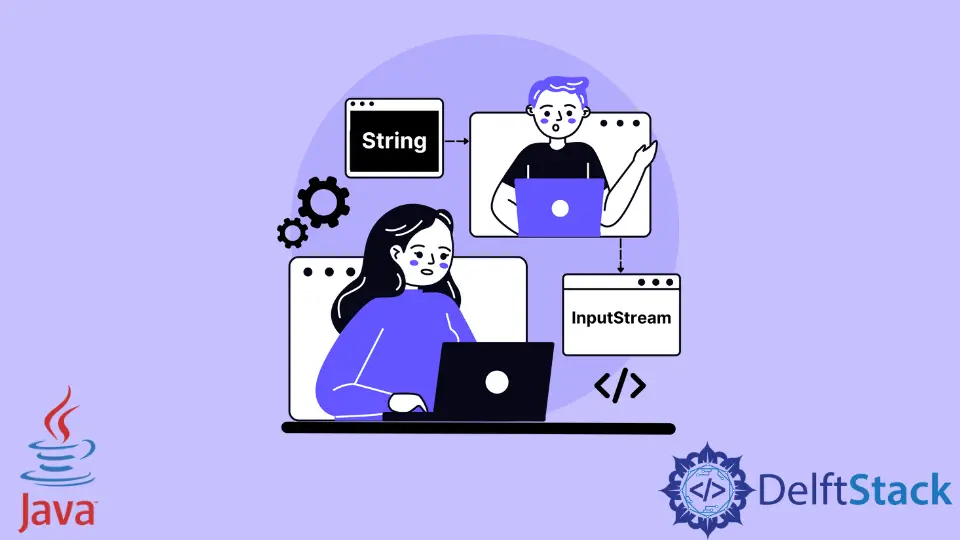
몇 가지 방법을 사용하여 Java에서 문자열을 InputStream
으로 변환하는 방법에 대해 설명합니다. 문자열은 문자 집합이고 InputStream
은 바이트 집합입니다. Java에서 문자열을 InputStream
으로 변환하는 방법을 살펴 보겠습니다.
Java에서ByteArrayInputStream()
을 사용하여 문자열을InputStream
으로 변환
Java의 입력 / 출력 패키지에는 바이트 배열을 InputStream
으로 읽는 ByteArrayInputStream
클래스가 있습니다. 먼저getBytes()
를 사용하여 charset UTF_8로exampleString
에서 바이트를 가져온 다음ByteArrayInputStream
에 전달합니다.
목표에 성공했는지 확인하기 위해read()
를 사용하여inputStream
을 읽고 모든byte
를char
로 변환 할 수 있습니다. 그러면 원래 문자열이 반환됩니다.
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.nio.charset.StandardCharsets;
public class Main {
public static void main(String[] args) throws IOException {
String exampleString = "This is a sample string";
InputStream inputStream =
new ByteArrayInputStream(exampleString.getBytes(StandardCharsets.UTF_8));
// To check if we can read the string back from the inputstream
int i;
while ((i = inputStream.read()) != -1) {
char getSingleChar = (char) i;
System.out.print(getSingleChar);
}
}
}
출력:
This is a sample string
StringReader
및ReaderInputStream
을 사용하여 Java에서 문자열을InputStream
으로 변환
문자열을 InputStream
으로 변환하는 두 번째 기법은 StringReader
와 ReaderInputStream
의 두 가지 방법을 사용합니다. 전자는 문자열을 읽고 reader
로 감싸는 데 사용되며 후자는 reader
와 charsets
라는 두 개의 인수를 사용합니다. 마침내 우리는InputStream
을 얻습니다.
import java.io.IOException;
import java.io.InputStream;
import java.io.StringReader;
import java.nio.charset.StandardCharsets;
import org.apache.commons.io.input.ReaderInputStream;
public class Main {
public static void main(String[] args) throws IOException {
String exampleString = "This is a sample string";
StringReader stringReader = new StringReader(exampleString);
InputStream inputStream = new ReaderInputStream(stringReader, StandardCharsets.UTF_8);
// To check if we can read the string back from the inputstream
int i;
while ((i = inputStream.read()) != -1) {
char getSingleChar = (char) i;
System.out.print(getSingleChar);
}
}
}
출력:
This is a sample string
org.apache.commons.io.IOUtils
를 사용하여 문자열을InputStream
으로 변환
Apache Commons 라이브러리를 사용하여 작업을 더 쉽게 할 수도 있습니다. 이 Apache Commons 라이브러리의IOUtls
클래스에는 사용할 문자열과 문자 집합을받는toInputStream()
메서드가 있습니다. 이 메서드는 Java 문자열을InputStream
으로 변환하기 위해 단일 메서드 만 호출하면되므로 가장 쉽습니다.
import java.io.IOException;
import java.io.InputStream;
import java.nio.charset.StandardCharsets;
import org.apache.commons.io.IOUtils;
public class Main {
public static void main(String[] args) throws IOException {
String exampleString = "This is a sample string";
InputStream is = IOUtils.toInputStream(exampleString, StandardCharsets.UTF_8);
// To check if we can read the string back from the inputstream
int i;
while ((i = is.read()) != -1) {
char getSingleChar = (char) i;
System.out.print(getSingleChar);
}
}
}
출력:
This is a sample string
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn관련 문장 - Java String
- Java 문자열을 바이트로 변환하는 방법
- Java에서 16 진 문자열의 바이트 배열을 변환하는 방법
- Java에서 문자열 대 문자열 배열 변환을 수행하는 방법
- Java에서 문자열에서 부분 문자열을 제거하는 방법
- Java에서 임의 문자열 생성
- Java의 스왑 방법